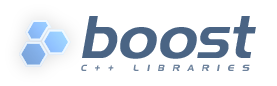 |
Boost.Hana
1.7.0
Your standard library for metaprogramming
|
|
Includes all the library components except the adapters for external libraries.
More...
Includes all the library components except the adapters for external libraries.
- Copyright
- Louis Dionne 2013-2017 Distributed under the Boost Software License, Version 1.0. (See accompanying file LICENSE.md or copy at http://boost.org/LICENSE_1_0.txt)
Forward declares boost::hana::remove_range and boost::hana::remove_range_c.
constexpr auto scan_right
Fold a Sequence to the right and return a list containing the successive reduction states.
Definition: scan_right.hpp:86
auto BOOST_HANA_ADAPT_STRUCT(...)
Defines a model of Struct with the given members.
Returns whether the Predicate is satisfied by any of the T....
Definition: any_of.hpp:42
Defines boost::hana::arg.
Defines boost::hana::detail::variadic::foldr1.
constexpr auto eval
Evaluate a lazy value and return it.
Definition: eval.hpp:42
constexpr auto reverse_partial
Partially apply a function to some arguments.
Definition: reverse_partial.hpp:42
Defines boost::hana::Foldable.
Forward declares boost::hana::remove.
Forward declares boost::hana::at_key.
Forward declares boost::hana::drop_front.
Defines several constexpr algorithms.
Defines the BOOST_HANA_DEFINE_STRUCT, BOOST_HANA_ADAPT_STRUCT, and BOOST_HANA_ADAPT_ADT macros.
Defines boost::hana::filter.
Forward declares boost::hana::back.
Defines boost::hana::less.
Defines boost::hana::detail::operators::adl.
Defines boost::hana::equal.
Defines boost::hana::always.
Forward declares boost::hana::span.
Returns whether any of the Ts are duplicate w.r.t. hana::equal.
Definition: has_duplicates.hpp:57
constexpr auto one
Identity of the Ring multiplication.
Definition: one.hpp:30
Provides a .by static constexpr function object.
Definition: nested_by_fwd.hpp:49
Defines boost::hana::chain.
Forward declares boost::hana::replace.
constexpr auto capture
Create a function capturing the given variables.
Definition: capture.hpp:45
Defines boost::hana::Applicative.
constexpr auto is_valid
Checks whether a SFINAE-friendly expression is valid.
Definition: type.hpp:369
Defines boost::hana::if_.
Tag representing hana::basic_tuple.
Definition: basic_tuple.hpp:39
Defines boost::hana::replace.
constexpr auto is_empty
Returns whether the iterable is empty.
Definition: is_empty.hpp:33
constexpr auto always
Return a constant function returning x regardless of the argument(s) it is invoked with.
Definition: always.hpp:37
constexpr auto less_equal
Returns a Logical representing whether x is less than or equal to y.
Definition: less_equal.hpp:38
Defines boost::hana::Sequence.
Forward declares boost::hana::remove_at and boost::hana::remove_at_c.
Forward declares boost::hana::optional.
Defines boost::hana::zip.
Defines boost::hana::find_if.
Defines boost::hana::common and boost::hana::common_t.
Defines boost::hana::unique.
Forward declares boost::hana::lexicographical_compare.
constexpr auto is_a
Returns whether the tag of an object matches a given tag.
Definition: is_a.hpp:40
Defines boost::hana::drop_front.
Defines boost::hana::flatten.
Defines operators for Comparables.
Forward declares boost::hana::concat.
Defines boost::hana::hash.
Defines boost::hana::any_of.
Forward declares boost::hana::map.
constexpr auto lift
Lift a value into an Applicative structure.
Definition: lift.hpp:44
Defines boost::hana::mod.
Forward declares boost::hana::intersection.
Documents the BOOST_HANA_ADAPT_ADT macro.
constexpr auto count_if
Return the number of elements in the structure for which the predicate is satisfied.
Definition: count_if.hpp:40
constexpr auto less
Returns a Logical representing whether x is less than y.
Definition: less.hpp:37
Forward declares boost::hana::fold_right.
constexpr map(map &&other)=default
Move-construct a map from another map. This constructor only exists when all the elements of the map ...
constexpr auto difference
Returns the difference of two maps.
Definition: map.hpp:349
Forward declares boost::hana::Functor.
auto type_name()
Returns a hana::string representing the name of the given type, at compile-time.
Definition: type_name.hpp:58
Forward declares boost::hana::EuclideanRing.
constexpr auto tap
Tap inside a monadic chain.
Definition: tap.hpp:50
constexpr set()=default
Default-construct a set. This constructor only exists when all the elements of the set are default-co...
Adapts boost::mpl::list for use with Hana.
constexpr auto slice_c
Shorthand to slice a contiguous range of elements.
Definition: slice.hpp:93
Defines boost::hana::zero.
constexpr auto at_c
Equivalent to at; provided for convenience.
Definition: at.hpp:80
Forward declares boost::hana::is_empty.
constexpr auto make< basic_tuple_tag >
Function object for creating a basic_tuple.
Definition: basic_tuple.hpp:55
Forward declares boost::hana::replace_if.
Forward declares boost::hana::not_equal.
Forward declares boost::hana::count.
constexpr auto make< lazy_tag >
Lifts a normal value to a lazy one.
Definition: lazy.hpp:110
Equivalent to std::decay, except faster.
Definition: decay.hpp:33
Defines configuration macros used throughout the library.
Defines boost::hana::basic_tuple.
Defines boost::hana::drop_back.
Forward declares boost::hana::tag_of and boost::hana::tag_of_t.
Defines the BOOST_HANA_ADAPT_STRUCT macro.
Defines boost::hana::tuple.
Defines boost::hana::repeat.
Forward declares boost::hana::mod.
constexpr auto negate
Return the inverse of an element of a group.
Definition: negate.hpp:26
Defines boost::hana::unfold_right.
when< true > when_valid
Variant of when allowing specializations to be enabled only if an expression is well-formed.
Definition: when.hpp:65
constexpr auto zip_shortest_with
Zip one sequence or more with a given function.
Definition: zip_shortest_with.hpp:46
constexpr pair & operator=(pair< T, U > const &other)
Assign a pair to another pair. Only exists when both elements of the destination pair are assignable ...
Forward declares boost::hana::div.
Forward declares boost::hana::drop_back.
constexpr map & operator=(map &&other)
Move-assign a map to another map with the exact same type. Only exists when all the elements of the m...
Adapter for IntegralConstants from the Boost.MPL.
Definition: integral_c.hpp:39
Defines boost::hana::detail::wrong.
constexpr auto intersperse
Insert a value between each pair of elements in a finite sequence.
Definition: intersperse.hpp:41
Defines the Logical and Comparable models of boost::hana::integral_constant.
Forward declares boost::hana::pair.
constexpr auto minus
Subtract two elements of a group.
Definition: minus.hpp:51
Forward declares boost::hana::less_equal.
Forward declares boost::hana::count_if.
Forward declares boost::hana::sort.
Metafunction returning whether two data types share a common data type.
Definition: common.hpp:48
Forward declares boost::hana::filter.
constexpr auto make
Create an object of the given tag with the given arguments.
Definition: make.hpp:50
Defines boost::hana::is_a and boost::hana::is_an.
Defines boost::hana::flip.
constexpr auto second
Returns the second element of a pair.
Definition: second.hpp:32
Defines boost::hana::set.
Forward declares boost::hana::is_disjoint.
Defines boost::hana::tap.
constexpr auto ordering
Returns a function performing less after applying a transformation to both arguments.
Definition: ordering.hpp:50
Forward declares boost::hana::equal.
Defines boost::hana::take_while.
Forward declares boost::hana::drop_while.
constexpr auto id
The identity function – returns its argument unchanged.
Definition: id.hpp:23
Defines boost::hana::then.
Forward declares boost::hana::integral_constant.
Forward declares boost::hana::cycle.
Defines boost::hana::integral_constant.
constexpr auto sum
Compute the sum of the numbers of a structure.
Definition: sum.hpp:66
Defines boost::hana::Constant.
Returns whether there is a Hana-conversion from a data type to another.
Definition: to.hpp:151
constexpr auto find_if
Finds the value associated to the first key satisfying a predicate.
Definition: find_if.hpp:41
Forward declares boost::hana::replicate.
Defines the BOOST_HANA_ADAPT_ADT macro.
Tag representing a hana::optional.
Definition: optional.hpp:286
Defines boost::hana::detail::ebo.
Forward declares boost::hana::take_front and boost::hana::take_front_c.
Defines boost::hana::make.
Forward declares boost::hana::detail::nested_to.
Forward declares boost::hana::detail::nested_than.
Forward declares boost::hana::negate.
Defines boost::hana::zip_with.
Defines an equivalent to the proposed std::void_t.
Defines boost::hana::comparing.
Defines boost::hana::zip_shortest_with.
Returns whether a tag-dispatched method implementation is a default implementation.
Definition: default.hpp:22
Defines operators for Searchables.
constexpr auto find
Finds the value associated to the given key in a structure.
Definition: find.hpp:44
Compile-time half-open interval of hana::integral_constants.
Definition: range.hpp:67
constexpr auto concat
Combine two monadic structures together.
Definition: concat.hpp:47
Basic associative container requiring unique, Comparable and Hashable keys.
Definition: map.hpp:93
constexpr auto make_lazy
Alias to make<lazy_tag>; provided for convenience.
Definition: lazy.hpp:121
Defines boost::hana::one.
Forward declares boost::hana::flatten.
Defines boost::hana::adjust_if.
constexpr auto then
Sequentially compose two monadic actions, discarding any value produced by the first but not its effe...
Definition: then.hpp:36
Mark a tag-dispatched method implementation as a default implementation.
Definition: default.hpp:30
Defines boost::hana::detail::type_at.
Provides a .to static constexpr function object.
Definition: nested_to_fwd.hpp:41
Defines boost::hana::map.
constexpr pair(pair< T, U > const &other)
Copy-initialize a pair from another pair. Only exists when both elements of the source pair are impli...
constexpr auto drop_front_exactly
Drop the first n elements of an iterable, and return the rest.
Definition: drop_front_exactly.hpp:48
Defines boost::hana::lexicographical_compare.
constexpr auto make_pair
Alias to make<pair_tag>; provided for convenience.
Definition: pair.hpp:155
Forward declares boost::hana::append.
Forward declares boost::hana::then.
Forward declares boost::hana::hash.
constexpr auto front
Returns the first element of a non-empty iterable.
Definition: front.hpp:32
constexpr auto zero
Identity of plus.
Definition: zero.hpp:30
Defines logical operators.
Defines boost::hana::reverse.
Forward declares boost::hana::unfold_right.
Forward declares boost::hana::difference.
constexpr auto is_disjoint
Returns whether two Searchables are disjoint.
Definition: is_disjoint.hpp:34
Forward declares boost::hana::is_subset.
Includes all the adaptors for the Boost.MPL library.
constexpr auto is_an
Equivalent to is_a; provided for consistency with the rules of the English language.
Definition: is_a.hpp:54
typename has_common_embedding_impl< Concept, T, U >::type has_common_embedding
Returns whether T and U both have an embedding into a common type.
Definition: has_common_embedding.hpp:46
Defines boost::hana::find.
Returns the index of the first element which does not satisfy Pred, or sizeof...(Xs) if no such eleme...
Definition: first_unsatisfied_index.hpp:46
constexpr auto index_if
Finds the value associated to the first key satisfying a predicate.
Definition: index_if.hpp:43
Forward declares boost::hana::zip_shortest.
Adapter for Boost.Fusion tuples.
Definition: tuple.hpp:36
constexpr insert_t insert
Insert a value at a given index in a sequence.
Definition: insert.hpp:29
constexpr auto append
Append an element to a monadic structure.
Definition: append.hpp:52
Forward declares boost::hana::sum.
constexpr auto make_basic_tuple
Alias to make<basic_tuple_tag>; provided for convenience.
Definition: basic_tuple.hpp:67
constexpr optional()=default
Default-construct an optional. Only exists if the optional contains a value, and if that value is Def...
Defines boost::hana::detail::variadic::foldl1.
constexpr auto or_
Return whether any of the arguments is true-valued.
Definition: or.hpp:34
Forward declares boost::hana::unfold_left.
Forward declares boost::hana::fold.
Metafunction returning the tag associated to T.
Definition: tag_of.hpp:103
Defines macros for tracking the version of the library.
Defines boost::hana::cycle.
Includes boost/hana/fwd/integral_constant.hpp.
Forward declares boost::hana::union_.
Defines boost::hana::detail::variadic::at.
constexpr auto eval_if
Conditionally execute one of two branches based on a condition.
Definition: eval_if.hpp:139
constexpr auto take_while
Take elements from a sequence while the predicate is satisfied.
Definition: take_while.hpp:40
constexpr auto union_
Returns the union of two maps.
Definition: map.hpp:281
Adaptation of std::integer_sequence for Hana.
Definition: integer_sequence.hpp:60
Defines concepts from the Standard library.
Forward declares boost::hana::monadic_fold_right.
Adapts std::vector for use with Hana.
Forward declares boost::hana::length.
Defines boost::hana::min.
constexpr auto not_
Negates a Logical.
Definition: not.hpp:31
constexpr auto value
Return the compile-time value associated to a constant.
Definition: value.hpp:54
Defines boost::hana::detail::create.
Documents the BOOST_HANA_ADAPT_STRUCT macro.
Defines boost::hana::infix.
constexpr auto zip_with
Zip one sequence or more with a given function.
Definition: zip_with.hpp:46
Defines boost::hana::take_front and boost::hana::take_front_c.
Defines boost::hana::EuclideanRing.
Forward declares boost::hana::adjust.
Forward declares boost::hana::contains and boost::hana::in.
constexpr auto size
Equivalent to length; provided for consistency with the standard library.
Definition: size.hpp:30
Defines boost::hana::group.
constexpr auto if_
Conditionally return one of two values based on a condition.
Definition: if.hpp:41
constexpr auto arg
Return the nth passed argument.
Definition: arg.hpp:56
Forward declares boost::hana::string.
Defines boost::hana::detail::first_unsatisfied_index.
Provides a .than static constexpr function object.
Definition: nested_than_fwd.hpp:41
Defines boost::hana::power.
Defines boost::hana::detail::array.
Defines boost::hana::reverse_partial.
Forward declares boost::hana::Ring.
Container optimized for holding types.
Definition: types.hpp:48
constexpr tuple(tuple< Yn... > const &other)
Copy-initialize a tuple from another tuple. Only exists when all the elements of the constructed tupl...
constexpr pair(T &&t, U &&u)
Initialize both elements of the pair by perfect-forwarding the corresponding argument....
Defines boost::hana::index_if.
constexpr auto on
Invoke a function with the result of invoking another function on each argument.
Definition: on.hpp:54
constexpr integral_constant< T, v > integral_c
Creates an integral_constant holding the given compile-time value.
Definition: integral_constant.hpp:39
constexpr auto members
Returns a Sequence containing the members of a Struct.
Definition: members.hpp:30
A minimal std::array with better constexpr support.
Definition: array.hpp:36
Adapts boost::tuple for use with Hana.
Defines boost::hana::back.
Compile-time value of an integral type.
Definition: integral_constant.hpp:120
Forward declares boost::hana::greater.
Defines boost::hana::when and boost::hana::when_valid.
Defines boost::hana::count_if.
Adapter for Boost.MPL lists.
Definition: list.hpp:92
Defines boost::hana::detail::nested_by.
Defines boost::hana::insert_range.
Forward declares boost::hana::monadic_compose.
constexpr auto compose
Return the composition of two functions or more.
Definition: compose.hpp:52
constexpr auto drop_while
Drop elements from an iterable up to, but excluding, the first element for which the predicate is not...
Definition: drop_while.hpp:44
Forward declares boost::hana::find.
Defines boost::hana::Logical.
Defines macros for commonly used type traits.
Adapts std::pair for use with Hana.
constexpr pair & operator=(pair< T, U > &&other)
Move-assign a pair to another pair. Only exists when both elements of the destination pair are move-a...
Defines boost::hana::permutations.
constexpr unspecified _
Create simple functions representing C++ operators inline.
Definition: placeholder.hpp:70
constexpr auto take_back
Returns the last n elements of a sequence, or the whole sequence if the sequence has less than n elem...
Definition: take_back.hpp:42
Forward declares boost::hana::fold_left.
constexpr auto make< pair_tag >
Creates a hana::pair with the given elements.
Definition: pair.hpp:142
Defines boost::hana::partial.
constexpr auto min
Returns the smallest of its arguments according to the less ordering.
Definition: min.hpp:35
constexpr auto fold
Equivalent to fold_left; provided for convenience.
Definition: fold.hpp:35
Defines boost::hana::all_of.
Forward declares boost::hana::second.
Defines boost::hana::string.
Forward declares boost::hana::common and boost::hana::common_t.
Defines the BOOST_HANA_DEFINE_STRUCT macro.
Forward declares boost::hana::Monoid.
constexpr auto length
Return the number of elements in a foldable structure.
Definition: length.hpp:34
Forward declares boost::hana::less.
constexpr T & operator*()
Equivalent to value(), provided for convenience.
Optional value whose optional-ness is known at compile-time.
Definition: optional.hpp:136
constexpr auto make_type
Equivalent to make<type_tag>, provided for convenience.
Definition: type.hpp:262
Forward declares boost::hana::or_.
constexpr auto keys
Returns a Sequence of the keys of the map, in unspecified order.
Definition: map.hpp:186
Forward declares boost::hana::adjust_if.
Defines boost::hana::count.
constexpr T * operator->()
Returns a pointer to the contained value, or a nullptr if the optional is empty.
Forward declares boost::hana::intersperse.
Forward declares boost::hana::cartesian_product.
Defines boost::hana::Orderable.
constexpr auto plus
Associative binary operation on a Monoid.
Definition: plus.hpp:47
Forward declares boost::hana::Orderable.
Adapter for Boost.Fusion lists.
Definition: list.hpp:49
constexpr auto prepend
Prepend an element to a monadic structure.
Definition: prepend.hpp:57
Defines boost::hana::erase_key.
Forward declares boost::hana::make.
Forward declares boost::hana::find_if.
Forward declares boost::hana::if_.
constexpr set(set const &other)=default
Copy-construct a set from another set. This constructor only exists when all the elements of the set ...
constexpr auto fix
Return a function computing the fixed point of a function.
Definition: fix.hpp:53
The IntegralConstant concept represents compile-time integral values.
Definition: integral_constant.hpp:70
Defines boost::hana::Monad.
Defines boost::hana::slice and boost::hana::slice_c.
Forward declares boost::hana::monadic_fold_left.
Forward declares boost::hana::eval.
Enables ADL in the hana::detail::operators namespace.
Definition: adl.hpp:31
constexpr auto max
Returns the greatest of its arguments according to the less ordering.
Definition: max.hpp:28
constexpr auto take_front
Returns the first n elements of a sequence, or the whole sequence if the sequence has less than n ele...
Definition: take_front.hpp:42
Tag representing the hana::set container.
Definition: set.hpp:107
Forward declares boost::hana::mult.
Forward declares boost::hana::insert.
constexpr auto curry
Curry a function up to the given number of arguments.
Definition: curry.hpp:88
Defines boost::hana::unfold_left.
Defines boost::hana::and_.
constexpr auto alignof_
alignof keyword, lifted to Hana.
Definition: type.hpp:326
Forward declares boost::hana::reverse.
Forward declares boost::hana::zip_with.
Forward declares boost::hana::greater_equal.
constexpr auto zip
Zip one sequence or more.
Definition: zip.hpp:45
Adapter for Boost.Fusion deques.
Definition: deque.hpp:46
Defines boost::hana::negate.
Forward declares boost::hana::group.
Forward declares boost::hana::reverse_fold.
Defines boost::hana::tag_of and boost::hana::tag_of_t.
Defines boost::hana::eval.
Forward declares boost::hana::lift.
Forward declares boost::hana::not_.
constexpr optional(T &&t)
Construct an optional holding a value of type T from another object of type T. The value is move-cons...
Definition: optional.hpp:167
Defines boost::hana::capture.
Defines operators for Orderables.
constexpr auto value_of
Equivalent to value, but can be passed to higher-order algorithms.
Definition: value.hpp:86
Forward declares boost::hana::when and boost::hana::when_valid.
Defines boost::hana::contains and boost::hana::in.
constexpr friend auto operator|(lazy< T... >, F)
Equivalent to hana::chain.
Defines boost::hana::plus.
Defines boost::hana::range.
Defines boost::hana::accessors.
constexpr auto for_each
Perform an action on each element of a foldable, discarding the result each time.
Definition: for_each.hpp:39
Forward declares boost::hana::fuse.
Defines boost::hana::intersection.
Forward declares boost::hana::ordering.
Defines common methods for all Boost.Fusion sequences.
Defines boost::hana::greater.
Forward declares boost::hana::Searchable.
Defines boost::hana::type and related utilities.
optional(optional const &)=default
Copy-construct an optional. An empty optional may only be copy-constructed from another empty optiona...
Enable a partial specialization only if a boolean condition is true.
Definition: when.hpp:39
Forward declares boost::hana::take_back.
Equivalent to a type-dependent std::false_type.
Definition: wrong.hpp:30
Defines boost::hana::less_equal.
constexpr auto none_of
Returns whether none of the keys of the structure satisfy the predicate.
Definition: none_of.hpp:39
Defines the Functional module.
Adapter for Boost.Fusion vectors.
Definition: vector.hpp:48
Defines boost::hana::detail::fast_and.
Defines boost::hana::Hashable.
Defines boost::hana::fuse.
Adapts boost::fusion::list for use with Hana.
Defines boost::hana::extend.
Defines boost::hana::minus.
Defines boost::hana::detail::has_duplicates.
Defines boost::hana::apply.
Forward declares boost::hana::none.
Defines boost::hana::size.
Defines boost::hana::maximum.
Adaptation of std::ratio for Hana.
Definition: ratio.hpp:58
Tag representing a hana::range.
Definition: range.hpp:87
auto print
Returns a string representation of the given object.
Definition: printable.hpp:69
Defines boost::hana::concat.
Forward declares boost::hana::zip_shortest_with.
Defines boost::hana::detail::index_if.
Defines boost::hana::take_back.
constexpr auto flip
Invoke a function with its two first arguments reversed.
Definition: flip.hpp:31
Forward declares boost::hana::and_.
Defines boost::hana::replicate.
constexpr auto scan_left
Fold a Sequence to the left and return a list containing the successive reduction states.
Definition: scan_left.hpp:86
constexpr auto div
Generalized integer division.
Definition: div.hpp:43
Forward declares boost::hana::eval_if.
Forward declares boost::hana::minimum.
Defines boost::hana::lift.
Defines generally useful preprocessor macros.
Defines boost::hana::suffix.
Defines boost::hana::IntegralConstant.
Forward declares boost::hana::to and related utilities.
constexpr auto to
Converts an object from one data type to another.
Definition: to.hpp:97
Defines boost::hana::remove_if.
constexpr set(set &&other)=default
Move-construct a set from another set. This constructor only exists when all the elements of the set ...
Defines boost::hana::remove_at and boost::hana::remove_at_c.
Forward declares boost::hana::product.
Forward declares boost::hana::scan_right.
Forward declares boost::hana::partition.
times A(T_1) \times \cdots \times A(T_n) \to A(U) @f$. const expr auto ap
Lifted application.
constexpr auto any
Returns whether any key of the structure is true-valued.
Definition: any.hpp:30
Defines boost::hana::first.
constexpr tuple(Xn const &...xn)
Initialize each element of the tuple with the corresponding element from xn.... Only exists when all ...
Forward declares boost::hana::prepend.
Adaptation of std::pair for Hana.
Definition: pair.hpp:35
Forward declares boost::hana::ap.
Forward declares boost::hana::keys.
Defines boost::hana::prepend.
auto BOOST_HANA_DEFINE_STRUCT(...)
Defines members of a structure, while at the same time modeling Struct.
constexpr auto unpack
Invoke a function with the elements of a Foldable as arguments.
Definition: unpack.hpp:79
constexpr auto none
Returns whether all of the keys of the structure are false-valued.
Definition: none.hpp:30
constexpr auto remove_at_c
Equivalent to remove_at; provided for convenience.
Definition: remove_at.hpp:70
Defines a SFINAE-friendly version of std::common_type.
Defines boost::hana::detail::nested_than.
constexpr auto mult
Associative operation of a Ring.
Definition: mult.hpp:47
constexpr auto power
Elevate a ring element to its nth power.
Definition: power.hpp:40
Forward declares boost::hana::Iterable.
Forward declares boost::hana::scan_left.
Forward declares boost::hana::one.
Equivalent to std::common_type, except it is SFINAE-friendly and does not support custom specializati...
Definition: std_common_type.hpp:24
constexpr auto in
Return whether the key occurs in the structure.
Definition: contains.hpp:70
constexpr auto replicate
Create a monadic structure by combining a lifted value with itself n times.
Definition: replicate.hpp:60
Defines macros to perform different kinds of assertions.
Defines boost::hana::scan_left.
auto BOOST_HANA_ADAPT_ADT(...)
Defines a model of Struct with the given accessors.
Documents the BOOST_HANA_DEFINE_STRUCT macro.
Defines boost::hana::ordering.
constexpr auto any_of
Returns whether any key of the structure satisfies the predicate.
Definition: any_of.hpp:37
Defines boost::hana::fold_left.
#define BOOST_HANA_DISPATCH_IF(IMPL,...)
Dispatch to the given implementation method only when a condition is satisfied.
Definition: dispatch_if.hpp:52
Defines boost::hana::detail::variadic::reverse_apply_unrolled.
Defines boost::hana::remove_range and boost::hana::remove_range_c.
Forward declares boost::hana::none_of.
Defines boost::hana::mult.
constexpr auto permutations
Return a sequence of all the permutations of the given sequence.
Definition: permutations.hpp:34
Defines boost::hana::Monoid.
constexpr auto overload_linearly
Call the first function that produces a valid call expression.
Definition: overload_linearly.hpp:38
Implementation of the generic std::make_xxx pattern for arbitrary xxxs.
Definition: create.hpp:22
Defines boost::hana::members.
Defines boost::hana::lockstep.
Forward declares boost::hana::basic_tuple.
Defines boost::hana::replace_if.
typename has_nontrivial_common_embedding_impl< Concept, T, U >::type has_nontrivial_common_embedding
Returns whether T and U are distinct and both have an embedding into a common type.
Definition: has_common_embedding.hpp:66
optional(optional &&)=default
Move-construct an optional. An empty optional may only be move-constructed from another empty optiona...
Defines boost::hana::max.
constexpr map()=default
Default-construct a map. This constructor only exists when all the elements of the map are default-co...
Defines boost::hana::to and related utilities.
Forward declares boost::hana::plus.
Defines boost::hana::eval_if.
Defines boost::hana::detail::CanonicalConstant.
constexpr optional & operator=(optional const &)=default
Copy-assign an optional. An empty optional may only be copy-assigned from another empty optional,...
Forward declares boost::hana::unpack.
Forward declares boost::hana::power.
Defines boost::hana::keys.
Defines boost::hana::value.
Base class of hana::type; used for pattern-matching.
Definition: type.hpp:25
Adapts std::array for use with Hana.
Defines BOOST_HANA_DISPATCH_IF.
Forward declares boost::hana::chain.
Forward declares boost::hana::size.
Defines boost::hana::div.
constexpr auto remove_at
Remove the element at a given index from a sequence.
Definition: remove_at.hpp:46
Defines boost::hana::fix.
Adapter for boost::tuples.
Definition: tuple.hpp:45
constexpr auto drop_front
Drop the first n elements of an iterable, and return the rest.
Definition: drop_front.hpp:47
constexpr tuple & operator=(tuple< Yn... > &&other)
Move-assign a tuple to another tuple. Only exists when all the elements of the destination tuple are ...
constexpr pair()
Default constructs the pair. Only exists when both elements of the pair are default constructible.
Includes all the adaptors for the Boost.Fusion library.
Defines the barebones boost::hana::integral_constant template, but no operations on it.
Classic MPL-style metafunction returning the nth element of a type parameter pack.
Definition: type_at.hpp:47
constexpr auto operator+() const
Returns rvalue of self. See description.
Adapts std::integer_sequence for use with Hana.
constexpr auto reverse
Reverse a sequence.
Definition: reverse.hpp:33
Defines boost::hana::not_equal.
Forward declares boost::hana::insert_range.
Defines boost::hana::Functor.
Defines boost::hana::none.
Defines boost::hana::difference.
Forward declares boost::hana::Comonad.
Adapts Boost.MPL IntegralConstants for use with Hana.
Adapter for std::tuples.
Definition: tuple.hpp:49
Forward declares boost::hana::Foldable.
Defines boost::hana::Product.
Defines boost::hana::Iterable.
constexpr tuple()
Default constructs the tuple. Only exists when all the elements of the tuple are default constructibl...
Definition: common.hpp:67
hana::lazy implements superficial laziness via a monadic interface.
Definition: lazy.hpp:71
Defines boost::hana::fold.
Defines boost::hana::sum.
Defines boost::hana::Ring.
Forward declares boost::hana::tuple.
Forward declares boost::hana::suffix.
Forward declares boost::hana::extend.
Defines boost::hana::is_disjoint.
Tag representing hana::lazy.
Definition: lazy.hpp:85
Forward declares boost::hana::min.
Forward declares boost::hana::max.
constexpr auto greater_equal
Returns a Logical representing whether x is greater than or equal to y.
Definition: greater_equal.hpp:38
Defines boost::hana::detail::has_[nontrivial_]common_embedding.
constexpr auto at
Returns the nth element of an iterable.
Definition: at.hpp:50
Defines boost::hana::remove.
Defines boost::hana::at and boost::hana::at_c.
Forward declares boost::hana::value.
constexpr auto repeat
Invokes a nullary function n times.
Definition: repeat.hpp:42
constexpr auto infix
Return an equivalent function that can also be applied in infix notation.
Definition: infix.hpp:79
Defines boost::hana::Struct.
Defines boost::hana::empty.
constexpr auto comparing
Returns a function performing equal after applying a transformation to both arguments.
Definition: comparing.hpp:50
Tag representing hana::maps.
Definition: map.hpp:24
constexpr auto and_
Return whether all the arguments are true-valued.
Definition: and.hpp:34
Defines boost::hana::prefix.
Defines boost::hana::detail::any_of.
constexpr optional(T const &t)
Construct an optional holding a value of type T from another object of type T. The value is copy-cons...
Definition: optional.hpp:161
Defines boost::hana::overload_linearly.
constexpr auto remove_range_c
Equivalent to remove_range; provided for convenience.
Definition: remove_range.hpp:73
typename hana::tag_of< T >::type tag_of_t
Alias to tag_of<T>::type, provided for convenience.
Definition: tag_of.hpp:117
constexpr auto count
Return the number of elements in the structure that compare equal to a given value.
Definition: count.hpp:41
constexpr auto mod
Generalized integer modulus.
Definition: mod.hpp:46
Defines boost::hana::second.
Defines boost::hana::cartesian_product.
constexpr auto empty
Identity of the monadic combination concat.
Definition: empty.hpp:36
Forward declares boost::hana::fill.
Defines a replacement for std::decay, which is sometimes too slow at compile-time.
Adapter for Boost.MPL vectors.
Definition: vector.hpp:92
Defines boost::hana::detail::nested_to.
constexpr pair(First const &first, Second const &second)
Initialize each element of the pair with the corresponding element. Only exists when both elements of...
Defines boost::hana::detail::variadic::split_at.
Includes all the headers needed to setup tag-dispatching.
Forward declares boost::hana::Constant.
constexpr auto decltype_
decltype keyword, lifted to Hana.
Definition: type.hpp:190
constexpr auto insert_range
Insert several values at a given index in a sequence.
Definition: insert_range.hpp:41
Defines boost::hana::drop_front_exactly.
constexpr tuple(tuple< Yn... > &&other)
Move-initialize a tuple from another tuple. Only exists when all the elements of the constructed tupl...
Forward declares boost::hana::Product.
Forward declares boost::hana::any_of.
Defines boost::hana::symmetric_difference.
Forward declares boost::hana::range.
constexpr pair(pair< T, U > &&other)
Move-initialize a pair from another pair. Only exists when both elements of the source pair are impli...
Forward declares boost::hana::first.
Defines boost::hana::is_subset.
constexpr type< T > type_c
Creates an object representing the C++ type T.
Definition: type.hpp:128
Forward declares boost::hana::Comparable.
constexpr auto all_of
Returns whether all the keys of the structure satisfy the predicate.
Definition: all_of.hpp:38
Defines boost::hana::detail::hash_table.
Defines boost::hana::monadic_fold_left.
constexpr auto suffix
Inserts a value after each element of a monadic structure.
Definition: suffix.hpp:56
constexpr auto is_subset
Returns whether a structure contains a subset of the keys of another structure.
Definition: is_subset.hpp:63
constexpr auto all
Returns whether all the keys of the structure are true-valued.
Definition: all.hpp:30
constexpr auto contains
Returns whether the key occurs in the structure.
Definition: contains.hpp:42
Forward declares boost::hana::IntegralConstant.
Defines boost::hana::reverse_fold.
Defines boost::hana::is_empty.
Forward declares boost::hana::Monad.
Defines boost::hana::fill.
constexpr auto typeid_
Returns a hana::type representing the type of a given object.
Definition: type.hpp:233
Defines boost::hana::partition.
Returns whether a data type can be embedded into another data type.
Definition: to.hpp:162
Metafunction returning the common data type between two data types.
Definition: common.hpp:68
constexpr auto while_
Apply a function to an initial state while some predicate is satisfied.
Definition: while.hpp:55
Defines boost::hana::front.
constexpr map & operator=(map const &other)
Assign a map to another map with the exact same type. Only exists when all the elements of the map ar...
Defines arithmetic operators.
Forward declares boost::hana::index_if.
Forward declares boost::hana::zero.
Defines boost::hana::monadic_compose.
Forward declares boost::hana::Applicative.
Defines boost::hana::none_of.
Defines boost::hana::at_key.
Defines boost::hana::minimum.
constexpr auto not_equal
Returns a Logical representing whether x is not equal to y.
Definition: not_equal.hpp:54
Forward declares boost::hana::all_of.
Forward declares boost::hana::comparing.
Adapts boost::fusion::tuple for use with Hana.
Defines boost::hana::length.
constexpr friend auto operator|(tuple< T... >, F)
Equivalent to hana::chain.
Defines boost::hana::while_.
Forward declares boost::hana::drop_front_exactly.
Forward declares boost::hana::is_a and boost::hana::is_an.
Forward declares boost::hana::Hashable.
Defines boost::hana::sort.
Forward declares boost::hana::Group.
Defines boost::hana::fold_right.
Forward declares boost::hana::duplicate.
Adaptation of std::array for Hana.
Definition: array.hpp:64
Defines boost::hana::overload.
Stripped down version of hana::tuple.
Definition: basic_tuple.hpp:35
Forward declares boost::hana::accessors.
Defines boost::hana::zip_shortest.
Defines boost::hana::detail::unpack_flatten.
Defines boost::hana::Comparable.
Adapts boost::mpl::vector for use with Hana.
Defines boost::hana::intersperse.
Adapter for std::integral_constants.
Definition: integral_constant.hpp:37
constexpr auto cycle
Combine a monadic structure with itself n times.
Definition: cycle.hpp:60
Adapts std::integral_constant for use with Hana.
Forward declares boost::hana::lazy.
General purpose index-based heterogeneous sequence with a fixed length.
Definition: tuple.hpp:69
Adapts boost::fusion::deque for use with Hana.
constexpr auto first
Returns the first element of a pair.
Definition: first.hpp:33
constexpr auto zip_shortest
Zip one sequence or more.
Definition: zip_shortest.hpp:45
Forward declares boost::hana::members.
constexpr auto slice
Extract the elements of a Sequence at the given indices.
Definition: slice.hpp:53
constexpr tuple & operator=(tuple< Yn... > const &other)
Assign a tuple to another tuple. Only exists when all the elements of the destination tuple are assig...
Forward declares boost::hana::any.
constexpr auto take_back_c
Equivalent to take_back; provided for convenience.
Definition: take_back.hpp:66
Forward declares boost::hana::front.
Adapts std::tuple for use with Hana.
Compile-time string.
Definition: string.hpp:117
Defines boost::hana::duplicate.
Defines boost::hana::not_.
Defines boost::hana::for_each.
Defines boost::hana::insert.
constexpr auto make< type_tag >
Equivalent to decltype_, provided for convenience.
Definition: type.hpp:252
Forward declares boost::hana::for_each.
Defines boost::hana::adjust.
Forward declares boost::hana::Sequence.
Forward declares boost::hana::all.
Defines function-like equivalents to the standard <type_traits>, and also to some utilities like std:...
constexpr auto symmetric_difference
Returns the symmetric set-theoretic difference of two maps.
Definition: map.hpp:374
constexpr map(map const &other)=default
Copy-construct a map from another map. This constructor only exists when all the elements of the map ...
Forward declares boost::hana::permutations.
Forward declares boost::hana::remove_if.
Forward declares boost::hana::type and related utilities.
Forward declares boost::hana::default_ and boost::hana::is_default.
Forward declares boost::hana::Struct.
Tag representing a canonical Constant.
Definition: canonical_constant.hpp:26
constexpr T & value()
Extract the content of an optional, or fail at compile-time.
constexpr auto drop_back
Drop the last n elements of a finite sequence, and return the rest.
Definition: drop_back.hpp:44
constexpr auto sizeof_
sizeof keyword, lifted to Hana.
Definition: type.hpp:290
Forward declares boost::hana::tap.
Forward declares boost::hana::set.
constexpr auto take_front_c
Equivalent to take_front; provided for convenience.
Definition: take_front.hpp:66
Defines boost::hana::demux.
Defines boost::hana::greater_equal.
constexpr auto overload
Pick one of several functions to call based on overload resolution.
Definition: overload.hpp:35
Tag representing hana::type.
Definition: type.hpp:123
Defines boost::hana::Group.
constexpr auto fuse
Transform a function taking multiple arguments into a function that can be called with a compile-time...
Definition: fuse.hpp:40
Defines boost::hana::Comonad.
Defines boost::hana::any.
Defines boost::hana::union.
Forward declares boost::hana::minus.
constexpr auto filter
Filter a monadic structure using a custom predicate.
Definition: filter.hpp:65
constexpr auto back
Returns the last element of a non-empty and finite iterable.
Definition: back.hpp:32
Forward declares boost::hana::Logical.
Defines boost::hana::pair.
Master header for the boost/hana/concept/ subdirectory.
Forward declares boost::hana::repeat.
constexpr auto partial
Partially apply a function to some arguments.
Definition: partial.hpp:43
Defines boost::hana::span.
Forward declares boost::hana::MonadPlus.
Forward declares boost::hana::maximum.
Forward declares boost::hana::empty.
Forward declares boost::hana::erase_key.
Tag representing a compile-time string.
Definition: string.hpp:166
constexpr auto apply
Invokes a Callable with the given arguments.
Definition: apply.hpp:40
Defines boost::hana::curry.
Defines boost::hana::drop_while.
Tag representing hana::tuples.
Definition: tuple.hpp:159
Defines boost::hana::lazy.
constexpr keys_t keys
Returns a Sequence containing the name of the members of the data structure.
Definition: keys.hpp:29
Defines operators for Monads.
Defines boost::hana::scan_right.
Defines boost::hana::product.
constexpr auto lockstep
Invoke a function with the result of invoking other functions on its arguments, in lockstep.
Definition: lockstep.hpp:39
Defines operators for Iterables.
Forward declares boost::hana::slice and boost::hana::slice_c.
C++ type in value-level representation.
Definition: type.hpp:100
Defines boost::hana::default_ and boost::hana::is_default.
Forward declares boost::hana::take_while.
Generic container for two elements.
Definition: pair.hpp:61
Forward declares boost::hana::zip.
Forward declares boost::hana::prefix.
Defines boost::hana::append.
Forward declares boost::hana::detail::nested_by.
Defines boost::hana::or_.
constexpr auto product
Compute the product of the numbers of a structure.
Definition: product.hpp:57
constexpr auto at_key
Returns the value associated to the given key in a structure, or fail.
Definition: at_key.hpp:51
Adapts std::ratio for use with Hana.
constexpr auto remove_range
Remove the elements inside a given range of indices from a sequence.
Definition: remove_range.hpp:49
Forward declares boost::hana::at and boost::hana::at_c.
constexpr auto intersection
Returns the intersection of two maps.
Definition: map.hpp:315
Forward declares boost::hana::unique.
Tag representing hana::pair.
Definition: pair.hpp:131
Defines boost::hana::all.
constexpr auto accessors
Returns a Sequence of pairs representing the accessors of the data structure.
Definition: accessors.hpp:35
Basic unordered container requiring unique, Comparable and Hashable keys.
Definition: set.hpp:75
typename common< T, U >::type common_t
Alias to common<T, U>::type, provided for convenience.
Definition: common.hpp:100
Defines boost::hana::monadic_fold_right.
constexpr auto greater
Returns a Logical representing whether x is greater than y.
Definition: greater.hpp:37
constexpr optional & operator=(optional &&)=default
Move-assign an optional. An empty optional may only be move-assigned from another empty optional,...
constexpr auto equal
Returns a Logical representing whether x is equal to y.
Definition: equal.hpp:64
Forward declares boost::hana::symmetric_difference.
constexpr map(P &&...pairs)
Construct the map from the provided pairs. P... must be pairs of the same type (modulo ref and cv-qua...
Tag representing hana::integral_constant.
Definition: integral_constant.hpp:24
Forward declares boost::hana::while_.
static constexpr void times(F &&f)
Call a function n times.
Definition: integral_constant.hpp:168
Defines boost::hana::compose.
Adapts boost::fusion::vector for use with Hana.
constexpr auto prefix
Inserts a value before each element of a monadic structure.
Definition: prefix.hpp:56
Defines boost::hana::unpack.
Defines boost::hana::MonadPlus.
Defines boost::hana::Searchable.
Defines boost::hana::iterate.
Defines boost::hana::optional.
constexpr tuple(Yn &&...yn)
Initialize each element of the tuple by perfect-forwarding the corresponding element in yn....