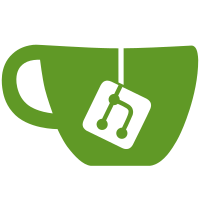
Reason: Unfortunately we depend on AppRTC being in this location for the bots in our Chromium WebRTC waterfalls so I'm reverting this until we've solved that dependency. This reverts apprtc and adapter.js from being removed in r5871. R=phoglund@webrtc.org TBR=dutton@google.com BUG= Review URL: https://webrtc-codereview.appspot.com/11529004 git-svn-id: http://webrtc.googlecode.com/svn/trunk@5873 4adac7df-926f-26a2-2b94-8c16560cd09d
199 lines
6.2 KiB
JavaScript
199 lines
6.2 KiB
JavaScript
var RTCPeerConnection = null;
|
|
var getUserMedia = null;
|
|
var attachMediaStream = null;
|
|
var reattachMediaStream = null;
|
|
var webrtcDetectedBrowser = null;
|
|
var webrtcDetectedVersion = null;
|
|
|
|
function trace(text) {
|
|
// This function is used for logging.
|
|
if (text[text.length - 1] == '\n') {
|
|
text = text.substring(0, text.length - 1);
|
|
}
|
|
console.log((performance.now() / 1000).toFixed(3) + ": " + text);
|
|
}
|
|
function maybeFixConfiguration(pcConfig) {
|
|
if (pcConfig == null) {
|
|
return;
|
|
}
|
|
for (var i = 0; i < pcConfig.iceServers.length; i++) {
|
|
if (pcConfig.iceServers[i].hasOwnProperty('urls')){
|
|
pcConfig.iceServers[i]['url'] = pcConfig.iceServers[i]['urls'];
|
|
delete pcConfig.iceServers[i]['urls'];
|
|
}
|
|
}
|
|
}
|
|
|
|
if (navigator.mozGetUserMedia) {
|
|
console.log("This appears to be Firefox");
|
|
|
|
webrtcDetectedBrowser = "firefox";
|
|
|
|
webrtcDetectedVersion =
|
|
parseInt(navigator.userAgent.match(/Firefox\/([0-9]+)\./)[1], 10);
|
|
|
|
// The RTCPeerConnection object.
|
|
var RTCPeerConnection = function(pcConfig, pcConstraints) {
|
|
// .urls is not supported in FF yet.
|
|
maybeFixConfiguration(pcConfig);
|
|
return new mozRTCPeerConnection(pcConfig, pcConstraints);
|
|
}
|
|
|
|
// The RTCSessionDescription object.
|
|
RTCSessionDescription = mozRTCSessionDescription;
|
|
|
|
// The RTCIceCandidate object.
|
|
RTCIceCandidate = mozRTCIceCandidate;
|
|
|
|
// Get UserMedia (only difference is the prefix).
|
|
// Code from Adam Barth.
|
|
getUserMedia = navigator.mozGetUserMedia.bind(navigator);
|
|
navigator.getUserMedia = getUserMedia;
|
|
|
|
// Creates iceServer from the url for FF.
|
|
createIceServer = function(url, username, password) {
|
|
var iceServer = null;
|
|
var url_parts = url.split(':');
|
|
if (url_parts[0].indexOf('stun') === 0) {
|
|
// Create iceServer with stun url.
|
|
iceServer = { 'url': url };
|
|
} else if (url_parts[0].indexOf('turn') === 0) {
|
|
if (webrtcDetectedVersion < 27) {
|
|
// Create iceServer with turn url.
|
|
// Ignore the transport parameter from TURN url for FF version <=27.
|
|
var turn_url_parts = url.split("?");
|
|
// Return null for createIceServer if transport=tcp.
|
|
if (turn_url_parts.length === 1 ||
|
|
turn_url_parts[1].indexOf('transport=udp') === 0) {
|
|
iceServer = {'url': turn_url_parts[0],
|
|
'credential': password,
|
|
'username': username};
|
|
}
|
|
} else {
|
|
// FF 27 and above supports transport parameters in TURN url,
|
|
// So passing in the full url to create iceServer.
|
|
iceServer = {'url': url,
|
|
'credential': password,
|
|
'username': username};
|
|
}
|
|
}
|
|
return iceServer;
|
|
};
|
|
|
|
createIceServers = function(urls, username, password) {
|
|
var iceServers = [];
|
|
// Use .url for FireFox.
|
|
for (i = 0; i < urls.length; i++) {
|
|
var iceServer = createIceServer(urls[i],
|
|
username,
|
|
password);
|
|
if (iceServer !== null) {
|
|
iceServers.push(iceServer);
|
|
}
|
|
}
|
|
return iceServers;
|
|
}
|
|
|
|
// Attach a media stream to an element.
|
|
attachMediaStream = function(element, stream) {
|
|
console.log("Attaching media stream");
|
|
element.mozSrcObject = stream;
|
|
element.play();
|
|
};
|
|
|
|
reattachMediaStream = function(to, from) {
|
|
console.log("Reattaching media stream");
|
|
to.mozSrcObject = from.mozSrcObject;
|
|
to.play();
|
|
};
|
|
|
|
// Fake get{Video,Audio}Tracks
|
|
if (!MediaStream.prototype.getVideoTracks) {
|
|
MediaStream.prototype.getVideoTracks = function() {
|
|
return [];
|
|
};
|
|
}
|
|
|
|
if (!MediaStream.prototype.getAudioTracks) {
|
|
MediaStream.prototype.getAudioTracks = function() {
|
|
return [];
|
|
};
|
|
}
|
|
} else if (navigator.webkitGetUserMedia) {
|
|
console.log("This appears to be Chrome");
|
|
|
|
webrtcDetectedBrowser = "chrome";
|
|
webrtcDetectedVersion =
|
|
parseInt(navigator.userAgent.match(/Chrom(e|ium)\/([0-9]+)\./)[2], 10);
|
|
|
|
// Creates iceServer from the url for Chrome M33 and earlier.
|
|
createIceServer = function(url, username, password) {
|
|
var iceServer = null;
|
|
var url_parts = url.split(':');
|
|
if (url_parts[0].indexOf('stun') === 0) {
|
|
// Create iceServer with stun url.
|
|
iceServer = { 'url': url };
|
|
} else if (url_parts[0].indexOf('turn') === 0) {
|
|
// Chrome M28 & above uses below TURN format.
|
|
iceServer = {'url': url,
|
|
'credential': password,
|
|
'username': username};
|
|
}
|
|
return iceServer;
|
|
};
|
|
|
|
// Creates iceServers from the urls for Chrome M34 and above.
|
|
createIceServers = function(urls, username, password) {
|
|
var iceServers = [];
|
|
if (webrtcDetectedVersion >= 34) {
|
|
// .urls is supported since Chrome M34.
|
|
iceServers = {'urls': urls,
|
|
'credential': password,
|
|
'username': username };
|
|
} else {
|
|
for (i = 0; i < urls.length; i++) {
|
|
var iceServer = createIceServer(urls[i],
|
|
username,
|
|
password);
|
|
if (iceServer !== null) {
|
|
iceServers.push(iceServer);
|
|
}
|
|
}
|
|
}
|
|
return iceServers;
|
|
};
|
|
|
|
// The RTCPeerConnection object.
|
|
var RTCPeerConnection = function(pcConfig, pcConstraints) {
|
|
// .urls is supported since Chrome M34.
|
|
if (webrtcDetectedVersion < 34) {
|
|
maybeFixConfiguration(pcConfig);
|
|
}
|
|
return new webkitRTCPeerConnection(pcConfig, pcConstraints);
|
|
}
|
|
|
|
// Get UserMedia (only difference is the prefix).
|
|
// Code from Adam Barth.
|
|
getUserMedia = navigator.webkitGetUserMedia.bind(navigator);
|
|
navigator.getUserMedia = getUserMedia;
|
|
|
|
// Attach a media stream to an element.
|
|
attachMediaStream = function(element, stream) {
|
|
if (typeof element.srcObject !== 'undefined') {
|
|
element.srcObject = stream;
|
|
} else if (typeof element.mozSrcObject !== 'undefined') {
|
|
element.mozSrcObject = stream;
|
|
} else if (typeof element.src !== 'undefined') {
|
|
element.src = URL.createObjectURL(stream);
|
|
} else {
|
|
console.log('Error attaching stream to element.');
|
|
}
|
|
};
|
|
|
|
reattachMediaStream = function(to, from) {
|
|
to.src = from.src;
|
|
};
|
|
} else {
|
|
console.log("Browser does not appear to be WebRTC-capable");
|
|
}
|