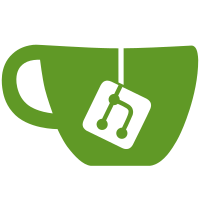
The Reset function was modified so that the encoder is destroyed and recreated on reset. Initialization of the encoder and setting of the encoder speed is now done in a private method, to avoid code duplication. (It is used both in InitEncode and in Reset.) This change is needed to make the unit tests pass with newer versions of libvpx. Review URL: http://webrtc-codereview.appspot.com/33004 git-svn-id: http://webrtc.googlecode.com/svn/trunk@56 4adac7df-926f-26a2-2b94-8c16560cd09d
228 lines
8.6 KiB
C++
228 lines
8.6 KiB
C++
/*
|
|
* Copyright (c) 2011 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
/*
|
|
* vp8.h
|
|
* WEBRTC VP8 wrapper interface
|
|
*/
|
|
|
|
|
|
#ifndef WEBRTC_MODULES_VIDEO_CODING_CODECS_VP8_H_
|
|
#define WEBRTC_MODULES_VIDEO_CODING_CODECS_VP8_H_
|
|
|
|
#include "video_codec_interface.h"
|
|
|
|
// VPX forward declaration
|
|
typedef struct vpx_codec_ctx vpx_codec_ctx_t;
|
|
typedef struct vpx_codec_ctx vpx_dec_ctx_t;
|
|
typedef struct vpx_codec_enc_cfg vpx_codec_enc_cfg_t;
|
|
typedef struct vpx_image vpx_image_t;
|
|
|
|
namespace webrtc
|
|
{
|
|
|
|
/******************************/
|
|
/* VP8Encoder class */
|
|
/******************************/
|
|
class VP8Encoder : public VideoEncoder
|
|
{
|
|
public:
|
|
VP8Encoder();
|
|
virtual ~VP8Encoder();
|
|
|
|
// Free encoder memory.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK, < 0 otherwise.
|
|
virtual WebRtc_Word32 Release();
|
|
|
|
// Reset encoder state and prepare for a new call.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK, < 0 otherwise.
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERR_PARAMETER
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
virtual WebRtc_Word32 Reset();
|
|
|
|
// Initialize the encoder with the information from the codecSettings
|
|
//
|
|
// Input:
|
|
// - codecSettings : Codec settings
|
|
// - numberOfCores : Number of cores available for the encoder
|
|
// - maxPayloadSize : The maximum size each payload is allowed
|
|
// to have. Usually MTU - overhead.
|
|
//
|
|
// Return value : Set bit rate if OK
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERR_PARAMETER
|
|
// WEBRTC_VIDEO_CODEC_ERR_SIZE
|
|
// WEBRTC_VIDEO_CODEC_LEVEL_EXCEEDED
|
|
// WEBRTC_VIDEO_CODEC_MEMORY
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
virtual WebRtc_Word32 InitEncode(const VideoCodec* codecSettings,
|
|
WebRtc_Word32 numberOfCores,
|
|
WebRtc_UWord32 maxPayloadSize);
|
|
|
|
// Encode an I420 image (as a part of a video stream). The encoded image
|
|
// will be returned to the user through the encode complete callback.
|
|
//
|
|
// Input:
|
|
// - inputImage : Image to be encoded
|
|
// - frameTypes : Frame type to be generated by the encoder.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERR_PARAMETER
|
|
// WEBRTC_VIDEO_CODEC_MEMORY
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
// WEBRTC_VIDEO_CODEC_TIMEOUT
|
|
|
|
virtual WebRtc_Word32 Encode(const RawImage& inputImage,
|
|
const void* codecSpecificInfo,
|
|
VideoFrameType frameType);
|
|
|
|
// Register an encode complete callback object.
|
|
//
|
|
// Input:
|
|
// - callback : Callback object which handles encoded images.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK, < 0 otherwise.
|
|
virtual WebRtc_Word32 RegisterEncodeCompleteCallback(EncodedImageCallback* callback);
|
|
|
|
// Inform the encoder of the new packet loss rate in the network
|
|
//
|
|
// - packetLoss : Fraction lost
|
|
// (loss rate in percent = 100 * packetLoss / 255)
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
//
|
|
virtual WebRtc_Word32 SetPacketLoss(WebRtc_UWord32 packetLoss);
|
|
|
|
// Inform the encoder about the new target bit rate.
|
|
//
|
|
// - newBitRate : New target bit rate
|
|
// - frameRate : The target frame rate
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK, < 0 otherwise.
|
|
virtual WebRtc_Word32 SetRates(WebRtc_UWord32 newBitRateKbit, WebRtc_UWord32 frameRate);
|
|
|
|
// Get version number for the codec.
|
|
//
|
|
// Input:
|
|
// - version : Pointer to allocated char buffer.
|
|
// - buflen : Length of provided char buffer.
|
|
//
|
|
// Output:
|
|
// - version : Version number string written to char buffer.
|
|
//
|
|
// Return value : >0 - Length of written string.
|
|
// <0 - WEBRTC_VIDEO_CODEC_ERR_SIZE
|
|
virtual WebRtc_Word32 Version(WebRtc_Word8 *version, WebRtc_Word32 length) const;
|
|
static WebRtc_Word32 VersionStatic(WebRtc_Word8 *version, WebRtc_Word32 length);
|
|
|
|
private:
|
|
// Call encoder initialize function and set speed.
|
|
WebRtc_Word32 InitAndSetSpeed();
|
|
|
|
EncodedImage _encodedImage;
|
|
EncodedImageCallback* _encodedCompleteCallback;
|
|
WebRtc_Word32 _width;
|
|
WebRtc_Word32 _height;
|
|
WebRtc_Word32 _maxBitRateKbit;
|
|
int _maxFrameRate;
|
|
bool _inited;
|
|
WebRtc_UWord16 _pictureID;
|
|
bool _pictureLossIndicationOn;
|
|
bool _feedbackModeOn;
|
|
bool _nextRefIsGolden;
|
|
bool _lastAcknowledgedIsGolden;
|
|
bool _haveReceivedAcknowledgement;
|
|
WebRtc_UWord16 _pictureIDLastSentRef;
|
|
WebRtc_UWord16 _pictureIDLastAcknowledgedRef;
|
|
int _cpuSpeed;
|
|
|
|
vpx_codec_ctx_t* _encoder;
|
|
vpx_codec_enc_cfg_t* _cfg;
|
|
vpx_image_t* _raw;
|
|
};// end of VP8Encoder class
|
|
|
|
/******************************/
|
|
/* VP8Decoder class */
|
|
/******************************/
|
|
class VP8Decoder : public VideoDecoder
|
|
{
|
|
public:
|
|
VP8Decoder();
|
|
virtual ~VP8Decoder();
|
|
|
|
// Initialize the decoder.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK.
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
virtual WebRtc_Word32 InitDecode(const VideoCodec* inst,
|
|
WebRtc_Word32 numberOfCores);
|
|
|
|
// Decode encoded image (as a part of a video stream). The decoded image
|
|
// will be returned to the user through the decode complete callback.
|
|
//
|
|
// Input:
|
|
// - inputImage : Encoded image to be decoded
|
|
// - missingFrames : True if one or more frames have been lost
|
|
// since the previous decode call.
|
|
// - codecSpecificInfo : pointer to specific codec data
|
|
// - renderTimeMs : Render time in Ms
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
// WEBRTC_VIDEO_CODEC_ERR_PARAMETER
|
|
virtual WebRtc_Word32 Decode(const EncodedImage& inputImage,
|
|
bool missingFrames,
|
|
const void* /*codecSpecificInfo*/,
|
|
WebRtc_Word64 /*renderTimeMs*/);
|
|
|
|
// Register a decode complete callback object.
|
|
//
|
|
// Input:
|
|
// - callback : Callback object which handles decoded images.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK, < 0 otherwise.
|
|
virtual WebRtc_Word32 RegisterDecodeCompleteCallback(DecodedImageCallback* callback);
|
|
|
|
// Free decoder memory.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK if OK
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
virtual WebRtc_Word32 Release();
|
|
|
|
// Reset decoder state and prepare for a new call.
|
|
//
|
|
// Return value : WEBRTC_VIDEO_CODEC_OK.
|
|
// <0 - Errors:
|
|
// WEBRTC_VIDEO_CODEC_UNINITIALIZED
|
|
// WEBRTC_VIDEO_CODEC_ERROR
|
|
virtual WebRtc_Word32 Reset();
|
|
virtual WebRtc_Word32 SetCodecConfigParameters(WebRtc_UWord8* /*buffer*/, WebRtc_Word32 /*size*/) { return -1; }
|
|
|
|
private:
|
|
RawImage _decodedImage;
|
|
DecodedImageCallback* _decodeCompleteCallback;
|
|
bool _inited;
|
|
bool _feedbackModeOn;
|
|
vpx_dec_ctx_t* _decoder;
|
|
|
|
};// end of VP8Decoder class
|
|
|
|
} // namespace webrtc
|
|
|
|
#endif // WEBRTC_MODULES_VIDEO_CODING_CODECS_VP8_H_
|