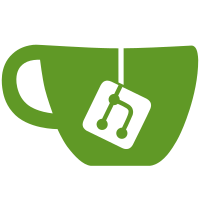
TBR=phoglund BUG=none TEST=Tested with local modifications on the live installation. Review URL: https://webrtc-codereview.appspot.com/675005 git-svn-id: http://webrtc.googlecode.com/svn/trunk@2496 4adac7df-926f-26a2-2b94-8c16560cd09d
54 lines
1.9 KiB
Python
54 lines
1.9 KiB
Python
#!/usr/bin/env python
|
|
#-*- coding: utf-8 -*-
|
|
# Copyright (c) 2012 The WebRTC project authors. All Rights Reserved.
|
|
#
|
|
# Use of this source code is governed by a BSD-style license
|
|
# that can be found in the LICENSE file in the root of the source
|
|
# tree. An additional intellectual property rights grant can be found
|
|
# in the file PATENTS. All contributing project authors may
|
|
# be found in the AUTHORS file in the root of the source tree.
|
|
|
|
"""Unit test for the build status tracker script."""
|
|
|
|
|
|
import copy
|
|
import unittest
|
|
|
|
import track_build_status
|
|
|
|
|
|
NORMAL_BOT_TO_STATUS_MAPPING = {'1455--ChromeOS': '455--OK',
|
|
'1455--Chrome': '900--failed',
|
|
'1455--Linux32DBG': '344--OK',
|
|
'1456--ChromeOS': '456--OK'}
|
|
|
|
|
|
class TrackBuildStatusTest(unittest.TestCase):
|
|
|
|
def test_that_filter_chrome_only_builds_filter_properly(self):
|
|
bot_to_status_mapping = copy.deepcopy(NORMAL_BOT_TO_STATUS_MAPPING)
|
|
bot_to_status_mapping['133445--Chrome'] = '901--OK'
|
|
bot_to_status_mapping['133441--ChromeBloat'] = '344--OK'
|
|
|
|
result = track_build_status._filter_chrome_only_builds(
|
|
bot_to_status_mapping)
|
|
|
|
self.assertEquals(NORMAL_BOT_TO_STATUS_MAPPING, result)
|
|
|
|
def test_ensure_filter_chrome_only_builds_doesnt_filter_too_much(self):
|
|
result = track_build_status._filter_chrome_only_builds(
|
|
NORMAL_BOT_TO_STATUS_MAPPING)
|
|
|
|
self.assertEquals(NORMAL_BOT_TO_STATUS_MAPPING, result)
|
|
|
|
def test_get_desired_bots(self):
|
|
bot_to_status_mapping = copy.deepcopy(NORMAL_BOT_TO_STATUS_MAPPING)
|
|
desired_bot_names = ['Linux32DBG']
|
|
result = track_build_status._get_desired_bots(bot_to_status_mapping,
|
|
desired_bot_names)
|
|
self.assertEquals(1, len(result))
|
|
self.assertTrue(desired_bot_names[0] in result.keys()[0])
|
|
|
|
if __name__ == '__main__':
|
|
unittest.main()
|