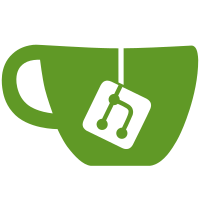
git-svn-id: http://webrtc.googlecode.com/svn/trunk@66 4adac7df-926f-26a2-2b94-8c16560cd09d
157 lines
3.7 KiB
C++
157 lines
3.7 KiB
C++
/*
|
|
* Copyright (c) 2011 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include "audio_device_test_defines.h"
|
|
#include "func_test_manager.h"
|
|
|
|
// Disable warning message 4996 ('scanf': This function or variable may be unsafe)
|
|
#pragma warning( disable : 4996 )
|
|
|
|
using namespace webrtc;
|
|
|
|
int func_test(int);
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// main()
|
|
// ----------------------------------------------------------------------------
|
|
|
|
#if !defined(MAC_IPHONE) && !defined(ANDROID)
|
|
int main(int /*argc*/, char* /*argv*/[])
|
|
{
|
|
func_test(0);
|
|
}
|
|
#endif
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// func_test()
|
|
// ----------------------------------------------------------------------------
|
|
|
|
int func_test(int sel)
|
|
{
|
|
TEST_LOG("=========================================\n");
|
|
TEST_LOG("Func Test of the WebRtcAudioDevice Module\n");
|
|
TEST_LOG("=========================================\n\n");
|
|
|
|
FuncTestManager funcMgr;
|
|
|
|
funcMgr.Init();
|
|
|
|
bool quit(false);
|
|
|
|
while (!quit)
|
|
{
|
|
TEST_LOG("---------------------------------------\n");
|
|
TEST_LOG("Select type of test\n\n");
|
|
TEST_LOG(" (0) Quit\n");
|
|
TEST_LOG(" (1) All\n");
|
|
TEST_LOG("- - - - - - - - - - - - - - - - - - - -\n");
|
|
TEST_LOG(" (2) Audio-layer selection\n");
|
|
TEST_LOG(" (3) Device enumeration\n");
|
|
TEST_LOG(" (4) Device selection\n");
|
|
TEST_LOG(" (5) Audio transport\n");
|
|
TEST_LOG(" (6) Speaker volume\n");
|
|
TEST_LOG(" (7) Microphone volume\n");
|
|
TEST_LOG(" (8) Speaker mute\n");
|
|
TEST_LOG(" (9) Microphone mute\n");
|
|
TEST_LOG(" (10) Microphone boost\n");
|
|
TEST_LOG(" (11) Microphone AGC\n");
|
|
TEST_LOG(" (12) Loopback measurements\n");
|
|
TEST_LOG(" (13) Device removal\n");
|
|
TEST_LOG(" (14) Advanced mobile device API\n");
|
|
TEST_LOG(" (66) XTEST\n");
|
|
TEST_LOG("- - - - - - - - - - - - - - - - - - - -\n");
|
|
TEST_LOG("\n: ");
|
|
|
|
int dummy(0);
|
|
int selection(0);
|
|
enum TestType testType(TTInvalid);
|
|
|
|
SHOW_MENU:
|
|
|
|
if (sel > 0)
|
|
{
|
|
selection = sel;
|
|
}
|
|
else
|
|
{
|
|
dummy = scanf("%d", &selection);
|
|
}
|
|
|
|
switch (selection)
|
|
{
|
|
case 0:
|
|
quit = true;
|
|
break;
|
|
case 1:
|
|
testType = TTAll;
|
|
break;
|
|
case 2:
|
|
testType = TTAudioLayerSelection;
|
|
break;
|
|
case 3:
|
|
testType = TTDeviceEnumeration;
|
|
break;
|
|
case 4:
|
|
testType = TTDeviceSelection;
|
|
break;
|
|
case 5:
|
|
testType = TTAudioTransport;
|
|
break;
|
|
case 6:
|
|
testType = TTSpeakerVolume;
|
|
break;
|
|
case 7:
|
|
testType = TTMicrophoneVolume;
|
|
break;
|
|
case 8:
|
|
testType = TTSpeakerMute;
|
|
break;
|
|
case 9:
|
|
testType = TTMicrophoneMute;
|
|
break;
|
|
case 10:
|
|
testType = TTMicrophoneBoost;
|
|
break;
|
|
case 11:
|
|
testType = TTMicrophoneAGC;
|
|
break;
|
|
case 12:
|
|
testType = TTLoopback;
|
|
break;
|
|
case 13:
|
|
testType = TTDeviceRemoval;
|
|
break;
|
|
case 14:
|
|
testType = TTMobileAPI;
|
|
break;
|
|
case 66:
|
|
testType = TTTest;
|
|
break;
|
|
default:
|
|
testType = TTInvalid;
|
|
TEST_LOG(": ");
|
|
goto SHOW_MENU;
|
|
break;
|
|
}
|
|
|
|
WebRtc_Word32 ret = funcMgr.DoTest(testType);
|
|
|
|
if (sel > 0)
|
|
{
|
|
quit = true;
|
|
}
|
|
}
|
|
|
|
funcMgr.Close();
|
|
|
|
return 0;
|
|
}
|