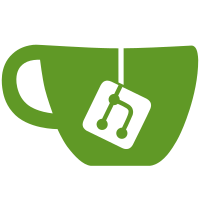
BUG= Review URL: https://webrtc-codereview.appspot.com/1166004 git-svn-id: http://webrtc.googlecode.com/svn/trunk@3627 4adac7df-926f-26a2-2b94-8c16560cd09d
42 lines
1.6 KiB
Python
42 lines
1.6 KiB
Python
#!/usr/bin/env python
|
|
#-*- coding: utf-8 -*-
|
|
# Copyright (c) 2012 The WebRTC project authors. All Rights Reserved.
|
|
#
|
|
# Use of this source code is governed by a BSD-style license
|
|
# that can be found in the LICENSE file in the root of the source
|
|
# tree. An additional intellectual property rights grant can be found
|
|
# in the file PATENTS. All contributing project authors may
|
|
# be found in the AUTHORS file in the root of the source tree.
|
|
|
|
"""Loads coverage data from the database."""
|
|
|
|
from google.appengine.ext import db
|
|
import gviz_api
|
|
|
|
|
|
class CoverageDataLoader:
|
|
""" Loads coverage data from the database."""
|
|
@staticmethod
|
|
def load_coverage_json_data(report_category):
|
|
coverage_entries = db.GqlQuery('SELECT * '
|
|
'FROM CoverageData '
|
|
'WHERE report_category = :1 '
|
|
'ORDER BY date ASC', report_category)
|
|
data = []
|
|
for coverage_entry in coverage_entries:
|
|
# Note: The date column must be first in alphabetical order since it is
|
|
# the primary column. This is a bug in the gviz api (or at least it
|
|
# doesn't make much sense).
|
|
data.append({'aa_date': coverage_entry.date,
|
|
'line_coverage': coverage_entry.line_coverage,
|
|
'function_coverage': coverage_entry.function_coverage,
|
|
})
|
|
|
|
description = {
|
|
'aa_date': ('datetime', 'Date'),
|
|
'line_coverage': ('number', 'Line Coverage'),
|
|
'function_coverage': ('number', 'Function Coverage'),
|
|
}
|
|
coverage_data = gviz_api.DataTable(description, data)
|
|
return coverage_data.ToJSon(order_by='date')
|