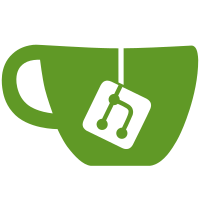
Implements the ExternalVideoDecoder interface for VideoReceiveStream. Also adds a FakeDecoder used in tests, removing the overhead of running the EngineTest tests with VP8 under Memcheck/TSan, allowing us to enable them under Memcheck/TSan as well. BUG=2346,2312 R=stefan@webrtc.org Review URL: https://webrtc-codereview.appspot.com/2172004 git-svn-id: http://webrtc.googlecode.com/svn/trunk@4702 4adac7df-926f-26a2-2b94-8c16560cd09d
85 lines
2.6 KiB
C++
85 lines
2.6 KiB
C++
/*
|
|
* Copyright (c) 2013 The WebRTC project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef WEBRTC_VIDEO_ENGINE_VIDEO_RECEIVE_STREAM_IMPL_H_
|
|
#define WEBRTC_VIDEO_ENGINE_VIDEO_RECEIVE_STREAM_IMPL_H_
|
|
|
|
#include <vector>
|
|
|
|
#include "webrtc/common_video/libyuv/include/webrtc_libyuv.h"
|
|
#include "webrtc/system_wrappers/interface/clock.h"
|
|
#include "webrtc/video_engine/include/vie_render.h"
|
|
#include "webrtc/video_engine/new_include/video_receive_stream.h"
|
|
|
|
namespace webrtc {
|
|
|
|
class VideoEngine;
|
|
class ViEBase;
|
|
class ViECodec;
|
|
class ViEExternalCodec;
|
|
class ViENetwork;
|
|
class ViERender;
|
|
class ViERTP_RTCP;
|
|
|
|
namespace internal {
|
|
|
|
class VideoReceiveStream : public webrtc::VideoReceiveStream,
|
|
public webrtc::ExternalRenderer,
|
|
public webrtc::Transport {
|
|
public:
|
|
VideoReceiveStream(webrtc::VideoEngine* video_engine,
|
|
const VideoReceiveStream::Config& config,
|
|
newapi::Transport* transport);
|
|
virtual ~VideoReceiveStream();
|
|
|
|
virtual void StartReceive() OVERRIDE;
|
|
virtual void StopReceive() OVERRIDE;
|
|
|
|
virtual void GetCurrentReceiveCodec(VideoCodec* receive_codec) OVERRIDE;
|
|
|
|
virtual int FrameSizeChange(unsigned int width, unsigned int height,
|
|
unsigned int /*number_of_streams*/) OVERRIDE;
|
|
virtual int DeliverFrame(uint8_t* frame, int buffer_size, uint32_t timestamp,
|
|
int64_t render_time, void* /*handle*/) OVERRIDE;
|
|
|
|
virtual bool IsTextureSupported() OVERRIDE;
|
|
|
|
virtual int SendPacket(int /*channel*/, const void* packet, int length)
|
|
OVERRIDE;
|
|
virtual int SendRTCPPacket(int /*channel*/, const void* packet, int length)
|
|
OVERRIDE;
|
|
|
|
public:
|
|
virtual bool DeliverRtcp(const uint8_t* packet, size_t length);
|
|
virtual bool DeliverRtp(const uint8_t* packet, size_t length);
|
|
|
|
private:
|
|
newapi::Transport* transport_;
|
|
VideoReceiveStream::Config config_;
|
|
Clock* clock_;
|
|
|
|
ViEBase* video_engine_base_;
|
|
ViECodec* codec_;
|
|
ViEExternalCodec* external_codec_;
|
|
ViENetwork* network_;
|
|
ViERender* render_;
|
|
ViERTP_RTCP* rtp_rtcp_;
|
|
|
|
int channel_;
|
|
|
|
// TODO(pbos): Remove VideoReceiveStream can operate on I420 frames directly.
|
|
unsigned int height_;
|
|
unsigned int width_;
|
|
};
|
|
} // internal
|
|
} // webrtc
|
|
|
|
#endif // WEBRTC_VIDEO_ENGINE_INTERNAL_VIDEO_RECEIVE_STREAM_H_
|