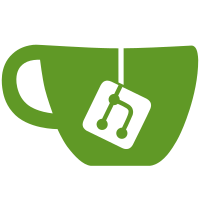
This commit added a 3 bit index to the bitstream, the index is used to look into the intra mode coding entropy context table. The commit uses the mode stats to calculate the cost of transmitting modes using 8 possible entropy distributions, and selects the distribution that provides the lowest cost to do the actual mode coding. Initial test show this provides additional .2%~.3% gain over quantizer adaptive intra mode coding. So the adaptive intra mode coding provides a total of .5%(psnr) to .6% gain(ssim) combined for all-key-encoding To build and test, configure with --enable-experimental --enable-qimode Change-Id: I7c41cd8bfb352bc1fe7c5da1848a58faea5ed74a
58 lines
1.7 KiB
C
58 lines
1.7 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
|
|
#include "vp8/common/blockd.h"
|
|
#include "onyx_int.h"
|
|
#include "treewriter.h"
|
|
#include "vp8/common/entropymode.h"
|
|
|
|
|
|
void vp8_init_mode_costs(VP8_COMP *c)
|
|
{
|
|
VP8_COMMON *x = &c->common;
|
|
{
|
|
const vp8_tree_p T = vp8_bmode_tree;
|
|
|
|
int i = 0;
|
|
|
|
do
|
|
{
|
|
int j = 0;
|
|
|
|
do
|
|
{
|
|
vp8_cost_tokens((int *)c->mb.bmode_costs[i][j], x->kf_bmode_prob[i][j], T);
|
|
}
|
|
while (++j < VP8_BINTRAMODES);
|
|
}
|
|
while (++i < VP8_BINTRAMODES);
|
|
|
|
vp8_cost_tokens((int *)c->mb.inter_bmode_costs, x->fc.bmode_prob, T);
|
|
}
|
|
vp8_cost_tokens((int *)c->mb.inter_bmode_costs, x->fc.sub_mv_ref_prob, vp8_sub_mv_ref_tree);
|
|
|
|
vp8_cost_tokens(c->mb.mbmode_cost[1], x->fc.ymode_prob, vp8_ymode_tree);
|
|
#if CONFIG_QIMODE
|
|
vp8_cost_tokens(c->mb.mbmode_cost[0],
|
|
x->kf_ymode_prob[c->common.kf_ymode_probs_index], vp8_kf_ymode_tree);
|
|
#else
|
|
vp8_cost_tokens(c->mb.mbmode_cost[0], x->kf_ymode_prob, vp8_kf_ymode_tree);
|
|
#endif
|
|
vp8_cost_tokens(c->mb.intra_uv_mode_cost[1], x->fc.uv_mode_prob, vp8_uv_mode_tree);
|
|
vp8_cost_tokens(c->mb.intra_uv_mode_cost[0], x->kf_uv_mode_prob, vp8_uv_mode_tree);
|
|
#if CONFIG_I8X8
|
|
vp8_cost_tokens(c->mb.i8x8_mode_costs,
|
|
x->i8x8_mode_prob,vp8_i8x8_mode_tree);
|
|
#endif
|
|
|
|
|
|
}
|