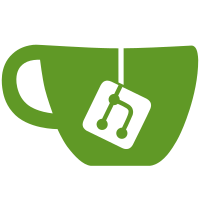
Adds following targets to configure script to support RVCT compilation without operating system support (for Profiler or bare metal images). - armv5te-none-rvct - armv6-none-rvct - armv7-none-rvct To strip OS specific parts from the code "os_support"-config was added to script and CONFIG_OS_SUPPORT flag is used in the code to exclude OS specific parts such as OS specific includes and function calls for timers and threads etc. This was done to enable RVCT compilation for profiling purposes or running the image on bare metal target with Lauterbach. Removed separate AREA directives for READONLY data in armv6 and neon assembly files to fix the RVCT compilation. Otherwise "ldr <reg>, =label" syntax would have been needed to prevent linker errors. This syntax is not supported by older gnu assemblers. Change-Id: I14f4c68529e8c27397502fbc3010a54e505ddb43
122 lines
2.4 KiB
C
122 lines
2.4 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
|
|
#ifndef VPX_TIMER_H
|
|
#define VPX_TIMER_H
|
|
|
|
#if CONFIG_OS_SUPPORT
|
|
|
|
#if defined(_WIN32)
|
|
/*
|
|
* Win32 specific includes
|
|
*/
|
|
#ifndef WIN32_LEAN_AND_MEAN
|
|
#define WIN32_LEAN_AND_MEAN
|
|
#endif
|
|
#include <windows.h>
|
|
#else
|
|
/*
|
|
* POSIX specific includes
|
|
*/
|
|
#include <sys/time.h>
|
|
|
|
/* timersub is not provided by msys at this time. */
|
|
#ifndef timersub
|
|
#define timersub(a, b, result) \
|
|
do { \
|
|
(result)->tv_sec = (a)->tv_sec - (b)->tv_sec; \
|
|
(result)->tv_usec = (a)->tv_usec - (b)->tv_usec; \
|
|
if ((result)->tv_usec < 0) { \
|
|
--(result)->tv_sec; \
|
|
(result)->tv_usec += 1000000; \
|
|
} \
|
|
} while (0)
|
|
#endif
|
|
#endif
|
|
|
|
|
|
struct vpx_usec_timer
|
|
{
|
|
#if defined(_WIN32)
|
|
LARGE_INTEGER begin, end;
|
|
#else
|
|
struct timeval begin, end;
|
|
#endif
|
|
};
|
|
|
|
|
|
static void
|
|
vpx_usec_timer_start(struct vpx_usec_timer *t)
|
|
{
|
|
#if defined(_WIN32)
|
|
QueryPerformanceCounter(&t->begin);
|
|
#else
|
|
gettimeofday(&t->begin, NULL);
|
|
#endif
|
|
}
|
|
|
|
|
|
static void
|
|
vpx_usec_timer_mark(struct vpx_usec_timer *t)
|
|
{
|
|
#if defined(_WIN32)
|
|
QueryPerformanceCounter(&t->end);
|
|
#else
|
|
gettimeofday(&t->end, NULL);
|
|
#endif
|
|
}
|
|
|
|
|
|
static long
|
|
vpx_usec_timer_elapsed(struct vpx_usec_timer *t)
|
|
{
|
|
#if defined(_WIN32)
|
|
LARGE_INTEGER freq, diff;
|
|
|
|
diff.QuadPart = t->end.QuadPart - t->begin.QuadPart;
|
|
|
|
if (QueryPerformanceFrequency(&freq) && diff.QuadPart < freq.QuadPart)
|
|
return (long)(diff.QuadPart * 1000000 / freq.QuadPart);
|
|
|
|
return 1000000;
|
|
#else
|
|
struct timeval diff;
|
|
|
|
timersub(&t->end, &t->begin, &diff);
|
|
return diff.tv_sec ? 1000000 : diff.tv_usec;
|
|
#endif
|
|
}
|
|
|
|
#else /* CONFIG_OS_SUPPORT = 0*/
|
|
|
|
/* Empty timer functions if CONFIG_OS_SUPPORT = 0 */
|
|
#ifndef timersub
|
|
#define timersub(a, b, result)
|
|
#endif
|
|
|
|
struct vpx_usec_timer
|
|
{
|
|
void *dummy;
|
|
};
|
|
|
|
static void
|
|
vpx_usec_timer_start(struct vpx_usec_timer *t) { }
|
|
|
|
static void
|
|
vpx_usec_timer_mark(struct vpx_usec_timer *t) { }
|
|
|
|
static long
|
|
vpx_usec_timer_elapsed(struct vpx_usec_timer *t) { return 0; }
|
|
|
|
#endif /* CONFIG_OS_SUPPORT */
|
|
|
|
#endif
|