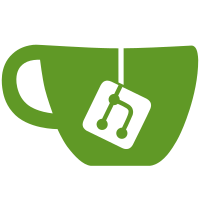
This commit added a set of loop filter functions for macroblocks using 8x8 transform. First we turned off the regular loop filtering on 4x4 block boundaries that do not exist in macroblocks using 8x8 transform. Second, we change to use the same loop filter(mask and 7 tap filter) that used for macroblock edge filtering. Change-Id: I3a00460b7674ced116917d86812ffc32578c1d3a
233 lines
5.8 KiB
C
233 lines
5.8 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
|
|
#include "vpx_ports/config.h"
|
|
#include "blockd.h"
|
|
#include "vpx_mem/vpx_mem.h"
|
|
#include "onyxc_int.h"
|
|
#include "findnearmv.h"
|
|
#include "entropymode.h"
|
|
#include "systemdependent.h"
|
|
|
|
|
|
extern void vp8_init_scan_order_mask();
|
|
|
|
static void update_mode_info_border(MODE_INFO *mi, int rows, int cols)
|
|
{
|
|
int i;
|
|
vpx_memset(mi - cols - 2, 0, sizeof(MODE_INFO) * (cols + 1));
|
|
|
|
for (i = 0; i < rows; i++)
|
|
{
|
|
/* TODO(holmer): Bug? This updates the last element of each row
|
|
* rather than the border element!
|
|
*/
|
|
vpx_memset(&mi[i*cols-1], 0, sizeof(MODE_INFO));
|
|
}
|
|
}
|
|
|
|
void vp8_de_alloc_frame_buffers(VP8_COMMON *oci)
|
|
{
|
|
int i;
|
|
|
|
for (i = 0; i < NUM_YV12_BUFFERS; i++)
|
|
vp8_yv12_de_alloc_frame_buffer(&oci->yv12_fb[i]);
|
|
|
|
vp8_yv12_de_alloc_frame_buffer(&oci->temp_scale_frame);
|
|
vp8_yv12_de_alloc_frame_buffer(&oci->post_proc_buffer);
|
|
|
|
vpx_free(oci->above_context);
|
|
vpx_free(oci->mip);
|
|
vpx_free(oci->prev_mip);
|
|
|
|
oci->above_context = 0;
|
|
oci->mip = 0;
|
|
oci->prev_mip = 0;
|
|
|
|
}
|
|
|
|
int vp8_alloc_frame_buffers(VP8_COMMON *oci, int width, int height)
|
|
{
|
|
int i;
|
|
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
|
|
/* our internal buffers are always multiples of 16 */
|
|
if ((width & 0xf) != 0)
|
|
width += 16 - (width & 0xf);
|
|
|
|
if ((height & 0xf) != 0)
|
|
height += 16 - (height & 0xf);
|
|
|
|
|
|
for (i = 0; i < NUM_YV12_BUFFERS; i++)
|
|
{
|
|
oci->fb_idx_ref_cnt[i] = 0;
|
|
oci->yv12_fb[i].flags = 0;
|
|
if (vp8_yv12_alloc_frame_buffer(&oci->yv12_fb[i], width, height, VP8BORDERINPIXELS) < 0)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
}
|
|
|
|
oci->new_fb_idx = 0;
|
|
oci->lst_fb_idx = 1;
|
|
oci->gld_fb_idx = 2;
|
|
oci->alt_fb_idx = 3;
|
|
|
|
oci->fb_idx_ref_cnt[0] = 1;
|
|
oci->fb_idx_ref_cnt[1] = 1;
|
|
oci->fb_idx_ref_cnt[2] = 1;
|
|
oci->fb_idx_ref_cnt[3] = 1;
|
|
|
|
if (vp8_yv12_alloc_frame_buffer(&oci->temp_scale_frame, width, 16, VP8BORDERINPIXELS) < 0)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
|
|
if (vp8_yv12_alloc_frame_buffer(&oci->post_proc_buffer, width, height, VP8BORDERINPIXELS) < 0)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
|
|
oci->mb_rows = height >> 4;
|
|
oci->mb_cols = width >> 4;
|
|
oci->MBs = oci->mb_rows * oci->mb_cols;
|
|
oci->mode_info_stride = oci->mb_cols + 1;
|
|
oci->mip = vpx_calloc((oci->mb_cols + 1) * (oci->mb_rows + 1), sizeof(MODE_INFO));
|
|
|
|
if (!oci->mip)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
|
|
oci->mi = oci->mip + oci->mode_info_stride + 1;
|
|
|
|
/* allocate memory for last frame MODE_INFO array */
|
|
#if CONFIG_ERROR_CONCEALMENT || CONFIG_NEWNEAR
|
|
oci->prev_mip = vpx_calloc((oci->mb_cols + 1) * (oci->mb_rows + 1), sizeof(MODE_INFO));
|
|
|
|
if (!oci->prev_mip)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
|
|
oci->prev_mi = oci->prev_mip + oci->mode_info_stride + 1;
|
|
#else
|
|
oci->prev_mip = NULL;
|
|
oci->prev_mi = NULL;
|
|
#endif
|
|
|
|
oci->above_context = vpx_calloc(sizeof(ENTROPY_CONTEXT_PLANES) * oci->mb_cols, 1);
|
|
|
|
if (!oci->above_context)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
return 1;
|
|
}
|
|
|
|
update_mode_info_border(oci->mi, oci->mb_rows, oci->mb_cols);
|
|
#if CONFIG_ERROR_CONCEALMENT
|
|
update_mode_info_border(oci->prev_mi, oci->mb_rows, oci->mb_cols);
|
|
#endif
|
|
|
|
return 0;
|
|
}
|
|
void vp8_setup_version(VP8_COMMON *cm)
|
|
{
|
|
if (cm->version & 0x4)
|
|
{
|
|
if (!CONFIG_EXPERIMENTAL)
|
|
vpx_internal_error(&cm->error, VPX_CODEC_UNSUP_BITSTREAM,
|
|
"Bitstream was created by an experimental "
|
|
"encoder");
|
|
cm->experimental = 1;
|
|
}
|
|
|
|
switch (cm->version & 0x3)
|
|
{
|
|
case 0:
|
|
cm->no_lpf = 0;
|
|
cm->filter_type = NORMAL_LOOPFILTER;
|
|
cm->use_bilinear_mc_filter = 0;
|
|
cm->full_pixel = 0;
|
|
break;
|
|
case 1:
|
|
cm->no_lpf = 0;
|
|
cm->filter_type = SIMPLE_LOOPFILTER;
|
|
cm->use_bilinear_mc_filter = 1;
|
|
cm->full_pixel = 0;
|
|
break;
|
|
case 2:
|
|
cm->no_lpf = 1;
|
|
cm->filter_type = NORMAL_LOOPFILTER;
|
|
cm->use_bilinear_mc_filter = 1;
|
|
cm->full_pixel = 0;
|
|
break;
|
|
case 3:
|
|
cm->no_lpf = 1;
|
|
cm->filter_type = SIMPLE_LOOPFILTER;
|
|
cm->use_bilinear_mc_filter = 1;
|
|
cm->full_pixel = 1;
|
|
break;
|
|
}
|
|
}
|
|
void vp8_create_common(VP8_COMMON *oci)
|
|
{
|
|
vp8_machine_specific_config(oci);
|
|
|
|
vp8_init_mbmode_probs(oci);
|
|
|
|
vp8_default_bmode_probs(oci->fc.bmode_prob);
|
|
|
|
oci->mb_no_coeff_skip = 1;
|
|
#if CONFIG_DUALPRED
|
|
oci->dual_pred_mode = HYBRID_PREDICTION;
|
|
#endif /* CONFIG_DUALPRED */
|
|
oci->no_lpf = 0;
|
|
oci->filter_type = NORMAL_LOOPFILTER;
|
|
oci->use_bilinear_mc_filter = 0;
|
|
oci->full_pixel = 0;
|
|
oci->multi_token_partition = ONE_PARTITION;
|
|
oci->clr_type = REG_YUV;
|
|
oci->clamp_type = RECON_CLAMP_REQUIRED;
|
|
|
|
/* Initialise reference frame sign bias structure to defaults */
|
|
vpx_memset(oci->ref_frame_sign_bias, 0, sizeof(oci->ref_frame_sign_bias));
|
|
|
|
/* Default disable buffer to buffer copying */
|
|
oci->copy_buffer_to_gf = 0;
|
|
oci->copy_buffer_to_arf = 0;
|
|
#if CONFIG_QIMODE
|
|
oci->kf_ymode_probs_update = 0;
|
|
#endif
|
|
}
|
|
|
|
void vp8_remove_common(VP8_COMMON *oci)
|
|
{
|
|
vp8_de_alloc_frame_buffers(oci);
|
|
}
|
|
|
|
void vp8_initialize_common()
|
|
{
|
|
vp8_coef_tree_initialize();
|
|
|
|
vp8_entropy_mode_init();
|
|
|
|
vp8_init_scan_order_mask();
|
|
|
|
}
|