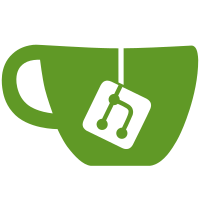
Produce the token partitions on-the-fly, while processing each MB. Context is updated at the beginning of each frame based on the previoud frame's counters. Optimally encoder outputs partitions in separate buffers. For frame based output, partitions are concatenated internally. Limitations: - enabled just in combination with realtime-only mode - number of encoding threads has to be equal or less than the number of token partitions. For this reason, by default the encoder will do 8 token partitions. - vpxenc supports partition output (-P) just in combination with IVF output format (--ivf) Performance: - Realtime encoder can be up to 13% faster (ARM) depending on the number of threads and bitrate settings. Constant gain over the 5-16 speed range. - Token buffer reduced from one frame to 8 MBs Quality: - quality is affected by the delayed context updates. This again dependents on input material, speed and bitrate settings. For VC style input the loss seen is up to 0.2dB. If error-resilient=2 mode is used than the effect of this change is negligible. Example: ./configure --enable-realtime-only --enable-onthefly-bitpacking ./vpxenc --rt --end-usage=1 --fps=30000/1000 -w 640 -h 480 --target-bitrate=1000 --token-parts=3 --static-thresh=2000 --ivf -P -t 4 -o strm.ivf tanya_640x480.yuv Change-Id: I127295cb85b835fc287e1c0201a67e378d025d76
47 lines
2.1 KiB
C
47 lines
2.1 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
|
|
#ifndef __INC_BITSTREAM_H
|
|
#define __INC_BITSTREAM_H
|
|
|
|
#if HAVE_EDSP
|
|
void vp8cx_pack_tokens_armv5(vp8_writer *w, const TOKENEXTRA *p, int xcount,
|
|
vp8_token *,
|
|
vp8_extra_bit_struct *,
|
|
const vp8_tree_index *);
|
|
void vp8cx_pack_tokens_into_partitions_armv5(VP8_COMP *,
|
|
unsigned char * cx_data,
|
|
const unsigned char *cx_data_end,
|
|
int num_parts,
|
|
vp8_token *,
|
|
vp8_extra_bit_struct *,
|
|
const vp8_tree_index *);
|
|
void vp8cx_pack_mb_row_tokens_armv5(VP8_COMP *cpi, vp8_writer *w,
|
|
vp8_token *,
|
|
vp8_extra_bit_struct *,
|
|
const vp8_tree_index *);
|
|
# define pack_tokens(a,b,c) \
|
|
vp8cx_pack_tokens_armv5(a,b,c,vp8_coef_encodings,vp8_extra_bits,vp8_coef_tree)
|
|
# define pack_tokens_into_partitions(a,b,c,d) \
|
|
vp8cx_pack_tokens_into_partitions_armv5(a,b,c,d,vp8_coef_encodings,vp8_extra_bits,vp8_coef_tree)
|
|
# define pack_mb_row_tokens(a,b) \
|
|
vp8cx_pack_mb_row_tokens_armv5(a,b,vp8_coef_encodings,vp8_extra_bits,vp8_coef_tree)
|
|
#else
|
|
|
|
void vp8_pack_tokens_c(vp8_writer *w, const TOKENEXTRA *p, int xcount);
|
|
|
|
# define pack_tokens(a,b,c) vp8_pack_tokens_c(a,b,c)
|
|
# define pack_tokens_into_partitions(a,b,c,d) pack_tokens_into_partitions_c(a,b,c,d)
|
|
# define pack_mb_row_tokens(a,b) pack_mb_row_tokens_c(a,b)
|
|
#endif
|
|
|
|
#endif
|