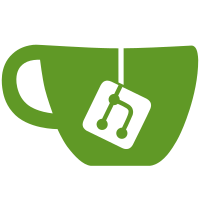
Implements some of the helper functions more efficiently with lookups rathers than branches. Modeling function is consolidated to reduce some computations. Also merged the two enums BLOCK_SIZE_TYPES and BlockSize into one because there is no need to keep them separate (even though the semantics are a little different). No bitstream or output change. About 0.5% speedup Change-Id: I7d71a66e8031ddb340744dc493f22976052b8f9f
68 lines
2.5 KiB
C
68 lines
2.5 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
#ifndef VP9_COMMON_VP9_ENUMS_H_
|
|
#define VP9_COMMON_VP9_ENUMS_H_
|
|
|
|
#include "./vpx_config.h"
|
|
|
|
#define LOG2_MI_SIZE 3
|
|
#define LOG2_MI_BLOCK_SIZE (6 - LOG2_MI_SIZE) // 64 = 2^6
|
|
|
|
#define MI_SIZE (1 << LOG2_MI_SIZE) // pixels per mi-unit
|
|
#define MI_BLOCK_SIZE (1 << LOG2_MI_BLOCK_SIZE) // mi-units per max block
|
|
|
|
#define MI_MASK (MI_BLOCK_SIZE - 1)
|
|
|
|
typedef enum BLOCK_SIZE_TYPE {
|
|
BLOCK_SIZE_AB4X4, BLOCK_4X4 = BLOCK_SIZE_AB4X4,
|
|
BLOCK_SIZE_SB4X8, BLOCK_4X8 = BLOCK_SIZE_SB4X8,
|
|
BLOCK_SIZE_SB8X4, BLOCK_8X4 = BLOCK_SIZE_SB8X4,
|
|
BLOCK_SIZE_SB8X8, BLOCK_8X8 = BLOCK_SIZE_SB8X8,
|
|
BLOCK_SIZE_SB8X16, BLOCK_8X16 = BLOCK_SIZE_SB8X16,
|
|
BLOCK_SIZE_SB16X8, BLOCK_16X8 = BLOCK_SIZE_SB16X8,
|
|
BLOCK_SIZE_MB16X16, BLOCK_16X16 = BLOCK_SIZE_MB16X16,
|
|
BLOCK_SIZE_SB16X32, BLOCK_16X32 = BLOCK_SIZE_SB16X32,
|
|
BLOCK_SIZE_SB32X16, BLOCK_32X16 = BLOCK_SIZE_SB32X16,
|
|
BLOCK_SIZE_SB32X32, BLOCK_32X32 = BLOCK_SIZE_SB32X32,
|
|
BLOCK_SIZE_SB32X64, BLOCK_32X64 = BLOCK_SIZE_SB32X64,
|
|
BLOCK_SIZE_SB64X32, BLOCK_64X32 = BLOCK_SIZE_SB64X32,
|
|
BLOCK_SIZE_SB64X64, BLOCK_64X64 = BLOCK_SIZE_SB64X64,
|
|
BLOCK_SIZE_TYPES, BLOCK_MAX_SB_SEGMENTS = BLOCK_SIZE_TYPES,
|
|
} BLOCK_SIZE_TYPE;
|
|
|
|
typedef enum PARTITION_TYPE {
|
|
PARTITION_NONE,
|
|
PARTITION_HORZ,
|
|
PARTITION_VERT,
|
|
PARTITION_SPLIT,
|
|
PARTITION_TYPES
|
|
} PARTITION_TYPE;
|
|
|
|
#define PARTITION_PLOFFSET 4 // number of probability models per block size
|
|
#define NUM_PARTITION_CONTEXTS (4 * PARTITION_PLOFFSET)
|
|
|
|
typedef enum {
|
|
TX_4X4 = 0, // 4x4 dct transform
|
|
TX_8X8 = 1, // 8x8 dct transform
|
|
TX_16X16 = 2, // 16x16 dct transform
|
|
TX_32X32 = 3, // 32x32 dct transform
|
|
TX_SIZE_MAX_SB, // Number of transforms available to SBs
|
|
} TX_SIZE;
|
|
|
|
typedef enum {
|
|
DCT_DCT = 0, // DCT in both horizontal and vertical
|
|
ADST_DCT = 1, // ADST in vertical, DCT in horizontal
|
|
DCT_ADST = 2, // DCT in vertical, ADST in horizontal
|
|
ADST_ADST = 3 // ADST in both directions
|
|
} TX_TYPE;
|
|
|
|
#endif // VP9_COMMON_VP9_ENUMS_H_
|