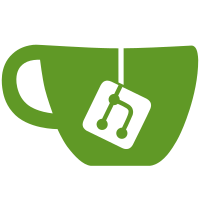
Add a new encoder control, VP8E_SET_TUNING, to allow the application to inform the encoder that the material will benefit from certain tuning. Expose this control as the --tune option to vpxenc. The args helper is expanded to support enumerated arguments by name or value. Two tunings are provided by this patch, PSNR (default) and SSIM. Activity masking is made dependent on setting --tune=ssim, as the current implementation hurts speed (10%) and PSNR (2.7% avg, 10% peak) too much for it to be a default yet. Change-Id: I110d969381c4805347ff5a0ffaf1a14ca1965257
55 lines
1.5 KiB
C
55 lines
1.5 KiB
C
/*
|
|
* Copyright (c) 2010 The WebM project authors. All Rights Reserved.
|
|
*
|
|
* Use of this source code is governed by a BSD-style license
|
|
* that can be found in the LICENSE file in the root of the source
|
|
* tree. An additional intellectual property rights grant can be found
|
|
* in the file PATENTS. All contributing project authors may
|
|
* be found in the AUTHORS file in the root of the source tree.
|
|
*/
|
|
|
|
|
|
#ifndef ARGS_H
|
|
#define ARGS_H
|
|
#include <stdio.h>
|
|
|
|
struct arg
|
|
{
|
|
char **argv;
|
|
const char *name;
|
|
const char *val;
|
|
unsigned int argv_step;
|
|
const struct arg_def *def;
|
|
};
|
|
|
|
struct arg_enum_list
|
|
{
|
|
const char *name;
|
|
int val;
|
|
};
|
|
#define ARG_ENUM_LIST_END {0}
|
|
|
|
typedef struct arg_def
|
|
{
|
|
const char *short_name;
|
|
const char *long_name;
|
|
int has_val;
|
|
const char *desc;
|
|
const struct arg_enum_list *enums;
|
|
} arg_def_t;
|
|
#define ARG_DEF(s,l,v,d) {s,l,v,d, NULL}
|
|
#define ARG_DEF_ENUM(s,l,v,d,e) {s,l,v,d,e}
|
|
#define ARG_DEF_LIST_END {0}
|
|
|
|
struct arg arg_init(char **argv);
|
|
int arg_match(struct arg *arg_, const struct arg_def *def, char **argv);
|
|
const char *arg_next(struct arg *arg);
|
|
void arg_show_usage(FILE *fp, const struct arg_def *const *defs);
|
|
char **argv_dup(int argc, const char **argv);
|
|
|
|
unsigned int arg_parse_uint(const struct arg *arg);
|
|
int arg_parse_int(const struct arg *arg);
|
|
struct vpx_rational arg_parse_rational(const struct arg *arg);
|
|
int arg_parse_enum_or_int(const struct arg *arg);
|
|
#endif
|