mirror of
https://github.com/pocoproject/poco.git
synced 2025-04-02 17:50:53 +02:00
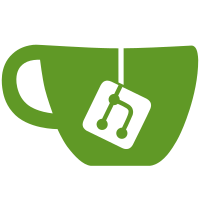
* feat(Context): DH init openssl3 port (1/2 hardcoded params) * create poco-1.11.3 branch, bump version * update copyright date * #3567: check legacy provider existence for legacy exception #3567 * fix(Placeholder): comparison for zero value * feat(Context): DH init openssl3 port (2/2 params from file) * test(HTTPSClientSession): try/catch to understand CI failure * chore(cmake): copy the DH parameters file * fix(OpenSSLInitializer): unload provider on uninitialize * chore(HTTPSClientSessionTest): remove try/catch * fix(OpenSSLInitializer): fix provider unloading * feat(CppUnit): make tests exceptions more descriptive * chore(CppUnit): a more descriptive name for callback Co-authored-by: Günter Obiltschnig <guenter.obiltschnig@appinf.com>
68 lines
1.3 KiB
C++
68 lines
1.3 KiB
C++
//
|
|
// Utility.cpp
|
|
//
|
|
// Library: NetSSL_OpenSSL
|
|
// Package: SSLCore
|
|
// Module: Utility
|
|
//
|
|
// Copyright (c) 2006-2009, Applied Informatics Software Engineering GmbH.
|
|
// and Contributors.
|
|
//
|
|
// SPDX-License-Identifier: BSL-1.0
|
|
//
|
|
|
|
|
|
#include "Poco/Net/Utility.h"
|
|
#include "Poco/String.h"
|
|
#include "Poco/Util/OptionException.h"
|
|
#include "Poco/Crypto/Crypto.h"
|
|
#include <openssl/err.h>
|
|
|
|
|
|
namespace Poco {
|
|
namespace Net {
|
|
|
|
|
|
Context::VerificationMode Utility::convertVerificationMode(const std::string& vMode)
|
|
{
|
|
std::string mode = Poco::toLower(vMode);
|
|
Context::VerificationMode verMode = Context::VERIFY_STRICT;
|
|
|
|
if (mode == "none")
|
|
verMode = Context::VERIFY_NONE;
|
|
else if (mode == "relaxed")
|
|
verMode = Context::VERIFY_RELAXED;
|
|
else if (mode == "strict")
|
|
verMode = Context::VERIFY_STRICT;
|
|
else if (mode == "once")
|
|
verMode = Context::VERIFY_ONCE;
|
|
else
|
|
throw Poco::InvalidArgumentException("Invalid verification mode. Should be relaxed, strict or once but got", vMode);
|
|
|
|
return verMode;
|
|
}
|
|
|
|
|
|
std::string Utility::convertCertificateError(long errCode)
|
|
{
|
|
std::string errMsg(X509_verify_cert_error_string(errCode));
|
|
return errMsg;
|
|
}
|
|
|
|
|
|
std::string Utility::getLastError()
|
|
{
|
|
std::string msg;
|
|
Poco::Crypto::getError(msg);
|
|
return msg;
|
|
}
|
|
|
|
|
|
void Utility::clearErrorStack()
|
|
{
|
|
ERR_clear_error();
|
|
}
|
|
|
|
|
|
} } // namespace Poco::Net
|