mirror of
https://github.com/pocoproject/poco.git
synced 2024-12-12 18:20:26 +01:00
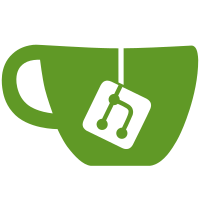
* enh(poco): Replace deprecated comments with C++ deprecated attribute. * enh(Poco): Replace some deprecated functionality in Poco sources. (#4426) * enh(Poco): Replace more deprecated functionality in Poco sources. (#4426) * fix(CMake): Variable BUILD_SHARED_LIBS must be defined properly to create valid binaries. * enh: Code improvements done while resolving deprecated functionality (#4426) * Un-deprecate LocalDateTme (#4426) * enh(Poco): Replace usage of deprecated functionality with other functions/classes (#4426) * chore(SSL): temporarily un-deprecate SSL-related functionality (#4426) * chore(SSL): temporarily un-deprecate old MongoDB protocol functionality (#4426) * enh(Poco): Minor Hash improvements (#4426) * enh(Foundation): Compile deprecated hash tests only when POCO_TEST_DEPRECATED is enabled (#4426) * enh(Net): Compile deprecated Socket::select functionality only when POCO_TEST_DEPRECATED is enabled (#4426) * enh(Bonjour): Replace deprecated Socket::select with PollSet (#4426) * enh(Poco): Introduce POCO_DEPRECATED macro to have the ability to disable deprecation warnings in applications (#4426) * test(ODBC): add few asserts to testStoredProcedureDynamicVar * fix(ODBC): rename DynamicAny -> DynamicVar in tests * fix(ODBC): make Dignostics static members inline to prevent explicit instantiation warnings on windows --------- Co-authored-by: Alex Fabijanic <alex@pocoproject.org>
159 lines
3.7 KiB
C++
Executable File
159 lines
3.7 KiB
C++
Executable File
//
|
|
// NObserver.h
|
|
//
|
|
// Library: Foundation
|
|
// Package: Notifications
|
|
// Module: NObserver
|
|
//
|
|
// Definition of the NObserver class template.
|
|
//
|
|
// Copyright (c) 2006, Applied Informatics Software Engineering GmbH.
|
|
// and Contributors.
|
|
//
|
|
// SPDX-License-Identifier: BSL-1.0
|
|
//
|
|
|
|
|
|
#ifndef Foundation_NObserver_INCLUDED
|
|
#define Foundation_NObserver_INCLUDED
|
|
|
|
|
|
#include "Poco/Foundation.h"
|
|
#include "Poco/AbstractObserver.h"
|
|
#include "Poco/Mutex.h"
|
|
|
|
|
|
namespace Poco {
|
|
|
|
|
|
template <class C, class N>
|
|
class NObserver: public AbstractObserver
|
|
/// This template class implements an adapter that sits between
|
|
/// a NotificationCenter and an object receiving notifications
|
|
/// from it. It is quite similar in concept to the
|
|
/// RunnableAdapter, but provides some NotificationCenter
|
|
/// specific additional methods.
|
|
/// See the NotificationCenter class for information on how
|
|
/// to use this template class.
|
|
///
|
|
/// This class template is quite similar to the Observer class
|
|
/// template. The differences are:
|
|
///
|
|
/// - NObserver expects the callback function to accept a const AutoPtr&
|
|
/// instead of a plain pointer as argument, thus simplifying memory
|
|
/// management.
|
|
///
|
|
/// - In addition to dispatching notifications based on the Notification runtime
|
|
/// type, NObserver can also notify subscribers based on the Notification name.
|
|
/// To enable this functionality, a matcher function must be provided.
|
|
/// Null matcher means no matching is performed and all notificiations
|
|
/// of the type subscribed to are dispatched.
|
|
{
|
|
public:
|
|
using Type = NObserver<C, N>;
|
|
using NotificationPtr = AutoPtr<N>;
|
|
using Callback = void (C::*)(const NotificationPtr&);
|
|
using Handler = Callback;
|
|
using Matcher = bool (C::*)(const std::string&) const;
|
|
|
|
NObserver() = delete;
|
|
|
|
NObserver(C& object, Handler method, Matcher matcher = nullptr):
|
|
_pObject(&object),
|
|
_handler(method),
|
|
_matcher(matcher)
|
|
{
|
|
}
|
|
|
|
NObserver(const NObserver& observer):
|
|
AbstractObserver(observer),
|
|
_pObject(observer._pObject),
|
|
_handler(observer._handler),
|
|
_matcher(observer._matcher)
|
|
{
|
|
}
|
|
|
|
~NObserver()
|
|
{
|
|
}
|
|
|
|
NObserver& operator = (const NObserver& observer)
|
|
{
|
|
if (&observer != this)
|
|
{
|
|
_pObject = observer._pObject;
|
|
_handler = observer._handler;
|
|
_matcher = observer._matcher;
|
|
}
|
|
return *this;
|
|
}
|
|
|
|
virtual void notify(Notification* pNf) const
|
|
{
|
|
handle(NotificationPtr(static_cast<N*>(pNf), true));
|
|
}
|
|
|
|
virtual bool equals(const AbstractObserver& abstractObserver) const
|
|
{
|
|
const NObserver* pObs = dynamic_cast<const NObserver*>(&abstractObserver);
|
|
return pObs && pObs->_pObject == _pObject && pObs->_handler == _handler && pObs->_matcher == _matcher;
|
|
}
|
|
|
|
POCO_DEPRECATED("use `bool accepts(const Notification::Ptr&)` instead")
|
|
virtual bool accepts(Notification* pNf, const char* pName) const
|
|
{
|
|
return (!pName || pNf->name() == pName) && dynamic_cast<N*>(pNf) != nullptr;
|
|
}
|
|
|
|
virtual bool accepts(const Notification::Ptr& pNf) const
|
|
{
|
|
return (match(pNf) && (pNf.template cast<N>() != nullptr));
|
|
}
|
|
|
|
virtual AbstractObserver* clone() const
|
|
{
|
|
return new NObserver(*this);
|
|
}
|
|
|
|
virtual void disable()
|
|
{
|
|
Poco::Mutex::ScopedLock lock(_mutex);
|
|
|
|
_pObject = nullptr;
|
|
}
|
|
|
|
protected:
|
|
|
|
void handle(const NotificationPtr& ptr) const
|
|
{
|
|
Mutex::ScopedLock lock(_mutex);
|
|
|
|
if (_pObject)
|
|
(_pObject->*_handler)(ptr);
|
|
}
|
|
|
|
bool match(const Notification::Ptr& ptr) const
|
|
{
|
|
Mutex::ScopedLock l(_mutex);
|
|
|
|
return _pObject && (!_matcher || (_pObject->*_matcher)(ptr->name()));
|
|
}
|
|
|
|
Mutex& mutex() const
|
|
{
|
|
return _mutex;
|
|
}
|
|
|
|
private:
|
|
C* _pObject;
|
|
Callback _handler;
|
|
Matcher _matcher;
|
|
mutable Poco::Mutex _mutex;
|
|
};
|
|
|
|
|
|
} // namespace Poco
|
|
|
|
|
|
#endif // Foundation_NObserver_INCLUDED
|