mirror of
https://github.com/msgpack/msgpack-c.git
synced 2025-07-01 00:13:30 +02:00
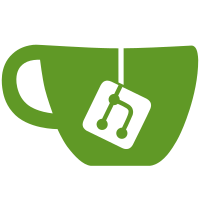
git-svn-id: file:///Users/frsyuki/project/msgpack-git/svn/x@67 5a5092ae-2292-43ba-b2d5-dcab9c1a2731
60 lines
1.5 KiB
C++
60 lines
1.5 KiB
C++
//
|
|
// MessagePack for C++ static resolution routine
|
|
//
|
|
// Copyright (C) 2008 FURUHASHI Sadayuki
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
//
|
|
#ifndef MSGPACK_TYPE_ARRAY_HPP__
|
|
#define MSGPACK_TYPE_ARRAY_HPP__
|
|
|
|
#include "msgpack/object.hpp"
|
|
#include <vector>
|
|
|
|
namespace msgpack {
|
|
namespace type {
|
|
|
|
|
|
template <typename T>
|
|
inline std::vector<T> operator<< (std::vector<T>& v, object o)
|
|
{
|
|
if(o.type != ARRAY) { throw type_error(); }
|
|
v.resize(o.via.container.size);
|
|
object* p(o.via.container.ptr);
|
|
object* const pend(o.via.container.ptr + o.via.container.size);
|
|
T* it(&v.front());
|
|
for(; p < pend; ++p, ++it) {
|
|
convert(*it, *p);
|
|
}
|
|
return v;
|
|
}
|
|
|
|
|
|
template <typename Stream, typename T>
|
|
inline const std::vector<T>& operator>> (const std::vector<T>& v, packer<Stream>& o)
|
|
{
|
|
o.pack_array(v.size());
|
|
for(typename std::vector<T>::const_iterator it(v.begin()), it_end(v.end());
|
|
it != it_end; ++it) {
|
|
pack(*it, o);
|
|
}
|
|
return v;
|
|
}
|
|
|
|
|
|
} // namespace type
|
|
} // namespace msgpack
|
|
|
|
#endif /* msgpack/type/array.hpp */
|
|
|