mirror of
https://github.com/msgpack/msgpack-c.git
synced 2025-07-01 00:13:30 +02:00
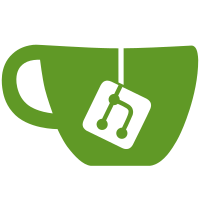
Removed obsolete unpack functions. Updated examples that no longer use obsolete functions. Added reference checking function to unpacked. ( unpacked::referenced() ) Added std:: namespace. Added reference or copy choice function and default behavior: When you use unpacker, default behavior is: STR, BIN, EXT types are always held by reference. When you don't use unpacker, default behavior is: STR, BIN, EXT types are always held by copy. The memory is allocated from zone. You can customize the behavior passing your custom judging function to unpack() or unpacker's constructor.
39 lines
1.0 KiB
C++
39 lines
1.0 KiB
C++
#include <msgpack.hpp>
|
|
#include <string>
|
|
#include <iostream>
|
|
#include <sstream>
|
|
|
|
int main(void)
|
|
{
|
|
msgpack::type::tuple<int, bool, std::string> src(1, true, "example");
|
|
|
|
// serialize the object into the buffer.
|
|
// any classes that implements write(const char*,size_t) can be a buffer.
|
|
std::stringstream buffer;
|
|
msgpack::pack(buffer, src);
|
|
|
|
// send the buffer ...
|
|
buffer.seekg(0);
|
|
|
|
// deserialize the buffer into msgpack::object instance.
|
|
std::string str(buffer.str());
|
|
|
|
msgpack::unpacked result;
|
|
|
|
msgpack::unpack(result, str.data(), str.size());
|
|
|
|
// deserialized object is valid during the msgpack::unpacked instance alive.
|
|
msgpack::object deserialized = result.get();
|
|
|
|
// msgpack::object supports ostream.
|
|
std::cout << deserialized << std::endl;
|
|
|
|
// convert msgpack::object instance into the original type.
|
|
// if the type is mismatched, it throws msgpack::type_error exception.
|
|
msgpack::type::tuple<int, bool, std::string> dst;
|
|
deserialized.convert(&dst);
|
|
|
|
return 0;
|
|
}
|
|
|