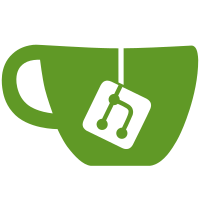
2 - Backport of svn revision 527: * Added API to ithread, created the following functions: - int ithread_initialize_library(void); - int ithread_cleanup_library(void); - int ithread_initialize_thread(void); - int ithread_cleanup_thread(void); * SF Bug Tracker [ 2876374 ] Access Violation when compiling with Visual Studio 2008 Submitted: Stulle ( stulleamgym ) - 2009-10-10 19:05 Hi, I am one of the devs of the MorphXT project and I use this lib in some other of my projects, too. When I tried to upgrade the lib earlier for one of my projects I had to realise that something did not work at first and while most of the things were reasonably ease to be fixed. Now, the last thing I encountered was not so easy to fix and I am uncertain if my fix is any good so I'll just post it here and wait for some comments. The problem was that I got an Access Violation when calling "UpnpInit". It would call "ithread_rwlock_init(&GlobalHndRWLock, NULL)" which eventually led to calling "pthread_cond_init" and I got the error notice at "EnterCriticalSection (&ptw32_cond_list_lock);". It appeared that "ptw32_cond_list_lock" was NULL. Now, I found two ways to fix this. Firstly moving the whole block after at least one of the "ThreadPoolInit" calls will fix the issue. Secondly, you could add: #ifdef WIN32 #ifdef PTW32_STATIC_LIB // to get the following working we need this... is it a good patch or not... I do not know! pthread_win32_process_attach_np(); #endif #endif right before "ithread_rwlock_init(&GlobalHndRWLock, NULL)". Just so you know, I am using libupnp 1.6.6 and libpthreads 2.8.0 and both are linked static into the binaries. I am currently using Visual Studio 2008 for development with Windows being the target OS. Any comment at your end? Regards, Stulle git-svn-id: https://pupnp.svn.sourceforge.net/svnroot/pupnp/branches/branch-1.6.x@529 119443c7-1b9e-41f8-b6fc-b9c35fce742c
338 lines
8.2 KiB
C
338 lines
8.2 KiB
C
/*******************************************************************************
|
|
*
|
|
* Copyright (c) 2000-2003 Intel Corporation
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* * Redistributions of source code must retain the above copyright notice,
|
|
* this list of conditions and the following disclaimer.
|
|
* * Redistributions in binary form must reproduce the above copyright notice,
|
|
* this list of conditions and the following disclaimer in the documentation
|
|
* and/or other materials provided with the distribution.
|
|
* * Neither name of Intel Corporation nor the names of its contributors
|
|
* may be used to endorse or promote products derived from this software
|
|
* without specific prior written permission.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
|
|
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
|
|
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
|
|
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL INTEL OR
|
|
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
|
|
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
|
|
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
|
|
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
|
|
* OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
|
|
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
|
|
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
*
|
|
******************************************************************************/
|
|
|
|
|
|
#ifndef UPNPAPI_H
|
|
#define UPNPAPI_H
|
|
|
|
|
|
/*!
|
|
* \file
|
|
*/
|
|
|
|
|
|
#include "client_table.h"
|
|
#include "upnp.h"
|
|
#include "VirtualDir.h" /* for struct VirtualDirCallbacks */
|
|
|
|
|
|
#define MAX_INTERFACES 256
|
|
|
|
#define DEFAULT_INTERFACE 1
|
|
|
|
#define DEV_LIMIT 200
|
|
|
|
#define NUM_HANDLE 200
|
|
|
|
#define DEFAULT_MX 5
|
|
|
|
#define DEFAULT_MAXAGE 1800
|
|
|
|
#define DEFAULT_SOAP_CONTENT_LENGTH 16000
|
|
#define MAX_SOAP_CONTENT_LENGTH 32000
|
|
|
|
extern size_t g_maxContentLength;
|
|
|
|
/* 30-second timeout */
|
|
#define UPNP_TIMEOUT 30
|
|
|
|
typedef enum {HND_INVALID=-1,HND_CLIENT,HND_DEVICE} Upnp_Handle_Type;
|
|
|
|
/* Data to be stored in handle table for */
|
|
struct Handle_Info
|
|
{
|
|
/*! . */
|
|
Upnp_Handle_Type HType;
|
|
/*! Callback function pointer. */
|
|
Upnp_FunPtr Callback;
|
|
/*! . */
|
|
char *Cookie;
|
|
/*! 0 = not installed; otherwise installed. */
|
|
int aliasInstalled;
|
|
|
|
/* Device Only */
|
|
#ifdef INCLUDE_DEVICE_APIS
|
|
/*! URL for the use of SSDP. */
|
|
char DescURL[LINE_SIZE];
|
|
/*! XML file path for device description. */
|
|
char DescXML[LINE_SIZE];
|
|
/* Advertisement timeout */
|
|
int MaxAge;
|
|
/*! Description parsed in terms of DOM document. */
|
|
IXML_Document *DescDocument;
|
|
/*! List of devices in the description document. */
|
|
IXML_NodeList *DeviceList;
|
|
/*! List of services in the description document. */
|
|
IXML_NodeList *ServiceList;
|
|
/*! Table holding subscriptions and URL information. */
|
|
service_table ServiceTable;
|
|
/*! . */
|
|
int MaxSubscriptions;
|
|
/*! . */
|
|
int MaxSubscriptionTimeOut;
|
|
/*! Address family: AF_INET or AF_INET6. */
|
|
int DeviceAf;
|
|
#endif
|
|
|
|
/* Client only */
|
|
#ifdef INCLUDE_CLIENT_APIS
|
|
/*! Client subscription list. */
|
|
ClientSubscription *ClientSubList;
|
|
/*! Active SSDP searches. */
|
|
LinkedList SsdpSearchList;
|
|
#endif
|
|
};
|
|
|
|
|
|
extern ithread_rwlock_t GlobalHndRWLock;
|
|
|
|
|
|
/*!
|
|
* \brief Get handle information.
|
|
*
|
|
* \return HND_DEVICE, UPNP_E_INVALID_HANDLE
|
|
*/
|
|
Upnp_Handle_Type GetHandleInfo(
|
|
/*! handle pointer (key for the client handle structure). */
|
|
int Hnd,
|
|
/*! handle structure passed by this function. */
|
|
struct Handle_Info **HndInfo);
|
|
|
|
|
|
#define HandleLock() HandleWriteLock()
|
|
|
|
|
|
#define HandleWriteLock() \
|
|
UpnpPrintf(UPNP_INFO, API, __FILE__, __LINE__, "Trying a write lock"); \
|
|
ithread_rwlock_wrlock(&GlobalHndRWLock); \
|
|
UpnpPrintf(UPNP_INFO, API, __FILE__, __LINE__, "Write lock acquired");
|
|
|
|
|
|
#define HandleReadLock() \
|
|
UpnpPrintf(UPNP_INFO, API, __FILE__, __LINE__, "Trying a read lock"); \
|
|
ithread_rwlock_rdlock(&GlobalHndRWLock); \
|
|
UpnpPrintf(UPNP_INFO, API, __FILE__, __LINE__, "Read lock acquired");
|
|
|
|
|
|
#define HandleUnlock() \
|
|
UpnpPrintf(UPNP_INFO, API,__FILE__, __LINE__, "Trying Unlock"); \
|
|
ithread_rwlock_unlock(&GlobalHndRWLock); \
|
|
UpnpPrintf(UPNP_INFO, API, __FILE__, __LINE__, "Unlocked rwlock");
|
|
|
|
|
|
/*!
|
|
* \brief Get client handle info.
|
|
*
|
|
* \note The logic around the use of this function should be revised.
|
|
*
|
|
* \return HND_CLIENT, HND_INVALID
|
|
*/
|
|
Upnp_Handle_Type GetClientHandleInfo(
|
|
/*! [in] client handle pointer (key for the client handle structure). */
|
|
int *client_handle_out,
|
|
/*! [out] Client handle structure passed by this function. */
|
|
struct Handle_Info **HndInfo);
|
|
/*!
|
|
* \brief Retrieves the device handle and information of the first device of
|
|
* the address family spcified.
|
|
*
|
|
* \return HND_DEVICE or HND_INVALID
|
|
*/
|
|
Upnp_Handle_Type GetDeviceHandleInfo(
|
|
/*! [in] Address family. */
|
|
const int AddressFamily,
|
|
/*! [out] Device handle pointer. */
|
|
int *device_handle_out,
|
|
/*! [out] Device handle structure passed by this function. */
|
|
struct Handle_Info **HndInfo);
|
|
|
|
|
|
extern char gIF_NAME[LINE_SIZE];
|
|
/*! INET_ADDRSTRLEN. */
|
|
extern char gIF_IPV4[22];
|
|
/*! INET6_ADDRSTRLEN. */
|
|
extern char gIF_IPV6[65];
|
|
extern int gIF_INDEX;
|
|
|
|
|
|
extern unsigned short LOCAL_PORT_V4;
|
|
extern unsigned short LOCAL_PORT_V6;
|
|
|
|
|
|
/*! NLS uuid. */
|
|
extern Upnp_SID gUpnpSdkNLSuuid;
|
|
|
|
|
|
extern TimerThread gTimerThread;
|
|
extern ThreadPool gRecvThreadPool;
|
|
extern ThreadPool gSendThreadPool;
|
|
extern ThreadPool gMiniServerThreadPool;
|
|
|
|
|
|
typedef enum {
|
|
SUBSCRIBE,
|
|
UNSUBSCRIBE,
|
|
DK_NOTIFY,
|
|
QUERY,
|
|
ACTION,
|
|
STATUS,
|
|
DEVDESCRIPTION,
|
|
SERVDESCRIPTION,
|
|
MINI,
|
|
RENEW
|
|
} UpnpFunName;
|
|
|
|
|
|
struct UpnpNonblockParam
|
|
{
|
|
UpnpFunName FunName;
|
|
int Handle;
|
|
int TimeOut;
|
|
char VarName[NAME_SIZE];
|
|
char NewVal[NAME_SIZE];
|
|
char DevType[NAME_SIZE];
|
|
char DevId[NAME_SIZE];
|
|
char ServiceType[NAME_SIZE];
|
|
char ServiceVer[NAME_SIZE];
|
|
char Url[NAME_SIZE];
|
|
Upnp_SID SubsId;
|
|
char *Cookie;
|
|
Upnp_FunPtr Fun;
|
|
IXML_Document *Header;
|
|
IXML_Document *Act;
|
|
struct DevDesc *Devdesc;
|
|
};
|
|
|
|
|
|
extern virtualDirList *pVirtualDirList;
|
|
extern struct VirtualDirCallbacks virtualDirCallback;
|
|
|
|
|
|
typedef enum {
|
|
WEB_SERVER_DISABLED,
|
|
WEB_SERVER_ENABLED
|
|
} WebServerState;
|
|
|
|
|
|
#define E_HTTP_SYNTAX -6
|
|
|
|
|
|
/*!
|
|
* \brief Retrieve interface information and keep it in global variables.
|
|
* If NULL, we'll find the first suitable interface for operation.
|
|
*
|
|
* The interface must fulfill these requirements:
|
|
* \li Be UP.
|
|
* \li Not be LOOPBACK.
|
|
* \li Support MULTICAST.
|
|
* \li Have a valid IPv4 or IPv6 address.
|
|
*
|
|
* We'll retrieve the following information from the interface:
|
|
* \li gIF_NAME -> Interface name (by input or found).
|
|
* \li gIF_IPV4 -> IPv4 address (if any).
|
|
* \li gIF_IPV6 -> IPv6 address (if any).
|
|
* \li gIF_INDEX -> Interface index number.
|
|
*
|
|
* \return UPNP_E_SUCCESS on success.
|
|
*/
|
|
int UpnpGetIfInfo(
|
|
/*! [in] Interface name (can be NULL). */
|
|
const char *IfName);
|
|
|
|
|
|
/*!
|
|
* \brief Initialize handle table.
|
|
*/
|
|
void InitHandleList();
|
|
|
|
|
|
/*!
|
|
* \brief Get a free handle.
|
|
*
|
|
* \return On success, an integer greater than zero or UPNP_E_OUTOF_HANDLE on
|
|
* failure.
|
|
*/
|
|
int GetFreeHandle();
|
|
|
|
|
|
/*!
|
|
* \brief Free handle.
|
|
*
|
|
* \return UPNP_E_SUCCESS if successful or UPNP_E_INVALID_HANDLE if not
|
|
*/
|
|
int FreeHandle(
|
|
/*! [in] Handle index. */
|
|
int Handle);
|
|
|
|
|
|
void UpnpThreadDistribution(struct UpnpNonblockParam * Param);
|
|
|
|
|
|
/*!
|
|
* \brief This function is a timer thread scheduled by UpnpSendAdvertisement
|
|
* to the send advetisement again.
|
|
*/
|
|
void AutoAdvertise(
|
|
/*! [in] Information provided to the thread. */
|
|
void *input);
|
|
|
|
|
|
/*!
|
|
* \brief Get local IP address.
|
|
*
|
|
* Gets the ip address for the DEFAULT_INTERFACE interface which is up and not
|
|
* a loopback. Assumes at most MAX_INTERFACES interfaces
|
|
*
|
|
* \return UPNP_E_SUCCESS if successful or UPNP_E_INIT.
|
|
*/
|
|
int getlocalhostname(
|
|
/*! [out] IP address of the interface. */
|
|
char *out,
|
|
/*! [in] Length of the output buffer. */
|
|
const int out_len);
|
|
|
|
|
|
/*!
|
|
* \brief Print handle info.
|
|
*
|
|
* \return UPNP_E_SUCCESS if successful, otherwise returns appropriate error.
|
|
*/
|
|
int PrintHandleInfo(
|
|
/*! [in] Handle index. */
|
|
UpnpClient_Handle Hnd);
|
|
|
|
|
|
extern WebServerState bWebServerState;
|
|
|
|
|
|
#endif /* UPNPAPI_H */
|
|
|