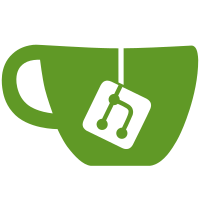
Windows sockets functions look on the outside like they behave similarly to POSIX functions, but there are many subtle and glaring differences, including errors reported via WSAGetLastError, read, write, and close do not work on sockets, setsockopt takes a (char *) rather than (void *), etc. This header implements wrappers that coerce more POSIX-like behavior from these functions, making portable code easier to develop. BENEFITS: One does not necessarily need to sprinkle #ifdefs around code to handle the Windows and non-Windows behavior when porting code. CAVEATS: There may be performance implications with the 'mother-may-I' approach to determining if a descriptor is a socket or a file. The errno mappings are not 100% what one might expect compared to POSIX since there were not always good 1:1 equivalents from the WSA errors.
70 lines
1.4 KiB
C
70 lines
1.4 KiB
C
/*
|
|
* Public domain
|
|
* string.h compatibility shim
|
|
*/
|
|
|
|
#include_next <string.h>
|
|
|
|
#ifndef LIBCRYPTOCOMPAT_STRING_H
|
|
#define LIBCRYPTOCOMPAT_STRING_H
|
|
|
|
#include <sys/types.h>
|
|
|
|
#if defined(__sun) || defined(__hpux)
|
|
/* Some functions historically defined in string.h were placed in strings.h by
|
|
* SUS. Use the same hack as OS X and FreeBSD use to work around on Solaris and HPUX.
|
|
*/
|
|
#include <strings.h>
|
|
#endif
|
|
|
|
#ifndef HAVE_STRLCPY
|
|
size_t strlcpy(char *dst, const char *src, size_t siz);
|
|
#endif
|
|
|
|
#ifndef HAVE_STRLCAT
|
|
size_t strlcat(char *dst, const char *src, size_t siz);
|
|
#endif
|
|
|
|
#ifndef HAVE_STRNDUP
|
|
char * strndup(const char *str, size_t maxlen);
|
|
/* the only user of strnlen is strndup, so only build it if needed */
|
|
#ifndef HAVE_STRNLEN
|
|
size_t strnlen(const char *str, size_t maxlen);
|
|
#endif
|
|
#endif
|
|
|
|
#ifndef HAVE_EXPLICIT_BZERO
|
|
void explicit_bzero(void *, size_t);
|
|
#endif
|
|
|
|
#ifndef HAVE_TIMINGSAFE_BCMP
|
|
int timingsafe_bcmp(const void *b1, const void *b2, size_t n);
|
|
#endif
|
|
|
|
#ifndef HAVE_TIMINGSAFE_MEMCMP
|
|
int timingsafe_memcmp(const void *b1, const void *b2, size_t len);
|
|
#endif
|
|
|
|
#ifndef HAVE_MEMMEM
|
|
void * memmem(const void *big, size_t big_len, const void *little,
|
|
size_t little_len);
|
|
#endif
|
|
|
|
#ifdef _WIN32
|
|
#include <errno.h>
|
|
|
|
static inline char *
|
|
posix_strerror(int errnum)
|
|
{
|
|
if (errnum == ECONNREFUSED) {
|
|
return "Connection refused";
|
|
}
|
|
return strerror(errnum);
|
|
}
|
|
|
|
#define strerror(errnum) posix_strerror(errnum)
|
|
|
|
#endif
|
|
|
|
#endif
|