mirror of
https://github.com/intel/isa-l.git
synced 2024-12-12 17:33:50 +01:00
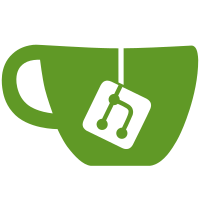
This patch addresses one build failure and fixes several build warnings for Arm (some for x86 too). - Fix dynamic relocation link failure of ld.bfd 2.30 on Arm [log] relocation R_AARCH64_ADR_PREL_PG_HI21 against symbol `xor_gen_neon' which may bind externally can not be used when making a shared object - Add arch dependent "other_tests" to exclude x86 specific tests on Arm [log] isa-l/erasure_code/gf_2vect_dot_prod_sse_test.c:181: undefined reference to `gf_2vect_dot_prod_sse' - Check "fread" return value to fix gcc warnings on Arm and x86 [log] warning: ignoring return value of ‘fread’, declared with attribute warn_unused_result [-Wunused-result] fread(in_buf, 1, in_size, in_file); ^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ - Fix issue of comparing "char" with "int" on Arm. "char" is unsigned on Arm by default, an unsigned char will never equal to EOF(-1). [Log] programs/igzip_cli.c:318:31: warning: comparison is always true due to limited range of data type [-Wtype-limits] while (tmp != '\n' && tmp != EOF) ^~ - Include <stdlib.h> to several files to fix build warnings on Arm [log] igzip/igzip_inflate_perf.c:339:5: warning: incompatible implicit declaration of built-in function ‘exit’ exit(0); ^~~~ Change-Id: I82c1b63316b634b3d398ffba2ff815679d9051a8 Signed-off-by: Yibo Cai <yibo.cai@arm.com>
42 lines
913 B
C
42 lines
913 B
C
#define _FILE_OFFSET_BITS 64
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <assert.h>
|
|
#include <zlib.h>
|
|
#include "huff_codes.h"
|
|
#include "igzip_lib.h"
|
|
#include "test.h"
|
|
|
|
extern int LLVMFuzzerTestOneInput(const uint8_t * data, size_t size);
|
|
|
|
int main(int argc, char *argv[])
|
|
{
|
|
FILE *in = NULL;
|
|
unsigned char *in_buf = NULL;
|
|
uint64_t in_file_size;
|
|
|
|
if (argc != 2) {
|
|
fprintf(stderr, "Usage: isal_fuzz_inflate <infile>\n");
|
|
exit(1);
|
|
}
|
|
in = fopen(argv[1], "rb");
|
|
if (!in) {
|
|
fprintf(stderr, "Can't open %s for reading\n", argv[1]);
|
|
exit(1);
|
|
}
|
|
in_file_size = get_filesize(in);
|
|
in_buf = malloc(in_file_size);
|
|
|
|
if (in_buf == NULL) {
|
|
fprintf(stderr, "Failed to malloc input and outputs buffers\n");
|
|
exit(1);
|
|
}
|
|
|
|
if (fread(in_buf, 1, in_file_size, in) != in_file_size) {
|
|
fprintf(stderr, "Failed to read from %s\n", argv[1]);
|
|
exit(1);
|
|
}
|
|
|
|
return LLVMFuzzerTestOneInput(in_buf, in_file_size);
|
|
}
|