mirror of
https://github.com/KjellKod/g3log.git
synced 2024-12-12 10:23:50 +01:00
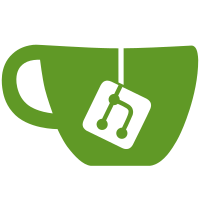
--HG-- rename : g2log/Build.cmake => Build.cmake rename : g2log/CMakeLists.txt => CMakeLists.txt rename : g2log/CPackLists.txt => CPackLists.txt rename : g2log/Dynamic.cmake => Dynamic.cmake rename : g2log/example/Example.cmake => example/Example.cmake rename : g2log/example/main_contract.cpp => example/main_contract.cpp rename : g2log/example/main_sigsegv.cpp => example/main_sigsegv.cpp rename : g2log/src/active.hpp => src/active.hpp rename : g2log/src/crashhandler.hpp => src/crashhandler.hpp rename : g2log/src/crashhandler_unix.cpp => src/crashhandler_unix.cpp rename : g2log/src/crashhandler_win.cpp => src/crashhandler_win.cpp rename : g2log/src/g2filesink.cpp => src/g2filesink.cpp rename : g2log/src/g2filesink.hpp => src/g2filesink.hpp rename : g2log/src/g2filesinkhelper.ipp => src/g2filesinkhelper.ipp rename : g2log/src/g2future.hpp => src/g2future.hpp rename : g2log/src/g2log.cpp => src/g2log.cpp rename : g2log/src/g2log.hpp => src/g2log.hpp rename : g2log/src/g2loglevels.cpp => src/g2loglevels.cpp rename : g2log/src/g2loglevels.hpp => src/g2loglevels.hpp rename : g2log/src/g2logmessage.cpp => src/g2logmessage.cpp rename : g2log/src/g2logmessage.hpp => src/g2logmessage.hpp rename : g2log/src/g2logmessagecapture.cpp => src/g2logmessagecapture.cpp rename : g2log/src/g2logmessagecapture.hpp => src/g2logmessagecapture.hpp rename : g2log/src/g2logworker.cpp => src/g2logworker.cpp rename : g2log/src/g2logworker.hpp => src/g2logworker.hpp rename : g2log/src/g2moveoncopy.hpp => src/g2moveoncopy.hpp rename : g2log/src/g2sink.hpp => src/g2sink.hpp rename : g2log/src/g2sinkhandle.hpp => src/g2sinkhandle.hpp rename : g2log/src/g2sinkwrapper.hpp => src/g2sinkwrapper.hpp rename : g2log/src/g2time.cpp => src/g2time.cpp rename : g2log/src/g2time.hpp => src/g2time.hpp rename : g2log/src/shared_queue.hpp => src/shared_queue.hpp rename : g2log/src/std2_make_unique.hpp => src/std2_make_unique.hpp rename : g2log/src/stlpatch_future.hpp => src/stlpatch_future.hpp rename : g2log/test_performance/Performance.cmake => test_performance/Performance.cmake rename : g2log/test_performance/main_threaded_mean.cpp => test_performance/main_threaded_mean.cpp rename : g2log/test_performance/main_threaded_worst.cpp => test_performance/main_threaded_worst.cpp rename : g2log/test_performance/performance.h => test_performance/performance.h rename : g2log/test_unit/Test.cmake => test_unit/Test.cmake rename : g2log/test_unit/test_concept_sink.cpp => test_unit/test_concept_sink.cpp rename : g2log/test_unit/test_configuration.cpp => test_unit/test_configuration.cpp rename : g2log/test_unit/test_filechange.cpp => test_unit/test_filechange.cpp rename : g2log/test_unit/test_io.cpp => test_unit/test_io.cpp rename : g2log/test_unit/test_linux_dynamic_loaded_sharedlib.cpp => test_unit/test_linux_dynamic_loaded_sharedlib.cpp rename : g2log/test_unit/test_sink.cpp => test_unit/test_sink.cpp rename : g2log/test_unit/tester_sharedlib.cpp => test_unit/tester_sharedlib.cpp rename : g2log/test_unit/tester_sharedlib.h => test_unit/tester_sharedlib.h rename : g2log/test_unit/testing_helpers.cpp => test_unit/testing_helpers.cpp rename : g2log/test_unit/testing_helpers.h => test_unit/testing_helpers.h
62 lines
2.3 KiB
C++
62 lines
2.3 KiB
C++
/** ==========================================================================
|
|
* 2011 by KjellKod.cc. This is PUBLIC DOMAIN to use at your own risk and comes
|
|
* with no warranties. This code is yours to share, use and modify with no
|
|
* strings attached and no restrictions or obligations.
|
|
*
|
|
* For more information see g3log/LICENSE or refer refer to http://unlicense.org
|
|
* ============================================================================*/
|
|
|
|
#include "g2logworker.hpp"
|
|
#include "g2log.hpp"
|
|
#include <iomanip>
|
|
#include <thread>
|
|
#include <iostream>
|
|
namespace
|
|
{
|
|
#if (defined(WIN32) || defined(_WIN32) || defined(__WIN32__))
|
|
const std::string path_to_log_file = "./";
|
|
#else
|
|
const std::string path_to_log_file = "/tmp/";
|
|
#endif
|
|
}
|
|
|
|
namespace example_fatal
|
|
{
|
|
void killWithContractIfNonEqual(int first, int second)
|
|
{
|
|
CHECK(first == second) << "Test to see if contract works: onetwothree: " << 123 << ". This should be at the end of the log, and will exit this example";
|
|
}
|
|
} // example fatal
|
|
|
|
int main(int argc, char** argv)
|
|
{
|
|
double pi_d = 3.1415926535897932384626433832795;
|
|
float pi_f = 3.1415926535897932384626433832795f;
|
|
|
|
auto logger_n_handle = g2::LogWorker::createWithDefaultLogger(argv[0], path_to_log_file);
|
|
g2::initializeLogging(logger_n_handle.worker.get());
|
|
std::future<std::string> log_file_name = logger_n_handle.sink->call(&g2::FileSink::fileName);
|
|
std::cout << "* This is an example of g2log. It WILL exit by a failed CHECK(...)" << std::endl;
|
|
std::cout << "* that acts as a FATAL trigger. Please see the generated log and " << std::endl;
|
|
std::cout << "* compare to the code at:\n* \t g2log/test_example/main_contract.cpp" << std::endl;
|
|
std::cout << "*\n* Log file: [" << log_file_name.get() << "]\n\n" << std::endl;
|
|
|
|
LOGF(INFO, "Hi log %d", 123);
|
|
LOG(INFO) << "Test SLOG INFO";
|
|
LOG(DEBUG) << "Test SLOG DEBUG";
|
|
LOG(INFO) << "one: " << 1;
|
|
LOG(INFO) << "two: " << 2;
|
|
LOG(INFO) << "one and two: " << 1 << " and " << 2;
|
|
LOG(DEBUG) << "float 2.14: " << 1000/2.14f;
|
|
LOG(DEBUG) << "pi double: " << pi_d;
|
|
LOG(DEBUG) << "pi float: " << pi_f;
|
|
LOG(DEBUG) << "pi float (width 10): " << std::setprecision(10) << pi_f;
|
|
LOGF(INFO, "pi float printf:%f", pi_f);
|
|
|
|
// FATAL SECTION
|
|
int smaller = 1;
|
|
int larger = 2;
|
|
example_fatal::killWithContractIfNonEqual(smaller, larger);
|
|
}
|
|
|