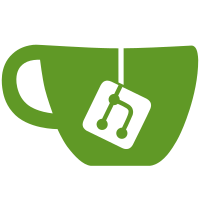
One of the last sections of tests that still fail in C++03 are the unique_ptr tests. This patch begins cleaning up the tests and fixing C++03 failures. The main changes of this patch: - The "Deleter" type in "deleter.h" tried to be "move-only" in C++03. However the move simulation no longer works (see "__rv"). "Deleter" is now copy constructible in C++03. However copying "Deleter" will "move" the test value instead of copying it. - Reduce the unique.ptr.single.ctor tests files from ~25 to 4. There is no reason the tests were split through so many files. git-svn-id: https://llvm.org/svn/llvm-project/libcxx/trunk@243730 91177308-0d34-0410-b5e6-96231b3b80d8
29 lines
752 B
C++
29 lines
752 B
C++
//===----------------------------------------------------------------------===//
|
|
//
|
|
// The LLVM Compiler Infrastructure
|
|
//
|
|
// This file is dual licensed under the MIT and the University of Illinois Open
|
|
// Source Licenses. See LICENSE.TXT for details.
|
|
//
|
|
//===----------------------------------------------------------------------===//
|
|
|
|
// <memory>
|
|
|
|
// unique_ptr
|
|
|
|
// Test unique_ptr move assignment
|
|
|
|
#include <memory>
|
|
|
|
#include "test_macros.h"
|
|
|
|
int main()
|
|
{
|
|
std::unique_ptr<int> s, s2;
|
|
#if TEST_STD_VER >= 11
|
|
s2 = s; // expected-error {{cannot be assigned because its copy assignment operator is implicitly deleted}}
|
|
#else
|
|
s2 = s; // expected-error {{'operator=' is a private member of 'std::__1::unique_ptr}}
|
|
#endif
|
|
}
|