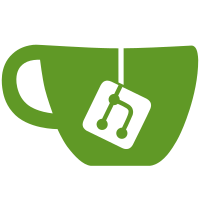
git-svn-id: https://llvm.org/svn/llvm-project/libcxx/trunk@121064 91177308-0d34-0410-b5e6-96231b3b80d8
10330 lines
380 KiB
C++
10330 lines
380 KiB
C++
// -*- C++ -*-
|
||
//===--------------------------- atomic -----------------------------------===//
|
||
//
|
||
// The LLVM Compiler Infrastructure
|
||
//
|
||
// This file is distributed under the University of Illinois Open Source
|
||
// License. See LICENSE.TXT for details.
|
||
//
|
||
//===----------------------------------------------------------------------===//
|
||
|
||
#ifndef _LIBCPP_ATOMIC
|
||
#define _LIBCPP_ATOMIC
|
||
|
||
/*
|
||
atomic synopsis
|
||
|
||
namespace std
|
||
{
|
||
|
||
// order and consistency
|
||
|
||
typedef enum memory_order
|
||
{
|
||
memory_order_relaxed,
|
||
memory_order_consume, // load-consume
|
||
memory_order_acquire, // load-acquire
|
||
memory_order_release, // store-release
|
||
memory_order_acq_rel, // store-release load-acquire
|
||
memory_order_seq_cst // store-release load-acquire
|
||
} memory_order;
|
||
|
||
template <class T> T kill_dependency(T y);
|
||
|
||
// lock-free property
|
||
|
||
#define ATOMIC_CHAR_LOCK_FREE unspecified
|
||
#define ATOMIC_CHAR16_T_LOCK_FREE unspecified
|
||
#define ATOMIC_CHAR32_T_LOCK_FREE unspecified
|
||
#define ATOMIC_WCHAR_T_LOCK_FREE unspecified
|
||
#define ATOMIC_SHORT_LOCK_FREE unspecified
|
||
#define ATOMIC_INT_LOCK_FREE unspecified
|
||
#define ATOMIC_LONG_LOCK_FREE unspecified
|
||
#define ATOMIC_LLONG_LOCK_FREE unspecified
|
||
|
||
// flag type and operations
|
||
|
||
typedef struct atomic_flag
|
||
{
|
||
bool test_and_set(memory_order m = memory_order_seq_cst) volatile;
|
||
bool test_and_set(memory_order m = memory_order_seq_cst);
|
||
void clear(memory_order m = memory_order_seq_cst) volatile;
|
||
void clear(memory_order m = memory_order_seq_cst);
|
||
atomic_flag() = default;
|
||
atomic_flag(const atomic_flag&) = delete;
|
||
atomic_flag& operator=(const atomic_flag&) = delete;
|
||
atomic_flag& operator=(const atomic_flag&) volatile = delete;
|
||
} atomic_flag;
|
||
|
||
bool
|
||
atomic_flag_test_and_set(volatile atomic_flag* obj);
|
||
|
||
bool
|
||
atomic_flag_test_and_set(atomic_flag* obj);
|
||
|
||
bool
|
||
atomic_flag_test_and_set_explicit(volatile atomic_flag* obj,
|
||
memory_order m);
|
||
|
||
bool
|
||
atomic_flag_test_and_set_explicit(atomic_flag* obj, memory_order m);
|
||
|
||
void
|
||
atomic_flag_clear(volatile atomic_flag* obj);
|
||
|
||
void
|
||
atomic_flag_clear(atomic_flag* obj);
|
||
|
||
void
|
||
atomic_flag_clear_explicit(volatile atomic_flag* obj, memory_order m);
|
||
|
||
void
|
||
atomic_flag_clear_explicit(atomic_flag* obj, memory_order m);
|
||
|
||
#define ATOMIC_FLAG_INIT see below
|
||
#define ATOMIC_VAR_INIT(value) see below
|
||
|
||
template <class T>
|
||
struct atomic
|
||
{
|
||
bool is_lock_free() const volatile;
|
||
bool is_lock_free() const;
|
||
void store(T desr, memory_order m = memory_order_seq_cst) volatile;
|
||
void store(T desr, memory_order m = memory_order_seq_cst);
|
||
T load(memory_order m = memory_order_seq_cst) const volatile;
|
||
T load(memory_order m = memory_order_seq_cst) const;
|
||
operator T() const volatile;
|
||
operator T() const;
|
||
T exchange(T desr, memory_order m = memory_order_seq_cst) volatile;
|
||
T exchange(T desr, memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_weak(T& expc, T desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_weak(T& expc, T desr, memory_order s, memory_order f);
|
||
bool compare_exchange_strong(T& expc, T desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_strong(T& expc, T desr,
|
||
memory_order s, memory_order f);
|
||
bool compare_exchange_weak(T& expc, T desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_weak(T& expc, T desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_strong(T& expc, T desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_strong(T& expc, T desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
|
||
atomic() = default;
|
||
constexpr atomic(T desr);
|
||
atomic(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) volatile = delete;
|
||
T operator=(T) volatile;
|
||
T operator=(T);
|
||
};
|
||
|
||
template <>
|
||
struct atomic<integral>
|
||
{
|
||
bool is_lock_free() const volatile;
|
||
bool is_lock_free() const;
|
||
void store(integral desr, memory_order m = memory_order_seq_cst) volatile;
|
||
void store(integral desr, memory_order m = memory_order_seq_cst);
|
||
integral load(memory_order m = memory_order_seq_cst) const volatile;
|
||
integral load(memory_order m = memory_order_seq_cst) const;
|
||
operator integral() const volatile;
|
||
operator integral() const;
|
||
integral exchange(integral desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
integral exchange(integral desr, memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_weak(integral& expc, integral desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_weak(integral& expc, integral desr,
|
||
memory_order s, memory_order f);
|
||
bool compare_exchange_strong(integral& expc, integral desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_strong(integral& expc, integral desr,
|
||
memory_order s, memory_order f);
|
||
bool compare_exchange_weak(integral& expc, integral desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_weak(integral& expc, integral desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_strong(integral& expc, integral desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_strong(integral& expc, integral desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
|
||
integral
|
||
fetch_add(integral op, memory_order m = memory_order_seq_cst) volatile;
|
||
integral fetch_add(integral op, memory_order m = memory_order_seq_cst);
|
||
integral
|
||
fetch_sub(integral op, memory_order m = memory_order_seq_cst) volatile;
|
||
integral fetch_sub(integral op, memory_order m = memory_order_seq_cst);
|
||
integral
|
||
fetch_and(integral op, memory_order m = memory_order_seq_cst) volatile;
|
||
integral fetch_and(integral op, memory_order m = memory_order_seq_cst);
|
||
integral
|
||
fetch_or(integral op, memory_order m = memory_order_seq_cst) volatile;
|
||
integral fetch_or(integral op, memory_order m = memory_order_seq_cst);
|
||
integral
|
||
fetch_xor(integral op, memory_order m = memory_order_seq_cst) volatile;
|
||
integral fetch_xor(integral op, memory_order m = memory_order_seq_cst);
|
||
|
||
atomic() = default;
|
||
constexpr atomic(integral desr);
|
||
atomic(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) volatile = delete;
|
||
integral operator=(integral desr) volatile;
|
||
integral operator=(integral desr);
|
||
|
||
integral operator++(int) volatile;
|
||
integral operator++(int);
|
||
integral operator--(int) volatile;
|
||
integral operator--(int);
|
||
integral operator++() volatile;
|
||
integral operator++();
|
||
integral operator--() volatile;
|
||
integral operator--();
|
||
integral operator+=(integral op) volatile;
|
||
integral operator+=(integral op);
|
||
integral operator-=(integral op) volatile;
|
||
integral operator-=(integral op);
|
||
integral operator&=(integral op) volatile;
|
||
integral operator&=(integral op);
|
||
integral operator|=(integral op) volatile;
|
||
integral operator|=(integral op);
|
||
integral operatorˆ=(integral op) volatile;
|
||
integral operatorˆ=(integral op);
|
||
};
|
||
|
||
template <class T>
|
||
struct atomic<T*>
|
||
{
|
||
bool is_lock_free() const volatile;
|
||
bool is_lock_free() const;
|
||
void store(T* desr, memory_order m = memory_order_seq_cst) volatile;
|
||
void store(T* desr, memory_order m = memory_order_seq_cst);
|
||
T* load(memory_order m = memory_order_seq_cst) const volatile;
|
||
T* load(memory_order m = memory_order_seq_cst) const;
|
||
operator T*() const volatile;
|
||
operator T*() const;
|
||
T* exchange(T* desr, memory_order m = memory_order_seq_cst) volatile;
|
||
T* exchange(T* desr, memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_weak(T*& expc, T* desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_weak(T*& expc, T* desr,
|
||
memory_order s, memory_order f);
|
||
bool compare_exchange_strong(T*& expc, T* desr,
|
||
memory_order s, memory_order f) volatile;
|
||
bool compare_exchange_strong(T*& expc, T* desr,
|
||
memory_order s, memory_order f);
|
||
bool compare_exchange_weak(T*& expc, T* desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_weak(T*& expc, T* desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
bool compare_exchange_strong(T*& expc, T* desr,
|
||
memory_order m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_strong(T*& expc, T* desr,
|
||
memory_order m = memory_order_seq_cst);
|
||
T* fetch_add(ptrdiff_t op, memory_order m = memory_order_seq_cst) volatile;
|
||
T* fetch_add(ptrdiff_t op, memory_order m = memory_order_seq_cst);
|
||
T* fetch_sub(ptrdiff_t op, memory_order m = memory_order_seq_cst) volatile;
|
||
T* fetch_sub(ptrdiff_t op, memory_order m = memory_order_seq_cst);
|
||
|
||
atomic() = default;
|
||
constexpr atomic(T* desr);
|
||
atomic(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) volatile = delete;
|
||
|
||
T* operator=(T*) volatile;
|
||
T* operator=(T*);
|
||
T* operator++(int) volatile;
|
||
T* operator++(int);
|
||
T* operator--(int) volatile;
|
||
T* operator--(int);
|
||
T* operator++() volatile;
|
||
T* operator++();
|
||
T* operator--() volatile;
|
||
T* operator--();
|
||
T* operator+=(ptrdiff_t op) volatile;
|
||
T* operator+=(ptrdiff_t op);
|
||
T* operator-=(ptrdiff_t op) volatile;
|
||
T* operator-=(ptrdiff_t op);
|
||
};
|
||
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic<T>* obj);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_is_lock_free(const atomic<T>* obj);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_init(volatile atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_init(atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_store(volatile atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_store(atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_store_explicit(volatile atomic<T>* obj, T desr, memory_order m);
|
||
|
||
template <class T>
|
||
void
|
||
atomic_store_explicit(atomic<T>* obj, T desr, memory_order m);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_load(const volatile atomic<T>* obj);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_load(const atomic<T>* obj);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_load_explicit(const volatile atomic<T>* obj, memory_order m);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_load_explicit(const atomic<T>* obj, memory_order m);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_exchange(volatile atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_exchange(atomic<T>* obj, T desr);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_exchange_explicit(volatile atomic<T>* obj, T desr, memory_order m);
|
||
|
||
template <class T>
|
||
T
|
||
atomic_exchange_explicit(atomic<T>* obj, T desr, memory_order m);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic<T>* obj, T* expc, T desr);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_weak(atomic<T>* obj, T* expc, T desr);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic<T>* obj, T* expc, T desr);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_strong(atomic<T>* obj, T* expc, T desr);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic<T>* obj, T* expc,
|
||
T desr,
|
||
memory_order s, memory_order f);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic<T>* obj, T* expc, T desr,
|
||
memory_order s, memory_order f);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic<T>* obj,
|
||
T* expc, T desr,
|
||
memory_order s, memory_order f);
|
||
|
||
template <class T>
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic<T>* obj, T* expc,
|
||
T desr,
|
||
memory_order s, memory_order f);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_add(volatile atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_add(atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_add_explicit(volatile atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_add_explicit(atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_sub(volatile atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_sub(atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_sub_explicit(volatile atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_sub_explicit(atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_and(volatile atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_and(atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_and_explicit(volatile atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_and_explicit(atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_or(volatile atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_or(atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_or_explicit(volatile atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_or_explicit(atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_xor(volatile atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_xor(atomic<Integral>* obj, Integral op);
|
||
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_xor_explicit(volatile atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
template <class Integral>
|
||
Integral
|
||
atomic_fetch_xor_explicit(atomic<Integral>* obj, Integral op,
|
||
memory_order m);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_add(volatile atomic<T*>* obj, ptrdiff_t op);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_add(atomic<T*>* obj, ptrdiff_t op);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_add_explicit(volatile atomic<T*>* obj, ptrdiff_t op,
|
||
memory_order m);
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_add_explicit(atomic<T*>* obj, ptrdiff_t op, memory_order m);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_sub(volatile atomic<T*>* obj, ptrdiff_t op);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_sub(atomic<T*>* obj, ptrdiff_t op);
|
||
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_sub_explicit(volatile atomic<T*>* obj, ptrdiff_t op,
|
||
memory_order m);
|
||
template <class T>
|
||
T*
|
||
atomic_fetch_sub_explicit(atomic<T*>* obj, ptrdiff_t op, memory_order m);
|
||
|
||
// Atomics for standard typedef types
|
||
|
||
typedef atomic<char> atomic_char;
|
||
typedef atomic<signed char> atomic_schar;
|
||
typedef atomic<unsigned char> atomic_uchar;
|
||
typedef atomic<short> atomic_short;
|
||
typedef atomic<unsigned short> atomic_ushort;
|
||
typedef atomic<int> atomic_int;
|
||
typedef atomic<unsigned int> atomic_uint;
|
||
typedef atomic<long> atomic_long;
|
||
typedef atomic<unsigned long> atomic_ulong;
|
||
typedef atomic<long long> atomic_llong;
|
||
typedef atomic<unsigned long long> atomic_ullong;
|
||
typedef atomic<char16_t> atomic_char16_t;
|
||
typedef atomic<char32_t> atomic_char32_t;
|
||
typedef atomic<wchar_t> atomic_wchar_t;
|
||
|
||
typedef atomic<int_least8_t> atomic_int_least8_t;
|
||
typedef atomic<uint_least8_t> atomic_uint_least8_t;
|
||
typedef atomic<int_least16_t> atomic_int_least16_t;
|
||
typedef atomic<uint_least16_t> atomic_uint_least16_t;
|
||
typedef atomic<int_least32_t> atomic_int_least32_t;
|
||
typedef atomic<uint_least32_t> atomic_uint_least32_t;
|
||
typedef atomic<int_least64_t> atomic_int_least64_t;
|
||
typedef atomic<uint_least64_t> atomic_uint_least64_t;
|
||
|
||
typedef atomic<int_fast8_t> atomic_int_fast8_t;
|
||
typedef atomic<uint_fast8_t> atomic_uint_fast8_t;
|
||
typedef atomic<int_fast16_t> atomic_int_fast16_t;
|
||
typedef atomic<uint_fast16_t> atomic_uint_fast16_t;
|
||
typedef atomic<int_fast32_t> atomic_int_fast32_t;
|
||
typedef atomic<uint_fast32_t> atomic_uint_fast32_t;
|
||
typedef atomic<int_fast64_t> atomic_int_fast64_t;
|
||
typedef atomic<uint_fast64_t> atomic_uint_fast64_t;
|
||
|
||
typedef atomic<intptr_t> atomic_intptr_t;
|
||
typedef atomic<uintptr_t> atomic_uintptr_t;
|
||
typedef atomic<size_t> atomic_size_t;
|
||
typedef atomic<ptrdiff_t> atomic_ptrdiff_t;
|
||
typedef atomic<intmax_t> atomic_intmax_t;
|
||
typedef atomic<uintmax_t> atomic_uintmax_t;
|
||
|
||
// fences
|
||
|
||
void atomic_thread_fence(memory_order m);
|
||
void atomic_signal_fence(memory_order m);
|
||
|
||
} // std
|
||
|
||
*/
|
||
|
||
#include <__config>
|
||
#include <cstddef>
|
||
#include <cstdint>
|
||
#include <type_traits>
|
||
|
||
#pragma GCC system_header
|
||
|
||
//// Begin Temporary Intrinsics ////
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
__atomic_is_lock_free(_Tp)
|
||
{
|
||
return false;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
__atomic_load(const volatile _Tp* __t, int)
|
||
{
|
||
return *__t;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
__atomic_store(volatile _Tp* __t, _Tp __d, int)
|
||
{
|
||
*__t = __d;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
__atomic_exchange(volatile _Tp* __t, _Tp __d, int)
|
||
{
|
||
_Tp __tmp = *__t;
|
||
*__t = __d;
|
||
return __tmp;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
__atomic_compare_exchange_strong(volatile _Tp* __o, _Tp* __e, _Tp __d, int, int)
|
||
{
|
||
if (const_cast<_Tp&>(*__o) == *__e)
|
||
{
|
||
*__o = __d;
|
||
return true;
|
||
}
|
||
*__e = __d;
|
||
return false;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
__atomic_compare_exchange_weak(volatile _Tp* __o, _Tp* __e, _Tp __d, int, int)
|
||
{
|
||
if (const_cast<_Tp&>(*__o) == *__e)
|
||
{
|
||
*__o = __d;
|
||
return true;
|
||
}
|
||
*__e = __d;
|
||
return false;
|
||
}
|
||
|
||
//// End Temporary Intrinsics ////
|
||
|
||
_LIBCPP_BEGIN_NAMESPACE_STD
|
||
|
||
typedef enum memory_order
|
||
{
|
||
memory_order_relaxed, memory_order_consume, memory_order_acquire,
|
||
memory_order_release, memory_order_acq_rel, memory_order_seq_cst
|
||
} memory_order;
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
kill_dependency(_Tp __y)
|
||
{
|
||
return __y;
|
||
}
|
||
|
||
template <class T> struct atomic;
|
||
|
||
// atomic_is_lock_free
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic<_Tp>*)
|
||
{
|
||
return __atomic_is_lock_free(_Tp());
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic<_Tp>*)
|
||
{
|
||
return __atomic_is_lock_free(_Tp());
|
||
}
|
||
|
||
// atomic_init
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
__o->__a_ = __d;
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
__o->__a_ = __d;
|
||
}
|
||
|
||
// atomic_store
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
__atomic_store(&__o->__a_, __d, memory_order_seq_cst);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
__atomic_store(&__o->__a_, __d, memory_order_seq_cst);
|
||
}
|
||
|
||
// atomic_store_explicit
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic<_Tp>* __o, _Tp __d, memory_order __m)
|
||
{
|
||
__atomic_store(&__o->__a_, __d, __m);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic<_Tp>* __o, _Tp __d, memory_order __m)
|
||
{
|
||
__atomic_store(&__o->__a_, __d, __m);
|
||
}
|
||
|
||
// atomic_load
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_load(const volatile atomic<_Tp>* __o)
|
||
{
|
||
return __atomic_load(&__o->__a_, memory_order_seq_cst);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_load(const atomic<_Tp>* __o)
|
||
{
|
||
return __atomic_load(&__o->__a_, memory_order_seq_cst);
|
||
}
|
||
|
||
// atomic_load_explicit
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_load_explicit(const volatile atomic<_Tp>* __o, memory_order __m)
|
||
{
|
||
return __atomic_load(&__o->__a_, __m);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_load_explicit(const atomic<_Tp>* __o, memory_order __m)
|
||
{
|
||
return __atomic_load(&__o->__a_, __m);
|
||
}
|
||
|
||
// atomic_exchange
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_exchange(volatile atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
return __atomic_exchange(&__o->__a_, __d, memory_order_seq_cst);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_exchange(atomic<_Tp>* __o, _Tp __d)
|
||
{
|
||
return __atomic_exchange(&__o->__a_, __d, memory_order_seq_cst);
|
||
}
|
||
|
||
// atomic_exchange_explicit
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_exchange_explicit(volatile atomic<_Tp>* __o, _Tp __d, memory_order __m)
|
||
{
|
||
return __atomic_exchange(&__o->__a_, __d, __m);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
_Tp
|
||
atomic_exchange_explicit(atomic<_Tp>* __o, _Tp __d, memory_order __m)
|
||
{
|
||
return __atomic_exchange(&__o->__a_, __d, __m);
|
||
}
|
||
|
||
// atomic_compare_exchange_weak
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic<_Tp>* __o, _Tp* __e, _Tp __d)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__o->__a_, __e, __d,
|
||
memory_order_seq_cst,
|
||
memory_order_seq_cst);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic<_Tp>* __o, _Tp* __e, _Tp __d)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__o->__a_, __e, __d,
|
||
memory_order_seq_cst,
|
||
memory_order_seq_cst);
|
||
}
|
||
|
||
// atomic_compare_exchange_strong
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic<_Tp>* __o, _Tp* __e, _Tp __d)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__o->__a_, __e, __d,
|
||
memory_order_seq_cst,
|
||
memory_order_seq_cst);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic<_Tp>* __o, _Tp* __e, _Tp __d)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__o->__a_, __e, __d,
|
||
memory_order_seq_cst,
|
||
memory_order_seq_cst);
|
||
}
|
||
|
||
// atomic_compare_exchange_weak_explicit
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic<_Tp>* __o, _Tp* __e,
|
||
_Tp __d,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__o->__a_, __e, __d, __s, __f);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic<_Tp>* __o, _Tp* __e, _Tp __d,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__o->__a_, __e, __d, __s, __f);
|
||
}
|
||
|
||
// atomic_compare_exchange_strong_explicit
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic<_Tp>* __o,
|
||
_Tp* __e, _Tp __d,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__o->__a_, __e, __d, __s, __f);
|
||
}
|
||
|
||
template <class _Tp>
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic<_Tp>* __o, _Tp* __e,
|
||
_Tp __d,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__o->__a_, __e, __d, __s, __f);
|
||
}
|
||
|
||
|
||
// atomic<T>
|
||
|
||
template <class _Tp>
|
||
struct atomic
|
||
{
|
||
_Tp __a_;
|
||
|
||
bool is_lock_free() const volatile;
|
||
bool is_lock_free() const;
|
||
void store(_Tp __d, memory_order __m = memory_order_seq_cst) volatile;
|
||
void store(_Tp __d, memory_order __m = memory_order_seq_cst);
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
_Tp load(memory_order __m = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __m);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
_Tp load(memory_order __m = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __m);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator _Tp() const volatile {return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator _Tp() const {return load();}
|
||
_Tp exchange(_Tp __d, memory_order __m = memory_order_seq_cst) volatile;
|
||
_Tp exchange(_Tp __d, memory_order __m = memory_order_seq_cst);
|
||
bool compare_exchange_weak(_Tp& __e, _Tp __d,
|
||
memory_order __s, memory_order __f) volatile;
|
||
bool compare_exchange_weak(_Tp& __e, _Tp __d,
|
||
memory_order __s, memory_order __f);
|
||
bool compare_exchange_strong(_Tp& __e, _Tp __d,
|
||
memory_order __s, memory_order __f) volatile;
|
||
bool compare_exchange_strong(_Tp& __e, _Tp __d,
|
||
memory_order __s, memory_order __f);
|
||
bool compare_exchange_weak(_Tp& __e, _Tp __d,
|
||
memory_order __m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_weak(_Tp& __e, _Tp __d,
|
||
memory_order __m = memory_order_seq_cst);
|
||
bool compare_exchange_strong(_Tp& __e, _Tp __d,
|
||
memory_order __m = memory_order_seq_cst) volatile;
|
||
bool compare_exchange_strong(_Tp& __e, _Tp __d,
|
||
memory_order __m = memory_order_seq_cst);
|
||
|
||
atomic() {} // = default;
|
||
constexpr atomic(_Tp __d);
|
||
atomic(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) = delete;
|
||
atomic& operator=(const atomic&) volatile = delete;
|
||
_Tp operator=(_Tp) volatile;
|
||
_Tp operator=(_Tp);
|
||
};
|
||
|
||
/*
|
||
// flag type and operations
|
||
|
||
typedef bool __atomic_flag__;
|
||
|
||
struct atomic_flag;
|
||
|
||
bool atomic_flag_test_and_set(volatile atomic_flag*);
|
||
bool atomic_flag_test_and_set(atomic_flag*);
|
||
bool atomic_flag_test_and_set_explicit(volatile atomic_flag*, memory_order);
|
||
bool atomic_flag_test_and_set_explicit(atomic_flag*, memory_order);
|
||
void atomic_flag_clear(volatile atomic_flag*);
|
||
void atomic_flag_clear(atomic_flag*);
|
||
void atomic_flag_clear_explicit(volatile atomic_flag*, memory_order);
|
||
void atomic_flag_clear_explicit(atomic_flag*, memory_order);
|
||
|
||
typedef struct _LIBCPP_VISIBLE atomic_flag
|
||
{
|
||
__atomic_flag__ __flg_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool test_and_set(memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_flag_test_and_set_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool test_and_set(memory_order __o = memory_order_seq_cst)
|
||
{return atomic_flag_test_and_set_explicit(this, __o);}
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void clear(memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_flag_clear_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void clear(memory_order __o = memory_order_seq_cst)
|
||
{atomic_flag_clear_explicit(this, __o);}
|
||
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_flag() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_flag() {};
|
||
#endif
|
||
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_flag(const atomic_flag&) = delete;
|
||
atomic_flag& operator=(const atomic_flag&) = delete;
|
||
atomic_flag& operator=(const atomic_flag&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_flag(const atomic_flag&);
|
||
atomic_flag& operator=(const atomic_flag&);
|
||
atomic_flag& operator=(const atomic_flag&) volatile;
|
||
public:
|
||
#endif
|
||
} atomic_flag;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_flag_test_and_set(volatile atomic_flag* __f)
|
||
{
|
||
return __atomic_exchange(&__f->__flg_, __atomic_flag__(true),
|
||
memory_order_seq_cst)
|
||
== __atomic_flag__(true);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_flag_test_and_set(atomic_flag* __f)
|
||
{
|
||
return atomic_flag_test_and_set(const_cast<volatile atomic_flag*>(__f));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_flag_test_and_set_explicit(volatile atomic_flag* __f, memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__f->__flg_, __atomic_flag__(true), __o)
|
||
== __atomic_flag__(true);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_flag_test_and_set_explicit(atomic_flag* __f, memory_order __o)
|
||
{
|
||
return atomic_flag_test_and_set_explicit(const_cast<volatile atomic_flag*>
|
||
(__f), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_flag_clear(volatile atomic_flag* __f)
|
||
{
|
||
__atomic_store(&__f->__flg_, __atomic_flag__(false), memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_flag_clear(atomic_flag* __f)
|
||
{
|
||
atomic_flag_clear(const_cast<volatile atomic_flag*>(__f));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_flag_clear_explicit(volatile atomic_flag* __f, memory_order __o)
|
||
{
|
||
__atomic_store(&__f->__flg_, __atomic_flag__(false), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_flag_clear_explicit(atomic_flag* __f, memory_order __o)
|
||
{
|
||
atomic_flag_clear_explicit(const_cast<volatile atomic_flag*>(__f), __o);
|
||
}
|
||
|
||
#define ATOMIC_FLAG_INIT {false}
|
||
#define ATOMIC_VAR_INIT(__v) {__v}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
memory_order
|
||
__translate_memory_order(memory_order __o)
|
||
{
|
||
switch (__o)
|
||
{
|
||
case memory_order_acq_rel:
|
||
return memory_order_acquire;
|
||
case memory_order_release:
|
||
return memory_order_relaxed;
|
||
}
|
||
return __o;
|
||
}
|
||
|
||
// atomic_bool
|
||
|
||
struct atomic_bool;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_bool*);
|
||
bool atomic_is_lock_free(const atomic_bool*);
|
||
void atomic_init(volatile atomic_bool*, bool);
|
||
void atomic_init(atomic_bool*, bool);
|
||
void atomic_store(volatile atomic_bool*, bool);
|
||
void atomic_store(atomic_bool*, bool);
|
||
void atomic_store_explicit(volatile atomic_bool*, bool, memory_order);
|
||
void atomic_store_explicit(atomic_bool*, bool, memory_order);
|
||
bool atomic_load(const volatile atomic_bool*);
|
||
bool atomic_load(const atomic_bool*);
|
||
bool atomic_load_explicit(const volatile atomic_bool*, memory_order);
|
||
bool atomic_load_explicit(const atomic_bool*, memory_order);
|
||
bool atomic_exchange(volatile atomic_bool*, bool);
|
||
bool atomic_exchange(atomic_bool*, bool);
|
||
bool atomic_exchange_explicit(volatile atomic_bool*, bool, memory_order);
|
||
bool atomic_exchange_explicit(atomic_bool*, bool, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_bool*, bool*, bool);
|
||
bool atomic_compare_exchange_weak(atomic_bool*, bool*, bool);
|
||
bool atomic_compare_exchange_strong(volatile atomic_bool*, bool*, bool);
|
||
bool atomic_compare_exchange_strong(atomic_bool*, bool*, bool);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_bool*, bool*, bool,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_bool*, bool*, bool,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_bool*, bool*, bool,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_bool*, bool*, bool,
|
||
memory_order, memory_order);
|
||
|
||
typedef struct atomic_bool
|
||
{
|
||
bool __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(bool __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(bool __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator bool() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator bool() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool exchange(bool __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool exchange(bool __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(bool& __v, bool __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(bool& __v, bool __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(bool& __v, bool __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(bool& __v, bool __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(bool& __v, bool __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(bool& __v, bool __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(bool& __v, bool __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(bool& __v, bool __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_bool() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_bool() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_bool(bool __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_bool(const atomic_bool&) = delete;
|
||
atomic_bool& operator=(const atomic_bool&) = delete;
|
||
atomic_bool& operator=(const atomic_bool&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_bool(const atomic_bool&);
|
||
atomic_bool& operator=(const atomic_bool&);
|
||
atomic_bool& operator=(const atomic_bool&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool operator=(bool __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool operator=(bool __v)
|
||
{store(__v); return __v;}
|
||
} atomic_bool;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_is_lock_free(const volatile atomic_bool*)
|
||
{
|
||
typedef bool type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_is_lock_free(const atomic_bool* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<const volatile atomic_bool*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_init(volatile atomic_bool* __obj, bool __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_init(atomic_bool* __obj, bool __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_store(volatile atomic_bool* __obj, bool __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_store(atomic_bool* __obj, bool __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_bool*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_store_explicit(volatile atomic_bool* __obj, bool __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void atomic_store_explicit(atomic_bool* __obj, bool __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_bool*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_load(const volatile atomic_bool* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_load(const atomic_bool* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_bool*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_load_explicit(const volatile atomic_bool* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_load_explicit(const atomic_bool* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_bool*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_exchange(volatile atomic_bool* __obj, bool __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_exchange(atomic_bool* __obj, bool __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_bool*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_exchange_explicit(volatile atomic_bool* __obj, bool __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_exchange_explicit(atomic_bool* __obj, bool __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_bool*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_weak(volatile atomic_bool* __obj, bool* __exp,
|
||
bool __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_weak(atomic_bool* __obj, bool* __exp, bool __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_bool*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_bool* __obj,
|
||
bool* __exp, bool __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_weak_explicit(atomic_bool* __obj, bool* __exp,
|
||
bool __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_bool*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_strong(volatile atomic_bool* __obj, bool* __exp,
|
||
bool __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_strong(atomic_bool* __obj, bool* __exp,
|
||
bool __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_bool*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_bool* __obj,
|
||
bool* __exp, bool __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool atomic_compare_exchange_strong_explicit(atomic_bool* __obj, bool* __exp,
|
||
bool __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_bool*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
// atomic_char
|
||
|
||
struct atomic_char;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_char*);
|
||
bool atomic_is_lock_free(const atomic_char*);
|
||
void atomic_init(volatile atomic_char*, char);
|
||
void atomic_init(atomic_char*, char);
|
||
void atomic_store(volatile atomic_char*, char);
|
||
void atomic_store(atomic_char*, char);
|
||
void atomic_store_explicit(volatile atomic_char*, char, memory_order);
|
||
void atomic_store_explicit(atomic_char*, char, memory_order);
|
||
char atomic_load(const volatile atomic_char*);
|
||
char atomic_load(const atomic_char*);
|
||
char atomic_load_explicit(const volatile atomic_char*, memory_order);
|
||
char atomic_load_explicit(const atomic_char*, memory_order);
|
||
char atomic_exchange(volatile atomic_char*, char);
|
||
char atomic_exchange(atomic_char*, char);
|
||
char atomic_exchange_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_exchange_explicit(atomic_char*, char, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_char*, char*, char);
|
||
bool atomic_compare_exchange_weak(atomic_char*, char*, char);
|
||
bool atomic_compare_exchange_strong(volatile atomic_char*, char*, char);
|
||
bool atomic_compare_exchange_strong(atomic_char*, char*, char);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_char*, char*, char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_char*, char*, char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_char*, char*, char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_char*, char*, char,
|
||
memory_order, memory_order);
|
||
char atomic_fetch_add(volatile atomic_char*, char);
|
||
char atomic_fetch_add(atomic_char*, char);
|
||
char atomic_fetch_add_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_fetch_add_explicit(atomic_char*, char, memory_order);
|
||
char atomic_fetch_sub(volatile atomic_char*, char);
|
||
char atomic_fetch_sub(atomic_char*, char);
|
||
char atomic_fetch_sub_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_fetch_sub_explicit(atomic_char*, char, memory_order);
|
||
char atomic_fetch_and(volatile atomic_char*, char);
|
||
char atomic_fetch_and(atomic_char*, char);
|
||
char atomic_fetch_and_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_fetch_and_explicit(atomic_char*, char, memory_order);
|
||
char atomic_fetch_or(volatile atomic_char*, char);
|
||
char atomic_fetch_or(atomic_char*, char);
|
||
char atomic_fetch_or_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_fetch_or_explicit(atomic_char*, char, memory_order);
|
||
char atomic_fetch_xor(volatile atomic_char*, char);
|
||
char atomic_fetch_xor(atomic_char*, char);
|
||
char atomic_fetch_xor_explicit(volatile atomic_char*, char, memory_order);
|
||
char atomic_fetch_xor_explicit(atomic_char*, char, memory_order);
|
||
|
||
typedef struct atomic_char
|
||
{
|
||
char __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char exchange(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char exchange(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char& __v, char __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char& __v, char __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char& __v, char __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char& __v, char __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char& __v, char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char& __v, char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char& __v, char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char& __v, char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_add(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_add(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_sub(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_sub(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_and(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_and(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_or(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_or(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_xor(char __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char fetch_xor(char __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_char() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_char() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_char(char __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_char(const atomic_char&) = delete;
|
||
atomic_char& operator=(const atomic_char&) = delete;
|
||
atomic_char& operator=(const atomic_char&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_char(const atomic_char&);
|
||
atomic_char& operator=(const atomic_char&);
|
||
atomic_char& operator=(const atomic_char&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator=(char __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator=(char __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++(int) volatile
|
||
{return fetch_add(char(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++(int)
|
||
{return fetch_add(char(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int) volatile
|
||
{return fetch_sub(char(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int)
|
||
{return fetch_sub(char(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++() volatile
|
||
{return char(fetch_add(char(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++()
|
||
{return char(fetch_add(char(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--() volatile
|
||
{return char(fetch_sub(char(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--()
|
||
{return char(fetch_sub(char(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v) volatile
|
||
{return char(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v)
|
||
{return char(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v) volatile
|
||
{return char(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v)
|
||
{return char(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v) volatile
|
||
{return char(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v)
|
||
{return char(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v) volatile
|
||
{return char(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v)
|
||
{return char(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v) volatile
|
||
{return char(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v)
|
||
{return char(fetch_xor(__v) ^ __v);}
|
||
} atomic_char;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_char*)
|
||
{
|
||
typedef char type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_char* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_char*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_char* __obj, char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_char* __obj, char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_char* __obj, char __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_char* __obj, char __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_char*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_char* __obj, char __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_char* __obj, char __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_char*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_load(const volatile atomic_char* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_load(const atomic_char* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_char*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_load_explicit(const volatile atomic_char* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_load_explicit(const atomic_char* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_char*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_exchange(volatile atomic_char* __obj, char __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_exchange(atomic_char* __obj, char __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_char*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_exchange_explicit(volatile atomic_char* __obj, char __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_exchange_explicit(atomic_char* __obj, char __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_char*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_char* __obj, char* __exp,
|
||
char __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_char* __obj, char* __exp, char __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_char*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_char* __obj, char* __exp,
|
||
char __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_char* __obj, char* __exp, char __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_char*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_char* __obj, char* __exp,
|
||
char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_char* __obj, char* __exp,
|
||
char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_char*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_char* __obj,
|
||
char* __exp, char __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_char* __obj, char* __exp,
|
||
char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_char*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_add(volatile atomic_char* __obj, char __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_add(atomic_char* __obj, char __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_char*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_add_explicit(volatile atomic_char* __obj, char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_add_explicit(atomic_char* __obj, char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_char*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_sub(volatile atomic_char* __obj, char __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_sub(atomic_char* __obj, char __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_char*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_sub_explicit(volatile atomic_char* __obj, char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_sub_explicit(atomic_char* __obj, char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_char*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_and(volatile atomic_char* __obj, char __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_and(atomic_char* __obj, char __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_char*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_and_explicit(volatile atomic_char* __obj, char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_and_explicit(atomic_char* __obj, char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_char*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_or(volatile atomic_char* __obj, char __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_or(atomic_char* __obj, char __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_char*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_or_explicit(volatile atomic_char* __obj, char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_or_explicit(atomic_char* __obj, char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_char*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_xor(volatile atomic_char* __obj, char __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_xor(atomic_char* __obj, char __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_char*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_xor_explicit(volatile atomic_char* __obj, char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char
|
||
atomic_fetch_xor_explicit(atomic_char* __obj, char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_char*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_schar
|
||
|
||
struct atomic_schar;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_schar*);
|
||
bool atomic_is_lock_free(const atomic_schar*);
|
||
void atomic_init(volatile atomic_schar*, signed char);
|
||
void atomic_init(atomic_schar*, signed char);
|
||
void atomic_store(volatile atomic_schar*, signed char);
|
||
void atomic_store(atomic_schar*, signed char);
|
||
void atomic_store_explicit(volatile atomic_schar*, signed char, memory_order);
|
||
void atomic_store_explicit(atomic_schar*, signed char, memory_order);
|
||
signed char atomic_load(const volatile atomic_schar*);
|
||
signed char atomic_load(const atomic_schar*);
|
||
signed char atomic_load_explicit(const volatile atomic_schar*, memory_order);
|
||
signed char atomic_load_explicit(const atomic_schar*, memory_order);
|
||
signed char atomic_exchange(volatile atomic_schar*, signed char);
|
||
signed char atomic_exchange(atomic_schar*, signed char);
|
||
signed char atomic_exchange_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_exchange_explicit(atomic_schar*, signed char, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_schar*, signed char*,
|
||
signed char);
|
||
bool atomic_compare_exchange_weak(atomic_schar*, signed char*, signed char);
|
||
bool atomic_compare_exchange_strong(volatile atomic_schar*, signed char*,
|
||
signed char);
|
||
bool atomic_compare_exchange_strong(atomic_schar*, signed char*, signed char);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_schar*, signed char*,
|
||
signed char, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_schar*, signed char*,
|
||
signed char, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_schar*,
|
||
signed char*, signed char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_schar*, signed char*,
|
||
signed char, memory_order,
|
||
memory_order);
|
||
signed char atomic_fetch_add(volatile atomic_schar*, signed char);
|
||
signed char atomic_fetch_add(atomic_schar*, signed char);
|
||
signed char atomic_fetch_add_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_fetch_add_explicit(atomic_schar*, signed char, memory_order);
|
||
signed char atomic_fetch_sub(volatile atomic_schar*, signed char);
|
||
signed char atomic_fetch_sub(atomic_schar*, signed char);
|
||
signed char atomic_fetch_sub_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_fetch_sub_explicit(atomic_schar*, signed char, memory_order);
|
||
signed char atomic_fetch_and(volatile atomic_schar*, signed char);
|
||
signed char atomic_fetch_and(atomic_schar*, signed char);
|
||
signed char atomic_fetch_and_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_fetch_and_explicit(atomic_schar*, signed char, memory_order);
|
||
signed char atomic_fetch_or(volatile atomic_schar*, signed char);
|
||
signed char atomic_fetch_or(atomic_schar*, signed char);
|
||
signed char atomic_fetch_or_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_fetch_or_explicit(atomic_schar*, signed char, memory_order);
|
||
signed char atomic_fetch_xor(volatile atomic_schar*, signed char);
|
||
signed char atomic_fetch_xor(atomic_schar*, signed char);
|
||
signed char atomic_fetch_xor_explicit(volatile atomic_schar*, signed char,
|
||
memory_order);
|
||
signed char atomic_fetch_xor_explicit(atomic_schar*, signed char, memory_order);
|
||
|
||
typedef struct atomic_schar
|
||
{
|
||
signed char __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(signed char __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator signed char() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator signed char() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char exchange(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char exchange(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(signed char& __v, signed char __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(signed char& __v, signed char __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(signed char& __v, signed char __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(signed char& __v, signed char __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(signed char& __v, signed char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(signed char& __v, signed char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(signed char& __v, signed char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(signed char& __v, signed char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_add(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_add(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_sub(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_sub(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_and(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_and(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_or(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_or(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_xor(signed char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char fetch_xor(signed char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_schar() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_schar() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_schar(signed char __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_schar(const atomic_schar&) = delete;
|
||
atomic_schar& operator=(const atomic_schar&) = delete;
|
||
atomic_schar& operator=(const atomic_schar&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_schar(const atomic_schar&);
|
||
atomic_schar& operator=(const atomic_schar&);
|
||
atomic_schar& operator=(const atomic_schar&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char operator=(signed char __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char operator=(signed char __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
signed char operator++(int) volatile
|
||
{typedef signed char type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++(int)
|
||
{typedef signed char type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int) volatile
|
||
{typedef signed char type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int)
|
||
{typedef signed char type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++() volatile
|
||
{typedef signed char type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++()
|
||
{typedef signed char type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--() volatile
|
||
{typedef signed char type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--()
|
||
{typedef signed char type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v) volatile
|
||
{typedef signed char type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v)
|
||
{typedef signed char type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v) volatile
|
||
{typedef signed char type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v)
|
||
{typedef signed char type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v) volatile
|
||
{typedef signed char type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v)
|
||
{typedef signed char type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v) volatile
|
||
{typedef signed char type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v)
|
||
{typedef signed char type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v) volatile
|
||
{typedef signed char type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v)
|
||
{typedef signed char type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_schar;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_schar*)
|
||
{
|
||
typedef signed char type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_schar* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_schar*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_schar* __obj, signed char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_schar* __obj, signed char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_schar* __obj, signed char __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_schar* __obj, signed char __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_schar*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_schar* __obj, signed char __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_schar* __obj, signed char __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_schar*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_load(const volatile atomic_schar* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_load(const atomic_schar* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_schar*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_load_explicit(const volatile atomic_schar* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_load_explicit(const atomic_schar* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_schar*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_exchange(volatile atomic_schar* __obj, signed char __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_exchange(atomic_schar* __obj, signed char __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_schar*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_exchange_explicit(volatile atomic_schar* __obj, signed char __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_exchange_explicit(atomic_schar* __obj, signed char __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_schar*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_schar*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_schar*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_schar* __obj,
|
||
signed char* __exp, signed char __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_schar*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_schar* __obj,
|
||
signed char* __exp, signed char __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_schar* __obj, signed char* __exp,
|
||
signed char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_schar*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_add(volatile atomic_schar* __obj, signed char __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_add(atomic_schar* __obj, signed char __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_schar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_add_explicit(volatile atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_add_explicit(atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_schar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_sub(volatile atomic_schar* __obj, signed char __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_sub(atomic_schar* __obj, signed char __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_schar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_sub_explicit(volatile atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_sub_explicit(atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_schar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_and(volatile atomic_schar* __obj, signed char __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_and(atomic_schar* __obj, signed char __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_schar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_and_explicit(volatile atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_and_explicit(atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_schar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_or(volatile atomic_schar* __obj, signed char __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_or(atomic_schar* __obj, signed char __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_schar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_or_explicit(volatile atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_or_explicit(atomic_schar* __obj, signed char __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_schar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_xor(volatile atomic_schar* __obj, signed char __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_xor(atomic_schar* __obj, signed char __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_schar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_xor_explicit(volatile atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
signed char
|
||
atomic_fetch_xor_explicit(atomic_schar* __obj, signed char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_schar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_uchar
|
||
|
||
struct atomic_uchar;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_uchar*);
|
||
bool atomic_is_lock_free(const atomic_uchar*);
|
||
void atomic_init(volatile atomic_uchar*, unsigned char);
|
||
void atomic_init(atomic_uchar*, unsigned char);
|
||
void atomic_store(volatile atomic_uchar*, unsigned char);
|
||
void atomic_store(atomic_uchar*, unsigned char);
|
||
void atomic_store_explicit(volatile atomic_uchar*, unsigned char, memory_order);
|
||
void atomic_store_explicit(atomic_uchar*, unsigned char, memory_order);
|
||
unsigned char atomic_load(const volatile atomic_uchar*);
|
||
unsigned char atomic_load(const atomic_uchar*);
|
||
unsigned char atomic_load_explicit(const volatile atomic_uchar*, memory_order);
|
||
unsigned char atomic_load_explicit(const atomic_uchar*, memory_order);
|
||
unsigned char atomic_exchange(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_exchange(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_exchange_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_exchange_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_uchar*, unsigned char*,
|
||
unsigned char);
|
||
bool atomic_compare_exchange_weak(atomic_uchar*, unsigned char*, unsigned char);
|
||
bool atomic_compare_exchange_strong(volatile atomic_uchar*, unsigned char*,
|
||
unsigned char);
|
||
bool atomic_compare_exchange_strong(atomic_uchar*, unsigned char*,
|
||
unsigned char);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_uchar*,
|
||
unsigned char*, unsigned char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_uchar*, unsigned char*,
|
||
unsigned char, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_uchar*,
|
||
unsigned char*, unsigned char,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_uchar*, unsigned char*,
|
||
unsigned char, memory_order,
|
||
memory_order);
|
||
unsigned char atomic_fetch_add(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_add(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_add_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_add_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_sub(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_sub(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_sub_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_sub_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_and(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_and(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_and_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_and_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_or(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_or(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_or_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_or_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_xor(volatile atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_xor(atomic_uchar*, unsigned char);
|
||
unsigned char atomic_fetch_xor_explicit(volatile atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
unsigned char atomic_fetch_xor_explicit(atomic_uchar*, unsigned char,
|
||
memory_order);
|
||
|
||
typedef struct atomic_uchar
|
||
{
|
||
unsigned char __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned char __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned char() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned char() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char exchange(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char exchange(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned char& __v, unsigned char __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned char& __v, unsigned char __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned char& __v, unsigned char __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned char& __v, unsigned char __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned char& __v, unsigned char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned char& __v, unsigned char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned char& __v, unsigned char __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned char& __v, unsigned char __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_add(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_add(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_sub(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_sub(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_and(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_and(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_or(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_or(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_xor(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char fetch_xor(unsigned char __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_uchar() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_uchar() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_uchar(unsigned char __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_uchar(const atomic_uchar&) = delete;
|
||
atomic_uchar& operator=(const atomic_uchar&) = delete;
|
||
atomic_uchar& operator=(const atomic_uchar&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_uchar(const atomic_uchar&);
|
||
atomic_uchar& operator=(const atomic_uchar&);
|
||
atomic_uchar& operator=(const atomic_uchar&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char operator=(unsigned char __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char operator=(unsigned char __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned char operator++(int) volatile
|
||
{typedef unsigned char type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++(int)
|
||
{typedef unsigned char type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int) volatile
|
||
{typedef unsigned char type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--(int)
|
||
{typedef unsigned char type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++() volatile
|
||
{typedef unsigned char type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator++()
|
||
{typedef unsigned char type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--() volatile
|
||
{typedef unsigned char type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator--()
|
||
{typedef unsigned char type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v) volatile
|
||
{typedef unsigned char type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator+=(char __v)
|
||
{typedef unsigned char type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v) volatile
|
||
{typedef unsigned char type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator-=(char __v)
|
||
{typedef unsigned char type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v) volatile
|
||
{typedef unsigned char type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator&=(char __v)
|
||
{typedef unsigned char type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v) volatile
|
||
{typedef unsigned char type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator|=(char __v)
|
||
{typedef unsigned char type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v) volatile
|
||
{typedef unsigned char type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char operator^=(char __v)
|
||
{typedef unsigned char type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_uchar;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_uchar*)
|
||
{
|
||
typedef unsigned char type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_uchar* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_uchar*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_uchar*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_uchar* __obj, unsigned char __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_uchar* __obj, unsigned char __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_uchar*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_load(const volatile atomic_uchar* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_load(const atomic_uchar* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_uchar*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_load_explicit(const volatile atomic_uchar* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_load_explicit(const atomic_uchar* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_uchar*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_exchange(volatile atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_exchange(atomic_uchar* __obj, unsigned char __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_uchar*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_exchange_explicit(volatile atomic_uchar* __obj, unsigned char __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_exchange_explicit(atomic_uchar* __obj, unsigned char __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_uchar*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_uchar* __obj, unsigned char* __exp,
|
||
unsigned char __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_uchar* __obj, unsigned char* __exp,
|
||
unsigned char __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_uchar*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_uchar* __obj,
|
||
unsigned char* __exp, unsigned char __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_uchar* __obj, unsigned char* __exp,
|
||
unsigned char __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_uchar*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_uchar* __obj,
|
||
unsigned char* __exp,
|
||
unsigned char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_uchar* __obj, unsigned char* __exp,
|
||
unsigned char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_uchar*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_uchar* __obj,
|
||
unsigned char* __exp,
|
||
unsigned char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_uchar* __obj,
|
||
unsigned char* __exp,
|
||
unsigned char __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_uchar*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_add(volatile atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_add(atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_uchar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_add_explicit(volatile atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_add_explicit(atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_uchar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_sub(volatile atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_sub(atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_uchar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_sub_explicit(volatile atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_sub_explicit(atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_uchar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_and(volatile atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_and(atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_uchar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_and_explicit(volatile atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_and_explicit(atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_uchar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_or(volatile atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_or(atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_uchar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_or_explicit(volatile atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_or_explicit(atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_uchar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_xor(volatile atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_xor(atomic_uchar* __obj, unsigned char __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_uchar*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_xor_explicit(volatile atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned char
|
||
atomic_fetch_xor_explicit(atomic_uchar* __obj, unsigned char __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_uchar*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_short
|
||
|
||
struct atomic_short;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_short*);
|
||
bool atomic_is_lock_free(const atomic_short*);
|
||
void atomic_init(volatile atomic_short*, short);
|
||
void atomic_init(atomic_short*, short);
|
||
void atomic_store(volatile atomic_short*, short);
|
||
void atomic_store(atomic_short*, short);
|
||
void atomic_store_explicit(volatile atomic_short*, short, memory_order);
|
||
void atomic_store_explicit(atomic_short*, short, memory_order);
|
||
short atomic_load(const volatile atomic_short*);
|
||
short atomic_load(const atomic_short*);
|
||
short atomic_load_explicit(const volatile atomic_short*, memory_order);
|
||
short atomic_load_explicit(const atomic_short*, memory_order);
|
||
short atomic_exchange(volatile atomic_short*, short);
|
||
short atomic_exchange(atomic_short*, short);
|
||
short atomic_exchange_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_exchange_explicit(atomic_short*, short, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_short*, short*, short);
|
||
bool atomic_compare_exchange_weak(atomic_short*, short*, short);
|
||
bool atomic_compare_exchange_strong(volatile atomic_short*, short*, short);
|
||
bool atomic_compare_exchange_strong(atomic_short*, short*, short);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_short*, short*,
|
||
short, memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_short*, short*, short,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_short*, short*,
|
||
short, memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_short*, short*, short,
|
||
memory_order, memory_order);
|
||
short atomic_fetch_add(volatile atomic_short*, short);
|
||
short atomic_fetch_add(atomic_short*, short);
|
||
short atomic_fetch_add_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_fetch_add_explicit(atomic_short*, short, memory_order);
|
||
short atomic_fetch_sub(volatile atomic_short*, short);
|
||
short atomic_fetch_sub(atomic_short*, short);
|
||
short atomic_fetch_sub_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_fetch_sub_explicit(atomic_short*, short, memory_order);
|
||
short atomic_fetch_and(volatile atomic_short*, short);
|
||
short atomic_fetch_and(atomic_short*, short);
|
||
short atomic_fetch_and_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_fetch_and_explicit(atomic_short*, short, memory_order);
|
||
short atomic_fetch_or(volatile atomic_short*, short);
|
||
short atomic_fetch_or(atomic_short*, short);
|
||
short atomic_fetch_or_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_fetch_or_explicit(atomic_short*, short, memory_order);
|
||
short atomic_fetch_xor(volatile atomic_short*, short);
|
||
short atomic_fetch_xor(atomic_short*, short);
|
||
short atomic_fetch_xor_explicit(volatile atomic_short*, short, memory_order);
|
||
short atomic_fetch_xor_explicit(atomic_short*, short, memory_order);
|
||
|
||
typedef struct atomic_short
|
||
{
|
||
short __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(short __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator short() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator short() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short exchange(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short exchange(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(short& __v, short __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(short& __v, short __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(short& __v, short __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(short& __v, short __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(short& __v, short __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(short& __v, short __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(short& __v, short __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(short& __v, short __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_add(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_add(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_sub(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_sub(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_and(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_and(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_or(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_or(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_xor(short __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short fetch_xor(short __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_short() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_short() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_short(short __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_short(const atomic_short&) = delete;
|
||
atomic_short& operator=(const atomic_short&) = delete;
|
||
atomic_short& operator=(const atomic_short&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_short(const atomic_short&);
|
||
atomic_short& operator=(const atomic_short&);
|
||
atomic_short& operator=(const atomic_short&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator=(short __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator=(short __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++(int) volatile
|
||
{return fetch_add(short(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++(int)
|
||
{return fetch_add(short(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--(int) volatile
|
||
{return fetch_sub(short(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--(int)
|
||
{return fetch_sub(short(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++() volatile
|
||
{return short(fetch_add(short(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++()
|
||
{return short(fetch_add(short(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--() volatile
|
||
{return short(fetch_sub(short(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--()
|
||
{return short(fetch_sub(short(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator+=(short __v) volatile
|
||
{return short(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator+=(short __v)
|
||
{return short(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator-=(short __v) volatile
|
||
{return short(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator-=(short __v)
|
||
{return short(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator&=(short __v) volatile
|
||
{return short(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator&=(short __v)
|
||
{return short(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator|=(short __v) volatile
|
||
{return short(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator|=(short __v)
|
||
{return short(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator^=(short __v) volatile
|
||
{return short(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator^=(short __v)
|
||
{return short(fetch_xor(__v) ^ __v);}
|
||
} atomic_short;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_short*)
|
||
{
|
||
typedef short type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_short* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_short*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_short* __obj, short __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_short* __obj, short __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_short* __obj, short __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_short* __obj, short __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_short*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_short* __obj, short __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_short* __obj, short __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_short*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_load(const volatile atomic_short* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_load(const atomic_short* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_short*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_load_explicit(const volatile atomic_short* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_load_explicit(const atomic_short* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_short*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_exchange(volatile atomic_short* __obj, short __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_exchange(atomic_short* __obj, short __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_short*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_exchange_explicit(volatile atomic_short* __obj, short __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_exchange_explicit(atomic_short* __obj, short __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_short*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_short* __obj, short* __exp,
|
||
short __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_short* __obj, short* __exp, short __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_short*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_short* __obj, short* __exp,
|
||
short __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_short* __obj, short* __exp, short __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_short*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_short* __obj,
|
||
short* __exp, short __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_short* __obj, short* __exp,
|
||
short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_short*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_short* __obj,
|
||
short* __exp, short __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_short* __obj, short* __exp,
|
||
short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_short*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_add(volatile atomic_short* __obj, short __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_add(atomic_short* __obj, short __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_short*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_add_explicit(volatile atomic_short* __obj, short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_add_explicit(atomic_short* __obj, short __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_short*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_sub(volatile atomic_short* __obj, short __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_sub(atomic_short* __obj, short __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_short*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_sub_explicit(volatile atomic_short* __obj, short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_sub_explicit(atomic_short* __obj, short __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_short*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_and(volatile atomic_short* __obj, short __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_and(atomic_short* __obj, short __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_short*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_and_explicit(volatile atomic_short* __obj, short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_and_explicit(atomic_short* __obj, short __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_short*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_or(volatile atomic_short* __obj, short __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_or(atomic_short* __obj, short __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_short*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_or_explicit(volatile atomic_short* __obj, short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_or_explicit(atomic_short* __obj, short __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_short*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_xor(volatile atomic_short* __obj, short __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_xor(atomic_short* __obj, short __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_short*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_xor_explicit(volatile atomic_short* __obj, short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
short
|
||
atomic_fetch_xor_explicit(atomic_short* __obj, short __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_short*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_ushort
|
||
|
||
struct atomic_ushort;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_ushort*);
|
||
bool atomic_is_lock_free(const atomic_ushort*);
|
||
void atomic_init(volatile atomic_ushort*, unsigned short);
|
||
void atomic_init(atomic_ushort*, unsigned short);
|
||
void atomic_store(volatile atomic_ushort*, unsigned short);
|
||
void atomic_store(atomic_ushort*, unsigned short);
|
||
void atomic_store_explicit(volatile atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
void atomic_store_explicit(atomic_ushort*, unsigned short, memory_order);
|
||
unsigned short atomic_load(const volatile atomic_ushort*);
|
||
unsigned short atomic_load(const atomic_ushort*);
|
||
unsigned short atomic_load_explicit(const volatile atomic_ushort*,
|
||
memory_order);
|
||
unsigned short atomic_load_explicit(const atomic_ushort*, memory_order);
|
||
unsigned short atomic_exchange(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_exchange(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_exchange_explicit(volatile atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_exchange_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_ushort*, unsigned short*,
|
||
unsigned short);
|
||
bool atomic_compare_exchange_weak(atomic_ushort*, unsigned short*,
|
||
unsigned short);
|
||
bool atomic_compare_exchange_strong(volatile atomic_ushort*, unsigned short*,
|
||
unsigned short);
|
||
bool atomic_compare_exchange_strong(atomic_ushort*, unsigned short*,
|
||
unsigned short);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_ushort*,
|
||
unsigned short*, unsigned short,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_ushort*, unsigned short*,
|
||
unsigned short, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_ushort*,
|
||
unsigned short*, unsigned short,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_ushort*, unsigned short*,
|
||
unsigned short, memory_order,
|
||
memory_order);
|
||
unsigned short atomic_fetch_add(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_add(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_add_explicit(volatile atomic_ushort*,
|
||
unsigned short, memory_order);
|
||
unsigned short atomic_fetch_add_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_fetch_sub(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_sub(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_sub_explicit(volatile atomic_ushort*,
|
||
unsigned short, memory_order);
|
||
unsigned short atomic_fetch_sub_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_fetch_and(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_and(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_and_explicit(volatile atomic_ushort*,
|
||
unsigned short, memory_order);
|
||
unsigned short atomic_fetch_and_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_fetch_or(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_or(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_or_explicit(volatile atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_fetch_or_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
unsigned short atomic_fetch_xor(volatile atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_xor(atomic_ushort*, unsigned short);
|
||
unsigned short atomic_fetch_xor_explicit(volatile atomic_ushort*,
|
||
unsigned short, memory_order);
|
||
unsigned short atomic_fetch_xor_explicit(atomic_ushort*, unsigned short,
|
||
memory_order);
|
||
|
||
typedef struct atomic_ushort
|
||
{
|
||
unsigned short __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned short __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned short() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned short() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short exchange(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short exchange(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned short& __v, unsigned short __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned short& __v, unsigned short __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned short& __v, unsigned short __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned short& __v, unsigned short __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned short& __v, unsigned short __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned short& __v, unsigned short __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned short& __v, unsigned short __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned short& __v, unsigned short __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_add(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_add(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_sub(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_sub(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_and(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_and(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_or(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_or(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_xor(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short fetch_xor(unsigned short __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_ushort() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_ushort() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_ushort(unsigned short __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_ushort(const atomic_ushort&) = delete;
|
||
atomic_ushort& operator=(const atomic_ushort&) = delete;
|
||
atomic_ushort& operator=(const atomic_ushort&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_ushort(const atomic_ushort&);
|
||
atomic_ushort& operator=(const atomic_ushort&);
|
||
atomic_ushort& operator=(const atomic_ushort&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short operator=(unsigned short __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short operator=(unsigned short __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned short operator++(int) volatile
|
||
{typedef unsigned short type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++(int)
|
||
{typedef unsigned short type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--(int) volatile
|
||
{typedef unsigned short type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--(int)
|
||
{typedef unsigned short type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++() volatile
|
||
{typedef unsigned short type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator++()
|
||
{typedef unsigned short type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--() volatile
|
||
{typedef unsigned short type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator--()
|
||
{typedef unsigned short type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator+=(short __v) volatile
|
||
{typedef unsigned short type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator+=(short __v)
|
||
{typedef unsigned short type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator-=(short __v) volatile
|
||
{typedef unsigned short type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator-=(short __v)
|
||
{typedef unsigned short type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator&=(short __v) volatile
|
||
{typedef unsigned short type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator&=(short __v)
|
||
{typedef unsigned short type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator|=(short __v) volatile
|
||
{typedef unsigned short type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator|=(short __v)
|
||
{typedef unsigned short type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator^=(short __v) volatile
|
||
{typedef unsigned short type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
short operator^=(short __v)
|
||
{typedef unsigned short type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_ushort;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_ushort*)
|
||
{
|
||
typedef unsigned short type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_ushort* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_ushort*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_ushort*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_ushort* __obj, unsigned short __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_ushort* __obj, unsigned short __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_ushort*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_load(const volatile atomic_ushort* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_load(const atomic_ushort* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_ushort*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_load_explicit(const volatile atomic_ushort* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_load_explicit(const atomic_ushort* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_ushort*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_exchange(volatile atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_exchange(atomic_ushort* __obj, unsigned short __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_ushort*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_exchange_explicit(volatile atomic_ushort* __obj, unsigned short __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_exchange_explicit(atomic_ushort* __obj, unsigned short __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_ushort*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_ushort* __obj,
|
||
unsigned short* __exp, unsigned short __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_ushort* __obj, unsigned short* __exp,
|
||
unsigned short __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_ushort*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_ushort* __obj,
|
||
unsigned short* __exp, unsigned short __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_ushort* __obj, unsigned short* __exp,
|
||
unsigned short __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_ushort*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_ushort* __obj,
|
||
unsigned short* __exp,
|
||
unsigned short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_ushort* __obj,
|
||
unsigned short* __exp,
|
||
unsigned short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_ushort*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_ushort* __obj,
|
||
unsigned short* __exp,
|
||
unsigned short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_ushort* __obj,
|
||
unsigned short* __exp,
|
||
unsigned short __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_ushort*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_add(volatile atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_add(atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_ushort*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_add_explicit(volatile atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_add_explicit(atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_ushort*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_sub(volatile atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_sub(atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_ushort*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_sub_explicit(volatile atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_sub_explicit(atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_ushort*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_and(volatile atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_and(atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_ushort*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_and_explicit(volatile atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_and_explicit(atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_ushort*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_or(volatile atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_or(atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_ushort*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_or_explicit(volatile atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_or_explicit(atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_ushort*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_xor(volatile atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_xor(atomic_ushort* __obj, unsigned short __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_ushort*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_xor_explicit(volatile atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned short
|
||
atomic_fetch_xor_explicit(atomic_ushort* __obj, unsigned short __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_ushort*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_int
|
||
|
||
struct atomic_int;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_int*);
|
||
bool atomic_is_lock_free(const atomic_int*);
|
||
void atomic_init(volatile atomic_int*, int);
|
||
void atomic_init(atomic_int*, int);
|
||
void atomic_store(volatile atomic_int*, int);
|
||
void atomic_store(atomic_int*, int);
|
||
void atomic_store_explicit(volatile atomic_int*, int, memory_order);
|
||
void atomic_store_explicit(atomic_int*, int, memory_order);
|
||
int atomic_load(const volatile atomic_int*);
|
||
int atomic_load(const atomic_int*);
|
||
int atomic_load_explicit(const volatile atomic_int*, memory_order);
|
||
int atomic_load_explicit(const atomic_int*, memory_order);
|
||
int atomic_exchange(volatile atomic_int*, int);
|
||
int atomic_exchange(atomic_int*, int);
|
||
int atomic_exchange_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_exchange_explicit(atomic_int*, int, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_int*, int*, int);
|
||
bool atomic_compare_exchange_weak(atomic_int*, int*, int);
|
||
bool atomic_compare_exchange_strong(volatile atomic_int*, int*, int);
|
||
bool atomic_compare_exchange_strong(atomic_int*, int*, int);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_int*, int*, int,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_int*, int*, int,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_int*, int*, int,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_int*, int*, int,
|
||
memory_order, memory_order);
|
||
int atomic_fetch_add(volatile atomic_int*, int);
|
||
int atomic_fetch_add(atomic_int*, int);
|
||
int atomic_fetch_add_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_fetch_add_explicit(atomic_int*, int, memory_order);
|
||
int atomic_fetch_sub(volatile atomic_int*, int);
|
||
int atomic_fetch_sub(atomic_int*, int);
|
||
int atomic_fetch_sub_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_fetch_sub_explicit(atomic_int*, int, memory_order);
|
||
int atomic_fetch_and(volatile atomic_int*, int);
|
||
int atomic_fetch_and(atomic_int*, int);
|
||
int atomic_fetch_and_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_fetch_and_explicit(atomic_int*, int, memory_order);
|
||
int atomic_fetch_or(volatile atomic_int*, int);
|
||
int atomic_fetch_or(atomic_int*, int);
|
||
int atomic_fetch_or_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_fetch_or_explicit(atomic_int*, int, memory_order);
|
||
int atomic_fetch_xor(volatile atomic_int*, int);
|
||
int atomic_fetch_xor(atomic_int*, int);
|
||
int atomic_fetch_xor_explicit(volatile atomic_int*, int, memory_order);
|
||
int atomic_fetch_xor_explicit(atomic_int*, int, memory_order);
|
||
|
||
typedef struct atomic_int
|
||
{
|
||
int __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(int __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator int() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator int() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int exchange(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int exchange(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(int& __v, int __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(int& __v, int __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(int& __v, int __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(int& __v, int __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(int& __v, int __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(int& __v, int __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(int& __v, int __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(int& __v, int __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_add(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_add(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_sub(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_sub(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_and(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_and(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_or(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_or(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_xor(int __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int fetch_xor(int __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_int() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_int() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_int(int __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_int(const atomic_int&) = delete;
|
||
atomic_int& operator=(const atomic_int&) = delete;
|
||
atomic_int& operator=(const atomic_int&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_int(const atomic_int&);
|
||
atomic_int& operator=(const atomic_int&);
|
||
atomic_int& operator=(const atomic_int&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator=(int __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator=(int __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++(int) volatile
|
||
{return fetch_add(int(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++(int)
|
||
{return fetch_add(int(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--(int) volatile
|
||
{return fetch_sub(int(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--(int)
|
||
{return fetch_sub(int(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++() volatile
|
||
{return int(fetch_add(int(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++()
|
||
{return int(fetch_add(int(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--() volatile
|
||
{return int(fetch_sub(int(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--()
|
||
{return int(fetch_sub(int(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator+=(int __v) volatile
|
||
{return int(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator+=(int __v)
|
||
{return int(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator-=(int __v) volatile
|
||
{return int(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator-=(int __v)
|
||
{return int(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator&=(int __v) volatile
|
||
{return int(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator&=(int __v)
|
||
{return int(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator|=(int __v) volatile
|
||
{return int(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator|=(int __v)
|
||
{return int(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator^=(int __v) volatile
|
||
{return int(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator^=(int __v)
|
||
{return int(fetch_xor(__v) ^ __v);}
|
||
} atomic_int;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_int*)
|
||
{
|
||
typedef int type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_int* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_int*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_int* __obj, int __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_int* __obj, int __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_int* __obj, int __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_int* __obj, int __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_int*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_int* __obj, int __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_int* __obj, int __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_int*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_load(const volatile atomic_int* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_load(const atomic_int* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_int*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_load_explicit(const volatile atomic_int* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_load_explicit(const atomic_int* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_int*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_exchange(volatile atomic_int* __obj, int __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_exchange(atomic_int* __obj, int __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_int*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_exchange_explicit(volatile atomic_int* __obj, int __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_exchange_explicit(atomic_int* __obj, int __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_int*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_int* __obj, int* __exp,
|
||
int __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_int* __obj, int* __exp, int __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_int*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_int* __obj, int* __exp,
|
||
int __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_int* __obj, int* __exp, int __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_int*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_int* __obj, int* __exp,
|
||
int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_int* __obj, int* __exp,
|
||
int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_int*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_int* __obj,
|
||
int* __exp, int __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_int* __obj, int* __exp,
|
||
int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_int*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_add(volatile atomic_int* __obj, int __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_add(atomic_int* __obj, int __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_int*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_add_explicit(volatile atomic_int* __obj, int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_add_explicit(atomic_int* __obj, int __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_int*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_sub(volatile atomic_int* __obj, int __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_sub(atomic_int* __obj, int __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_int*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_sub_explicit(volatile atomic_int* __obj, int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_sub_explicit(atomic_int* __obj, int __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_int*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_and(volatile atomic_int* __obj, int __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_and(atomic_int* __obj, int __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_int*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_and_explicit(volatile atomic_int* __obj, int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_and_explicit(atomic_int* __obj, int __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_int*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_or(volatile atomic_int* __obj, int __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_or(atomic_int* __obj, int __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_int*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_or_explicit(volatile atomic_int* __obj, int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_or_explicit(atomic_int* __obj, int __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_int*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_xor(volatile atomic_int* __obj, int __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_xor(atomic_int* __obj, int __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_int*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_xor_explicit(volatile atomic_int* __obj, int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
int
|
||
atomic_fetch_xor_explicit(atomic_int* __obj, int __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_int*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_uint
|
||
|
||
struct atomic_uint;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_uint*);
|
||
bool atomic_is_lock_free(const atomic_uint*);
|
||
void atomic_init(volatile atomic_uint*, unsigned int);
|
||
void atomic_init(atomic_uint*, unsigned int);
|
||
void atomic_store(volatile atomic_uint*, unsigned int);
|
||
void atomic_store(atomic_uint*, unsigned int);
|
||
void atomic_store_explicit(volatile atomic_uint*, unsigned int, memory_order);
|
||
void atomic_store_explicit(atomic_uint*, unsigned int, memory_order);
|
||
unsigned int atomic_load(const volatile atomic_uint*);
|
||
unsigned int atomic_load(const atomic_uint*);
|
||
unsigned int atomic_load_explicit(const volatile atomic_uint*, memory_order);
|
||
unsigned int atomic_load_explicit(const atomic_uint*, memory_order);
|
||
unsigned int atomic_exchange(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_exchange(atomic_uint*, unsigned int);
|
||
unsigned int atomic_exchange_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_exchange_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_uint*, unsigned int*,
|
||
unsigned int);
|
||
bool atomic_compare_exchange_weak(atomic_uint*, unsigned int*, unsigned int);
|
||
bool atomic_compare_exchange_strong(volatile atomic_uint*, unsigned int*,
|
||
unsigned int);
|
||
bool atomic_compare_exchange_strong(atomic_uint*, unsigned int*,
|
||
unsigned int);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_uint*,
|
||
unsigned int*, unsigned int,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_uint*, unsigned int*,
|
||
unsigned int, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_uint*,
|
||
unsigned int*, unsigned int,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_uint*, unsigned int*,
|
||
unsigned int, memory_order,
|
||
memory_order);
|
||
unsigned int atomic_fetch_add(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_add(atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_add_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_add_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_sub(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_sub(atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_sub_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_sub_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_and(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_and(atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_and_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_and_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_or(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_or(atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_or_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_or_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_xor(volatile atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_xor(atomic_uint*, unsigned int);
|
||
unsigned int atomic_fetch_xor_explicit(volatile atomic_uint*, unsigned int,
|
||
memory_order);
|
||
unsigned int atomic_fetch_xor_explicit(atomic_uint*, unsigned int,
|
||
memory_order);
|
||
|
||
typedef struct atomic_uint
|
||
{
|
||
unsigned int __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned int __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned int() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned int() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int exchange(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int exchange(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned int& __v, unsigned int __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned int& __v, unsigned int __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned int& __v, unsigned int __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned int& __v, unsigned int __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned int& __v, unsigned int __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned int& __v, unsigned int __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned int& __v, unsigned int __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned int& __v, unsigned int __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_add(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_add(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_sub(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_sub(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_and(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_and(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_or(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_or(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_xor(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int fetch_xor(unsigned int __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_uint() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_uint() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_uint(unsigned int __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_uint(const atomic_uint&) = delete;
|
||
atomic_uint& operator=(const atomic_uint&) = delete;
|
||
atomic_uint& operator=(const atomic_uint&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_uint(const atomic_uint&);
|
||
atomic_uint& operator=(const atomic_uint&);
|
||
atomic_uint& operator=(const atomic_uint&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int operator=(unsigned int __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int operator=(unsigned int __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned int operator++(int) volatile
|
||
{typedef unsigned int type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++(int)
|
||
{typedef unsigned int type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--(int) volatile
|
||
{typedef unsigned int type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--(int)
|
||
{typedef unsigned int type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++() volatile
|
||
{typedef unsigned int type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator++()
|
||
{typedef unsigned int type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--() volatile
|
||
{typedef unsigned int type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator--()
|
||
{typedef unsigned int type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator+=(int __v) volatile
|
||
{typedef unsigned int type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator+=(int __v)
|
||
{typedef unsigned int type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator-=(int __v) volatile
|
||
{typedef unsigned int type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator-=(int __v)
|
||
{typedef unsigned int type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator&=(int __v) volatile
|
||
{typedef unsigned int type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator&=(int __v)
|
||
{typedef unsigned int type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator|=(int __v) volatile
|
||
{typedef unsigned int type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator|=(int __v)
|
||
{typedef unsigned int type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator^=(int __v) volatile
|
||
{typedef unsigned int type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
int operator^=(int __v)
|
||
{typedef unsigned int type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_uint;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_uint*)
|
||
{
|
||
typedef unsigned int type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_uint* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_uint*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_uint*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_uint* __obj, unsigned int __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_uint* __obj, unsigned int __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_uint*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_load(const volatile atomic_uint* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_load(const atomic_uint* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_uint*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_load_explicit(const volatile atomic_uint* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_load_explicit(const atomic_uint* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_uint*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_exchange(volatile atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_exchange(atomic_uint* __obj, unsigned int __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_uint*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_exchange_explicit(volatile atomic_uint* __obj, unsigned int __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_exchange_explicit(atomic_uint* __obj, unsigned int __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_uint*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_uint* __obj, unsigned int* __exp,
|
||
unsigned int __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_uint* __obj, unsigned int* __exp,
|
||
unsigned int __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_uint*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_uint* __obj,
|
||
unsigned int* __exp, unsigned int __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_uint* __obj, unsigned int* __exp,
|
||
unsigned int __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_uint*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_uint* __obj,
|
||
unsigned int* __exp,
|
||
unsigned int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_uint* __obj, unsigned int* __exp,
|
||
unsigned int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_uint*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_uint* __obj,
|
||
unsigned int* __exp,
|
||
unsigned int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_uint* __obj,
|
||
unsigned int* __exp,
|
||
unsigned int __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_uint*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_add(volatile atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_add(atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_uint*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_add_explicit(volatile atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_add_explicit(atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_uint*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_sub(volatile atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_sub(atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_uint*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_sub_explicit(volatile atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_sub_explicit(atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_uint*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_and(volatile atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_and(atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_uint*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_and_explicit(volatile atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_and_explicit(atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_uint*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_or(volatile atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_or(atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_uint*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_or_explicit(volatile atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_or_explicit(atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_uint*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_xor(volatile atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_xor(atomic_uint* __obj, unsigned int __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_uint*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_xor_explicit(volatile atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned int
|
||
atomic_fetch_xor_explicit(atomic_uint* __obj, unsigned int __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_uint*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_long
|
||
|
||
struct atomic_long;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_long*);
|
||
bool atomic_is_lock_free(const atomic_long*);
|
||
void atomic_init(volatile atomic_long*, long);
|
||
void atomic_init(atomic_long*, long);
|
||
void atomic_store(volatile atomic_long*, long);
|
||
void atomic_store(atomic_long*, long);
|
||
void atomic_store_explicit(volatile atomic_long*, long, memory_order);
|
||
void atomic_store_explicit(atomic_long*, long, memory_order);
|
||
long atomic_load(const volatile atomic_long*);
|
||
long atomic_load(const atomic_long*);
|
||
long atomic_load_explicit(const volatile atomic_long*, memory_order);
|
||
long atomic_load_explicit(const atomic_long*, memory_order);
|
||
long atomic_exchange(volatile atomic_long*, long);
|
||
long atomic_exchange(atomic_long*, long);
|
||
long atomic_exchange_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_exchange_explicit(atomic_long*, long, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_long*, long*, long);
|
||
bool atomic_compare_exchange_weak(atomic_long*, long*, long);
|
||
bool atomic_compare_exchange_strong(volatile atomic_long*, long*, long);
|
||
bool atomic_compare_exchange_strong(atomic_long*, long*, long);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_long*, long*, long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_long*, long*, long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_long*, long*, long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_long*, long*, long,
|
||
memory_order, memory_order);
|
||
long atomic_fetch_add(volatile atomic_long*, long);
|
||
long atomic_fetch_add(atomic_long*, long);
|
||
long atomic_fetch_add_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_fetch_add_explicit(atomic_long*, long, memory_order);
|
||
long atomic_fetch_sub(volatile atomic_long*, long);
|
||
long atomic_fetch_sub(atomic_long*, long);
|
||
long atomic_fetch_sub_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_fetch_sub_explicit(atomic_long*, long, memory_order);
|
||
long atomic_fetch_and(volatile atomic_long*, long);
|
||
long atomic_fetch_and(atomic_long*, long);
|
||
long atomic_fetch_and_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_fetch_and_explicit(atomic_long*, long, memory_order);
|
||
long atomic_fetch_or(volatile atomic_long*, long);
|
||
long atomic_fetch_or(atomic_long*, long);
|
||
long atomic_fetch_or_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_fetch_or_explicit(atomic_long*, long, memory_order);
|
||
long atomic_fetch_xor(volatile atomic_long*, long);
|
||
long atomic_fetch_xor(atomic_long*, long);
|
||
long atomic_fetch_xor_explicit(volatile atomic_long*, long, memory_order);
|
||
long atomic_fetch_xor_explicit(atomic_long*, long, memory_order);
|
||
|
||
typedef struct atomic_long
|
||
{
|
||
long __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(long __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator long() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator long() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long exchange(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long exchange(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long& __v, long __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long& __v, long __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long& __v, long __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long& __v, long __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long& __v, long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long& __v, long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long& __v, long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long& __v, long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_add(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_add(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_sub(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_sub(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_and(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_and(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_or(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_or(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_xor(long __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long fetch_xor(long __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_long() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_long() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_long(long __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_long(const atomic_long&) = delete;
|
||
atomic_long& operator=(const atomic_long&) = delete;
|
||
atomic_long& operator=(const atomic_long&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_long(const atomic_long&);
|
||
atomic_long& operator=(const atomic_long&);
|
||
atomic_long& operator=(const atomic_long&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator=(long __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator=(long __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++(int) volatile
|
||
{return fetch_add(long(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++(int)
|
||
{return fetch_add(long(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int) volatile
|
||
{return fetch_sub(long(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int)
|
||
{return fetch_sub(long(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++() volatile
|
||
{return long(fetch_add(long(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++()
|
||
{return long(fetch_add(long(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--() volatile
|
||
{return long(fetch_sub(long(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--()
|
||
{return long(fetch_sub(long(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v) volatile
|
||
{return long(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v)
|
||
{return long(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v) volatile
|
||
{return long(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v)
|
||
{return long(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v) volatile
|
||
{return long(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v)
|
||
{return long(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v) volatile
|
||
{return long(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v)
|
||
{return long(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v) volatile
|
||
{return long(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v)
|
||
{return long(fetch_xor(__v) ^ __v);}
|
||
} atomic_long;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_long*)
|
||
{
|
||
typedef long type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_long* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_long*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_long* __obj, long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_long* __obj, long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_long* __obj, long __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_long* __obj, long __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_long*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_long* __obj, long __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_long* __obj, long __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_long*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_load(const volatile atomic_long* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_load(const atomic_long* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_long*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_load_explicit(const volatile atomic_long* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_load_explicit(const atomic_long* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_long*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_exchange(volatile atomic_long* __obj, long __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_exchange(atomic_long* __obj, long __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_long*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_exchange_explicit(volatile atomic_long* __obj, long __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_exchange_explicit(atomic_long* __obj, long __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_long*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_long* __obj, long* __exp,
|
||
long __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_long* __obj, long* __exp, long __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_long*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_long* __obj, long* __exp,
|
||
long __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_long* __obj, long* __exp, long __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_long*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_long* __obj, long* __exp,
|
||
long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_long* __obj, long* __exp,
|
||
long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_long*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_long* __obj,
|
||
long* __exp, long __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_long* __obj, long* __exp,
|
||
long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_long*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_add(volatile atomic_long* __obj, long __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_add(atomic_long* __obj, long __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_long*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_add_explicit(volatile atomic_long* __obj, long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_add_explicit(atomic_long* __obj, long __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_long*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_sub(volatile atomic_long* __obj, long __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_sub(atomic_long* __obj, long __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_long*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_sub_explicit(volatile atomic_long* __obj, long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_sub_explicit(atomic_long* __obj, long __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_long*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_and(volatile atomic_long* __obj, long __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_and(atomic_long* __obj, long __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_long*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_and_explicit(volatile atomic_long* __obj, long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_and_explicit(atomic_long* __obj, long __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_long*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_or(volatile atomic_long* __obj, long __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_or(atomic_long* __obj, long __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_long*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_or_explicit(volatile atomic_long* __obj, long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_or_explicit(atomic_long* __obj, long __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_long*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_xor(volatile atomic_long* __obj, long __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_xor(atomic_long* __obj, long __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_long*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_xor_explicit(volatile atomic_long* __obj, long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long
|
||
atomic_fetch_xor_explicit(atomic_long* __obj, long __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_long*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_ulong
|
||
|
||
struct atomic_ulong;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_ulong*);
|
||
bool atomic_is_lock_free(const atomic_ulong*);
|
||
void atomic_init(volatile atomic_ulong*, unsigned long);
|
||
void atomic_init(atomic_ulong*, unsigned long);
|
||
void atomic_store(volatile atomic_ulong*, unsigned long);
|
||
void atomic_store(atomic_ulong*, unsigned long);
|
||
void atomic_store_explicit(volatile atomic_ulong*, unsigned long, memory_order);
|
||
void atomic_store_explicit(atomic_ulong*, unsigned long, memory_order);
|
||
unsigned long atomic_load(const volatile atomic_ulong*);
|
||
unsigned long atomic_load(const atomic_ulong*);
|
||
unsigned long atomic_load_explicit(const volatile atomic_ulong*, memory_order);
|
||
unsigned long atomic_load_explicit(const atomic_ulong*, memory_order);
|
||
unsigned long atomic_exchange(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_exchange(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_exchange_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_exchange_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_ulong*, unsigned long*,
|
||
unsigned long);
|
||
bool atomic_compare_exchange_weak(atomic_ulong*, unsigned long*, unsigned long);
|
||
bool atomic_compare_exchange_strong(volatile atomic_ulong*, unsigned long*,
|
||
unsigned long);
|
||
bool atomic_compare_exchange_strong(atomic_ulong*, unsigned long*,
|
||
unsigned long);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_ulong*,
|
||
unsigned long*, unsigned long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_ulong*, unsigned long*,
|
||
unsigned long, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_ulong*,
|
||
unsigned long*, unsigned long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_ulong*, unsigned long*,
|
||
unsigned long, memory_order,
|
||
memory_order);
|
||
unsigned long atomic_fetch_add(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_add(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_add_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_add_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_sub(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_sub(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_sub_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_sub_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_and(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_and(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_and_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_and_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_or(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_or(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_or_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_or_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_xor(volatile atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_xor(atomic_ulong*, unsigned long);
|
||
unsigned long atomic_fetch_xor_explicit(volatile atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
unsigned long atomic_fetch_xor_explicit(atomic_ulong*, unsigned long,
|
||
memory_order);
|
||
|
||
typedef struct atomic_ulong
|
||
{
|
||
unsigned long __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned long __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned long() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned long() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long exchange(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long exchange(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long& __v, unsigned long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long& __v, unsigned long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long& __v, unsigned long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long& __v, unsigned long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long& __v, unsigned long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long& __v, unsigned long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long& __v, unsigned long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long& __v, unsigned long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_add(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_add(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_sub(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_sub(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_and(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_and(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_or(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_or(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_xor(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long fetch_xor(unsigned long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_ulong() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_ulong() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_ulong(unsigned long __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_ulong(const atomic_ulong&) = delete;
|
||
atomic_ulong& operator=(const atomic_ulong&) = delete;
|
||
atomic_ulong& operator=(const atomic_ulong&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_ulong(const atomic_ulong&);
|
||
atomic_ulong& operator=(const atomic_ulong&);
|
||
atomic_ulong& operator=(const atomic_ulong&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long operator=(unsigned long __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long operator=(unsigned long __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long operator++(int) volatile
|
||
{typedef unsigned long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++(int)
|
||
{typedef unsigned long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int) volatile
|
||
{typedef unsigned long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int)
|
||
{typedef unsigned long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++() volatile
|
||
{typedef unsigned long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++()
|
||
{typedef unsigned long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--() volatile
|
||
{typedef unsigned long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--()
|
||
{typedef unsigned long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v) volatile
|
||
{typedef unsigned long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v)
|
||
{typedef unsigned long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v) volatile
|
||
{typedef unsigned long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v)
|
||
{typedef unsigned long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v) volatile
|
||
{typedef unsigned long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v)
|
||
{typedef unsigned long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v) volatile
|
||
{typedef unsigned long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v)
|
||
{typedef unsigned long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v) volatile
|
||
{typedef unsigned long type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v)
|
||
{typedef unsigned long type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_ulong;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_ulong*)
|
||
{
|
||
typedef unsigned long type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_ulong* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_ulong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_ulong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_ulong* __obj, unsigned long __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_ulong* __obj, unsigned long __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_ulong*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_load(const volatile atomic_ulong* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_load(const atomic_ulong* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_ulong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_load_explicit(const volatile atomic_ulong* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_load_explicit(const atomic_ulong* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_ulong*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_exchange(volatile atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_exchange(atomic_ulong* __obj, unsigned long __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_ulong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_exchange_explicit(volatile atomic_ulong* __obj, unsigned long __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_exchange_explicit(atomic_ulong* __obj, unsigned long __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_ulong*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_ulong* __obj, unsigned long* __exp,
|
||
unsigned long __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_ulong* __obj, unsigned long* __exp,
|
||
unsigned long __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_ulong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_ulong* __obj,
|
||
unsigned long* __exp, unsigned long __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_ulong* __obj, unsigned long* __exp,
|
||
unsigned long __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_ulong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_ulong* __obj,
|
||
unsigned long* __exp,
|
||
unsigned long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_ulong* __obj, unsigned long* __exp,
|
||
unsigned long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_ulong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_ulong* __obj,
|
||
unsigned long* __exp,
|
||
unsigned long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_ulong* __obj,
|
||
unsigned long* __exp,
|
||
unsigned long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_ulong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_add(volatile atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_add(atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_ulong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_add_explicit(volatile atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_add_explicit(atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_ulong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_sub(volatile atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_sub(atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_ulong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_sub_explicit(volatile atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_sub_explicit(atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_ulong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_and(volatile atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_and(atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_ulong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_and_explicit(volatile atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_and_explicit(atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_ulong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_or(volatile atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_or(atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_ulong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_or_explicit(volatile atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_or_explicit(atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_ulong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_xor(volatile atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_xor(atomic_ulong* __obj, unsigned long __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_ulong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_xor_explicit(volatile atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long
|
||
atomic_fetch_xor_explicit(atomic_ulong* __obj, unsigned long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_ulong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_llong
|
||
|
||
struct atomic_llong;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_llong*);
|
||
bool atomic_is_lock_free(const atomic_llong*);
|
||
void atomic_init(volatile atomic_llong*, long long);
|
||
void atomic_init(atomic_llong*, long long);
|
||
void atomic_store(volatile atomic_llong*, long long);
|
||
void atomic_store(atomic_llong*, long long);
|
||
void atomic_store_explicit(volatile atomic_llong*, long long, memory_order);
|
||
void atomic_store_explicit(atomic_llong*, long long, memory_order);
|
||
long long atomic_load(const volatile atomic_llong*);
|
||
long long atomic_load(const atomic_llong*);
|
||
long long atomic_load_explicit(const volatile atomic_llong*, memory_order);
|
||
long long atomic_load_explicit(const atomic_llong*, memory_order);
|
||
long long atomic_exchange(volatile atomic_llong*, long long);
|
||
long long atomic_exchange(atomic_llong*, long long);
|
||
long long atomic_exchange_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_exchange_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_llong*, long long*,
|
||
long long);
|
||
bool atomic_compare_exchange_weak(atomic_llong*, long long*, long long);
|
||
bool atomic_compare_exchange_strong(volatile atomic_llong*, long long*,
|
||
long long);
|
||
bool atomic_compare_exchange_strong(atomic_llong*, long long*,
|
||
long long);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_llong*,
|
||
long long*, long long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_llong*, long long*,
|
||
long long, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_llong*,
|
||
long long*, long long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_llong*, long long*,
|
||
long long, memory_order,
|
||
memory_order);
|
||
long long atomic_fetch_add(volatile atomic_llong*, long long);
|
||
long long atomic_fetch_add(atomic_llong*, long long);
|
||
long long atomic_fetch_add_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_add_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_sub(volatile atomic_llong*, long long);
|
||
long long atomic_fetch_sub(atomic_llong*, long long);
|
||
long long atomic_fetch_sub_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_sub_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_and(volatile atomic_llong*, long long);
|
||
long long atomic_fetch_and(atomic_llong*, long long);
|
||
long long atomic_fetch_and_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_and_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_or(volatile atomic_llong*, long long);
|
||
long long atomic_fetch_or(atomic_llong*, long long);
|
||
long long atomic_fetch_or_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_or_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_xor(volatile atomic_llong*, long long);
|
||
long long atomic_fetch_xor(atomic_llong*, long long);
|
||
long long atomic_fetch_xor_explicit(volatile atomic_llong*, long long,
|
||
memory_order);
|
||
long long atomic_fetch_xor_explicit(atomic_llong*, long long,
|
||
memory_order);
|
||
|
||
typedef struct atomic_llong
|
||
{
|
||
long long __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(long long __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator long long() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator long long() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long exchange(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long exchange(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long long& __v, long long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long long& __v, long long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long long& __v, long long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long long& __v, long long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long long& __v, long long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(long long& __v, long long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long long& __v, long long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(long long& __v, long long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_add(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_add(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_sub(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_sub(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_and(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_and(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_or(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_or(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_xor(long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long fetch_xor(long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_llong() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_llong() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_llong(long long __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_llong(const atomic_llong&) = delete;
|
||
atomic_llong& operator=(const atomic_llong&) = delete;
|
||
atomic_llong& operator=(const atomic_llong&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_llong(const atomic_llong&);
|
||
atomic_llong& operator=(const atomic_llong&);
|
||
atomic_llong& operator=(const atomic_llong&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long operator=(long long __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long operator=(long long __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long long operator++(int) volatile
|
||
{typedef long long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++(int)
|
||
{typedef long long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int) volatile
|
||
{typedef long long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int)
|
||
{typedef long long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++() volatile
|
||
{typedef long long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++()
|
||
{typedef long long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--() volatile
|
||
{typedef long long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--()
|
||
{typedef long long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v) volatile
|
||
{typedef long long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v)
|
||
{typedef long long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v) volatile
|
||
{typedef long long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v)
|
||
{typedef long long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v) volatile
|
||
{typedef long long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v)
|
||
{typedef long long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v) volatile
|
||
{typedef long long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v)
|
||
{typedef long long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v) volatile
|
||
{typedef long long type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v)
|
||
{typedef long long type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_llong;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_llong*)
|
||
{
|
||
typedef long long type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_llong* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_llong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_llong* __obj, long long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_llong* __obj, long long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_llong* __obj, long long __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_llong* __obj, long long __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_llong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_llong* __obj, long long __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_llong* __obj, long long __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_llong*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_load(const volatile atomic_llong* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_load(const atomic_llong* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_llong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_load_explicit(const volatile atomic_llong* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_load_explicit(const atomic_llong* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_llong*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_exchange(volatile atomic_llong* __obj, long long __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_exchange(atomic_llong* __obj, long long __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_llong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_exchange_explicit(volatile atomic_llong* __obj, long long __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_exchange_explicit(atomic_llong* __obj, long long __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_llong*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_llong* __obj, long long* __exp,
|
||
long long __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_llong* __obj, long long* __exp,
|
||
long long __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_llong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_llong* __obj,
|
||
long long* __exp, long long __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_llong* __obj, long long* __exp,
|
||
long long __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_llong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_llong* __obj,
|
||
long long* __exp,
|
||
long long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_llong* __obj, long long* __exp,
|
||
long long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_llong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_llong* __obj,
|
||
long long* __exp,
|
||
long long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_llong* __obj,
|
||
long long* __exp,
|
||
long long __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_llong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_add(volatile atomic_llong* __obj, long long __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_add(atomic_llong* __obj, long long __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_llong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_add_explicit(volatile atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_add_explicit(atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_llong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_sub(volatile atomic_llong* __obj, long long __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_sub(atomic_llong* __obj, long long __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_llong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_sub_explicit(volatile atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_sub_explicit(atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_llong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_and(volatile atomic_llong* __obj, long long __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_and(atomic_llong* __obj, long long __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_llong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_and_explicit(volatile atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_and_explicit(atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_llong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_or(volatile atomic_llong* __obj, long long __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_or(atomic_llong* __obj, long long __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_llong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_or_explicit(volatile atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_or_explicit(atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_llong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_xor(volatile atomic_llong* __obj, long long __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_xor(atomic_llong* __obj, long long __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_llong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_xor_explicit(volatile atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
long long
|
||
atomic_fetch_xor_explicit(atomic_llong* __obj, long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_llong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_ullong
|
||
|
||
struct atomic_ullong;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_ullong*);
|
||
bool atomic_is_lock_free(const atomic_ullong*);
|
||
void atomic_init(volatile atomic_ullong*, unsigned long long);
|
||
void atomic_init(atomic_ullong*, unsigned long long);
|
||
void atomic_store(volatile atomic_ullong*, unsigned long long);
|
||
void atomic_store(atomic_ullong*, unsigned long long);
|
||
void atomic_store_explicit(volatile atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
void atomic_store_explicit(atomic_ullong*, unsigned long long, memory_order);
|
||
unsigned long long atomic_load(const volatile atomic_ullong*);
|
||
unsigned long long atomic_load(const atomic_ullong*);
|
||
unsigned long long atomic_load_explicit(const volatile atomic_ullong*,
|
||
memory_order);
|
||
unsigned long long atomic_load_explicit(const atomic_ullong*, memory_order);
|
||
unsigned long long atomic_exchange(volatile atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_exchange(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_exchange_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_exchange_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_ullong*, unsigned long long*,
|
||
unsigned long long);
|
||
bool atomic_compare_exchange_weak(atomic_ullong*, unsigned long long*,
|
||
unsigned long long);
|
||
bool atomic_compare_exchange_strong(volatile atomic_ullong*,
|
||
unsigned long long*, unsigned long long);
|
||
bool atomic_compare_exchange_strong(atomic_ullong*, unsigned long long*,
|
||
unsigned long long);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_ullong*,
|
||
unsigned long long*,
|
||
unsigned long long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_ullong*, unsigned long long*,
|
||
unsigned long long, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_ullong*,
|
||
unsigned long long*,
|
||
unsigned long long,
|
||
memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_ullong*,
|
||
unsigned long long*,
|
||
unsigned long long, memory_order,
|
||
memory_order);
|
||
unsigned long long atomic_fetch_add(volatile atomic_ullong*,
|
||
unsigned long long);
|
||
unsigned long long atomic_fetch_add(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_add_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_fetch_add_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
unsigned long long atomic_fetch_sub(volatile atomic_ullong*,
|
||
unsigned long long);
|
||
unsigned long long atomic_fetch_sub(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_sub_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_fetch_sub_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
unsigned long long atomic_fetch_and(volatile atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_and(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_and_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_fetch_and_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
unsigned long long atomic_fetch_or(volatile atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_or(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_or_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_fetch_or_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
unsigned long long atomic_fetch_xor(volatile atomic_ullong*,
|
||
unsigned long long);
|
||
unsigned long long atomic_fetch_xor(atomic_ullong*, unsigned long long);
|
||
unsigned long long atomic_fetch_xor_explicit(volatile atomic_ullong*,
|
||
unsigned long long, memory_order);
|
||
unsigned long long atomic_fetch_xor_explicit(atomic_ullong*, unsigned long long,
|
||
memory_order);
|
||
|
||
typedef struct atomic_ullong
|
||
{
|
||
unsigned long long __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(unsigned long long __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long load(memory_order __o = memory_order_seq_cst) const
|
||
volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned long long() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator unsigned long long() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long exchange(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long exchange(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(unsigned long long& __v, unsigned long long __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_add(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_add(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_sub(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_sub(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_and(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_and(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_or(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_or(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_xor(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long fetch_xor(unsigned long long __v,
|
||
memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_ullong() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_ullong() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_ullong(unsigned long long __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_ullong(const atomic_ullong&) = delete;
|
||
atomic_ullong& operator=(const atomic_ullong&) = delete;
|
||
atomic_ullong& operator=(const atomic_ullong&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_ullong(const atomic_ullong&);
|
||
atomic_ullong& operator=(const atomic_ullong&);
|
||
atomic_ullong& operator=(const atomic_ullong&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long operator=(unsigned long long __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long operator=(unsigned long long __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long operator++(int) volatile
|
||
{typedef unsigned long long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++(int)
|
||
{typedef unsigned long long type; return fetch_add(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int) volatile
|
||
{typedef unsigned long long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--(int)
|
||
{typedef unsigned long long type; return fetch_sub(type(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++() volatile
|
||
{typedef unsigned long long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator++()
|
||
{typedef unsigned long long type; return type(fetch_add(type(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--() volatile
|
||
{typedef unsigned long long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator--()
|
||
{typedef unsigned long long type; return type(fetch_sub(type(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v) volatile
|
||
{typedef unsigned long long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator+=(long __v)
|
||
{typedef unsigned long long type; return type(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v) volatile
|
||
{typedef unsigned long long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator-=(long __v)
|
||
{typedef unsigned long long type; return type(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v) volatile
|
||
{typedef unsigned long long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator&=(long __v)
|
||
{typedef unsigned long long type; return type(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v) volatile
|
||
{typedef unsigned long long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator|=(long __v)
|
||
{typedef unsigned long long type; return type(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v) volatile
|
||
{typedef unsigned long long type; return type(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
long operator^=(long __v)
|
||
{typedef unsigned long long type; return type(fetch_xor(__v) ^ __v);}
|
||
} atomic_ullong;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_ullong*)
|
||
{
|
||
typedef unsigned long long type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_ullong* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_ullong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_ullong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_ullong* __obj, unsigned long long __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_ullong* __obj, unsigned long long __desr,
|
||
memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_ullong*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_load(const volatile atomic_ullong* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_load(const atomic_ullong* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_ullong*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_load_explicit(const volatile atomic_ullong* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_load_explicit(const atomic_ullong* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_ullong*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_exchange(volatile atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_exchange(atomic_ullong* __obj, unsigned long long __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_ullong*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_exchange_explicit(volatile atomic_ullong* __obj,
|
||
unsigned long long __desr, memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_exchange_explicit(atomic_ullong* __obj, unsigned long long __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_ullong*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_ullong* __obj, unsigned long long* __exp,
|
||
unsigned long long __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_ullong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_ullong* __obj, unsigned long long* __exp,
|
||
unsigned long long __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_ullong*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_ullong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_ullong* __obj,
|
||
unsigned long long* __exp,
|
||
unsigned long long __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_ullong*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_add(volatile atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_add(atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_ullong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_add_explicit(volatile atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_add_explicit(atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_ullong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_sub(volatile atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_sub(atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_ullong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_sub_explicit(volatile atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_sub_explicit(atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_ullong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_and(volatile atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_and(atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_ullong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_and_explicit(volatile atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_and_explicit(atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_ullong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_or(volatile atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_or(atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_ullong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_or_explicit(volatile atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_or_explicit(atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_ullong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_xor(volatile atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_xor(atomic_ullong* __obj, unsigned long long __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_ullong*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_xor_explicit(volatile atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
unsigned long long
|
||
atomic_fetch_xor_explicit(atomic_ullong* __obj, unsigned long long __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_ullong*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
#ifndef _LIBCPP_HAS_NO_UNICODE_CHARS
|
||
|
||
// atomic_char16_t
|
||
|
||
struct atomic_char16_t;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_char16_t*);
|
||
bool atomic_is_lock_free(const atomic_char16_t*);
|
||
void atomic_init(volatile atomic_char16_t*, char16_t);
|
||
void atomic_init(atomic_char16_t*, char16_t);
|
||
void atomic_store(volatile atomic_char16_t*, char16_t);
|
||
void atomic_store(atomic_char16_t*, char16_t);
|
||
void atomic_store_explicit(volatile atomic_char16_t*, char16_t, memory_order);
|
||
void atomic_store_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
char16_t atomic_load(const volatile atomic_char16_t*);
|
||
char16_t atomic_load(const atomic_char16_t*);
|
||
char16_t atomic_load_explicit(const volatile atomic_char16_t*, memory_order);
|
||
char16_t atomic_load_explicit(const atomic_char16_t*, memory_order);
|
||
char16_t atomic_exchange(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_exchange(atomic_char16_t*, char16_t);
|
||
char16_t atomic_exchange_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_exchange_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_char16_t*, char16_t*,
|
||
char16_t);
|
||
bool atomic_compare_exchange_weak(atomic_char16_t*, char16_t*, char16_t);
|
||
bool atomic_compare_exchange_strong(volatile atomic_char16_t*, char16_t*,
|
||
char16_t);
|
||
bool atomic_compare_exchange_strong(atomic_char16_t*, char16_t*, char16_t);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_char16_t*, char16_t*,
|
||
char16_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_char16_t*, char16_t*,
|
||
char16_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_char16_t*,
|
||
char16_t*, char16_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_char16_t*, char16_t*,
|
||
char16_t, memory_order,
|
||
memory_order);
|
||
char16_t atomic_fetch_add(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_add(atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_add_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_fetch_add_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
char16_t atomic_fetch_sub(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_sub(atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_sub_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_fetch_sub_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
char16_t atomic_fetch_and(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_and(atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_and_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_fetch_and_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
char16_t atomic_fetch_or(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_or(atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_or_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_fetch_or_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
char16_t atomic_fetch_xor(volatile atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_xor(atomic_char16_t*, char16_t);
|
||
char16_t atomic_fetch_xor_explicit(volatile atomic_char16_t*, char16_t,
|
||
memory_order);
|
||
char16_t atomic_fetch_xor_explicit(atomic_char16_t*, char16_t, memory_order);
|
||
|
||
typedef struct atomic_char16_t
|
||
{
|
||
char16_t __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char16_t __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char16_t() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char16_t() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t exchange(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t exchange(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char16_t& __v, char16_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char16_t& __v, char16_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char16_t& __v, char16_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char16_t& __v, char16_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char16_t& __v, char16_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char16_t& __v, char16_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char16_t& __v, char16_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char16_t& __v, char16_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_add(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_add(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_sub(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_sub(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_and(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_and(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_or(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_or(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_xor(char16_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t fetch_xor(char16_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_char16_t() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_char16_t() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_char16_t(char16_t __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_char16_t(const atomic_char16_t&) = delete;
|
||
atomic_char16_t& operator=(const atomic_char16_t&) = delete;
|
||
atomic_char16_t& operator=(const atomic_char16_t&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_char16_t(const atomic_char16_t&);
|
||
atomic_char16_t& operator=(const atomic_char16_t&);
|
||
atomic_char16_t& operator=(const atomic_char16_t&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator=(char16_t __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator=(char16_t __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator++(int) volatile
|
||
{return fetch_add(char16_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator++(int)
|
||
{return fetch_add(char16_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator--(int) volatile
|
||
{return fetch_sub(char16_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator--(int)
|
||
{return fetch_sub(char16_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator++() volatile
|
||
{return char16_t(fetch_add(char16_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator++()
|
||
{return char16_t(fetch_add(char16_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator--() volatile
|
||
{return char16_t(fetch_sub(char16_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator--()
|
||
{return char16_t(fetch_sub(char16_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator+=(char16_t __v) volatile
|
||
{return char16_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator+=(char16_t __v)
|
||
{return char16_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator-=(char16_t __v) volatile
|
||
{return char16_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator-=(char16_t __v)
|
||
{return char16_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator&=(char16_t __v) volatile
|
||
{return char16_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator&=(char16_t __v)
|
||
{return char16_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator|=(char16_t __v) volatile
|
||
{return char16_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator|=(char16_t __v)
|
||
{return char16_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator^=(char16_t __v) volatile
|
||
{return char16_t(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char16_t operator^=(char16_t __v)
|
||
{return char16_t(fetch_xor(__v) ^ __v);}
|
||
} atomic_char16_t;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_char16_t*)
|
||
{
|
||
typedef char16_t type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_char16_t* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_char16_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_char16_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_char16_t* __obj, char16_t __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_char16_t* __obj, char16_t __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_char16_t*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_load(const volatile atomic_char16_t* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_load(const atomic_char16_t* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_char16_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_load_explicit(const volatile atomic_char16_t* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_load_explicit(const atomic_char16_t* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_char16_t*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_exchange(volatile atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_exchange(atomic_char16_t* __obj, char16_t __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_char16_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_exchange_explicit(volatile atomic_char16_t* __obj, char16_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_exchange_explicit(atomic_char16_t* __obj, char16_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_char16_t*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_char16_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_char16_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_char16_t* __obj,
|
||
char16_t* __exp, char16_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_char16_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_char16_t* __obj,
|
||
char16_t* __exp, char16_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_char16_t* __obj, char16_t* __exp,
|
||
char16_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_char16_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_add(volatile atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_add(atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_char16_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_add_explicit(volatile atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_add_explicit(atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_char16_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_sub(volatile atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_sub(atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_char16_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_sub_explicit(volatile atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_sub_explicit(atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_char16_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_and(volatile atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_and(atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_char16_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_and_explicit(volatile atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_and_explicit(atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_char16_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_or(volatile atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_or(atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_char16_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_or_explicit(volatile atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_or_explicit(atomic_char16_t* __obj, char16_t __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_char16_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_xor(volatile atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_xor(atomic_char16_t* __obj, char16_t __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_char16_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_xor_explicit(volatile atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char16_t
|
||
atomic_fetch_xor_explicit(atomic_char16_t* __obj, char16_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_char16_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_char32_t
|
||
|
||
struct atomic_char32_t;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_char32_t*);
|
||
bool atomic_is_lock_free(const atomic_char32_t*);
|
||
void atomic_init(volatile atomic_char32_t*, char32_t);
|
||
void atomic_init(atomic_char32_t*, char32_t);
|
||
void atomic_store(volatile atomic_char32_t*, char32_t);
|
||
void atomic_store(atomic_char32_t*, char32_t);
|
||
void atomic_store_explicit(volatile atomic_char32_t*, char32_t, memory_order);
|
||
void atomic_store_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
char32_t atomic_load(const volatile atomic_char32_t*);
|
||
char32_t atomic_load(const atomic_char32_t*);
|
||
char32_t atomic_load_explicit(const volatile atomic_char32_t*, memory_order);
|
||
char32_t atomic_load_explicit(const atomic_char32_t*, memory_order);
|
||
char32_t atomic_exchange(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_exchange(atomic_char32_t*, char32_t);
|
||
char32_t atomic_exchange_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_exchange_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_char32_t*, char32_t*,
|
||
char32_t);
|
||
bool atomic_compare_exchange_weak(atomic_char32_t*, char32_t*, char32_t);
|
||
bool atomic_compare_exchange_strong(volatile atomic_char32_t*, char32_t*,
|
||
char32_t);
|
||
bool atomic_compare_exchange_strong(atomic_char32_t*, char32_t*, char32_t);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_char32_t*, char32_t*,
|
||
char32_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_char32_t*, char32_t*,
|
||
char32_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_char32_t*,
|
||
char32_t*, char32_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_char32_t*, char32_t*,
|
||
char32_t, memory_order,
|
||
memory_order);
|
||
char32_t atomic_fetch_add(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_add(atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_add_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_fetch_add_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
char32_t atomic_fetch_sub(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_sub(atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_sub_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_fetch_sub_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
char32_t atomic_fetch_and(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_and(atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_and_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_fetch_and_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
char32_t atomic_fetch_or(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_or(atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_or_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_fetch_or_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
char32_t atomic_fetch_xor(volatile atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_xor(atomic_char32_t*, char32_t);
|
||
char32_t atomic_fetch_xor_explicit(volatile atomic_char32_t*, char32_t,
|
||
memory_order);
|
||
char32_t atomic_fetch_xor_explicit(atomic_char32_t*, char32_t, memory_order);
|
||
|
||
typedef struct atomic_char32_t
|
||
{
|
||
char32_t __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char32_t __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char32_t() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator char32_t() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t exchange(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t exchange(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char32_t& __v, char32_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char32_t& __v, char32_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char32_t& __v, char32_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char32_t& __v, char32_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char32_t& __v, char32_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(char32_t& __v, char32_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char32_t& __v, char32_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(char32_t& __v, char32_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_add(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_add(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_sub(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_sub(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_and(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_and(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_or(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_or(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_xor(char32_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t fetch_xor(char32_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_char32_t() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_char32_t() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_char32_t(char32_t __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_char32_t(const atomic_char32_t&) = delete;
|
||
atomic_char32_t& operator=(const atomic_char32_t&) = delete;
|
||
atomic_char32_t& operator=(const atomic_char32_t&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_char32_t(const atomic_char32_t&);
|
||
atomic_char32_t& operator=(const atomic_char32_t&);
|
||
atomic_char32_t& operator=(const atomic_char32_t&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator=(char32_t __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator=(char32_t __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator++(int) volatile
|
||
{return fetch_add(char32_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator++(int)
|
||
{return fetch_add(char32_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator--(int) volatile
|
||
{return fetch_sub(char32_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator--(int)
|
||
{return fetch_sub(char32_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator++() volatile
|
||
{return char32_t(fetch_add(char32_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator++()
|
||
{return char32_t(fetch_add(char32_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator--() volatile
|
||
{return char32_t(fetch_sub(char32_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator--()
|
||
{return char32_t(fetch_sub(char32_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator+=(char32_t __v) volatile
|
||
{return char32_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator+=(char32_t __v)
|
||
{return char32_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator-=(char32_t __v) volatile
|
||
{return char32_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator-=(char32_t __v)
|
||
{return char32_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator&=(char32_t __v) volatile
|
||
{return char32_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator&=(char32_t __v)
|
||
{return char32_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator|=(char32_t __v) volatile
|
||
{return char32_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator|=(char32_t __v)
|
||
{return char32_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator^=(char32_t __v) volatile
|
||
{return char32_t(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
char32_t operator^=(char32_t __v)
|
||
{return char32_t(fetch_xor(__v) ^ __v);}
|
||
} atomic_char32_t;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_char32_t*)
|
||
{
|
||
typedef char32_t type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_char32_t* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_char32_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_char32_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_char32_t* __obj, char32_t __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_char32_t* __obj, char32_t __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_char32_t*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_load(const volatile atomic_char32_t* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_load(const atomic_char32_t* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_char32_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_load_explicit(const volatile atomic_char32_t* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_load_explicit(const atomic_char32_t* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_char32_t*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_exchange(volatile atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_exchange(atomic_char32_t* __obj, char32_t __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_char32_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_exchange_explicit(volatile atomic_char32_t* __obj, char32_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_exchange_explicit(atomic_char32_t* __obj, char32_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_char32_t*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_char32_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_char32_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_char32_t* __obj,
|
||
char32_t* __exp, char32_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_char32_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_char32_t* __obj,
|
||
char32_t* __exp, char32_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_char32_t* __obj, char32_t* __exp,
|
||
char32_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_char32_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_add(volatile atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_add(atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_char32_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_add_explicit(volatile atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_add_explicit(atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_char32_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_sub(volatile atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_sub(atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_char32_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_sub_explicit(volatile atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_sub_explicit(atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_char32_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_and(volatile atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_and(atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_char32_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_and_explicit(volatile atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_and_explicit(atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_char32_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_or(volatile atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_or(atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_char32_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_or_explicit(volatile atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_or_explicit(atomic_char32_t* __obj, char32_t __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_char32_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_xor(volatile atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_xor(atomic_char32_t* __obj, char32_t __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_char32_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_xor_explicit(volatile atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
char32_t
|
||
atomic_fetch_xor_explicit(atomic_char32_t* __obj, char32_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_char32_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
#endif // _LIBCPP_HAS_NO_UNICODE_CHARS
|
||
|
||
// atomic_wchar_t
|
||
|
||
struct atomic_wchar_t;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_wchar_t*);
|
||
bool atomic_is_lock_free(const atomic_wchar_t*);
|
||
void atomic_init(volatile atomic_wchar_t*, wchar_t);
|
||
void atomic_init(atomic_wchar_t*, wchar_t);
|
||
void atomic_store(volatile atomic_wchar_t*, wchar_t);
|
||
void atomic_store(atomic_wchar_t*, wchar_t);
|
||
void atomic_store_explicit(volatile atomic_wchar_t*, wchar_t, memory_order);
|
||
void atomic_store_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
wchar_t atomic_load(const volatile atomic_wchar_t*);
|
||
wchar_t atomic_load(const atomic_wchar_t*);
|
||
wchar_t atomic_load_explicit(const volatile atomic_wchar_t*, memory_order);
|
||
wchar_t atomic_load_explicit(const atomic_wchar_t*, memory_order);
|
||
wchar_t atomic_exchange(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_exchange(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_exchange_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_exchange_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_wchar_t*, wchar_t*,
|
||
wchar_t);
|
||
bool atomic_compare_exchange_weak(atomic_wchar_t*, wchar_t*, wchar_t);
|
||
bool atomic_compare_exchange_strong(volatile atomic_wchar_t*, wchar_t*,
|
||
wchar_t);
|
||
bool atomic_compare_exchange_strong(atomic_wchar_t*, wchar_t*, wchar_t);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_wchar_t*, wchar_t*,
|
||
wchar_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_wchar_t*, wchar_t*,
|
||
wchar_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_wchar_t*,
|
||
wchar_t*, wchar_t, memory_order,
|
||
memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_wchar_t*, wchar_t*,
|
||
wchar_t, memory_order,
|
||
memory_order);
|
||
wchar_t atomic_fetch_add(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_add(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_add_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_fetch_add_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
wchar_t atomic_fetch_sub(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_sub(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_sub_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_fetch_sub_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
wchar_t atomic_fetch_and(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_and(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_and_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_fetch_and_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
wchar_t atomic_fetch_or(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_or(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_or_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_fetch_or_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
wchar_t atomic_fetch_xor(volatile atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_xor(atomic_wchar_t*, wchar_t);
|
||
wchar_t atomic_fetch_xor_explicit(volatile atomic_wchar_t*, wchar_t,
|
||
memory_order);
|
||
wchar_t atomic_fetch_xor_explicit(atomic_wchar_t*, wchar_t, memory_order);
|
||
|
||
typedef struct atomic_wchar_t
|
||
{
|
||
wchar_t __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(wchar_t __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator wchar_t() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator wchar_t() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t exchange(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t exchange(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(wchar_t& __v, wchar_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(wchar_t& __v, wchar_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(wchar_t& __v, wchar_t __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(wchar_t& __v, wchar_t __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(wchar_t& __v, wchar_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(wchar_t& __v, wchar_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(wchar_t& __v, wchar_t __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(wchar_t& __v, wchar_t __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_add(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_add(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_sub(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_sub(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_and(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_and(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_and_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_or(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_or(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_or_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_xor(wchar_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t fetch_xor(wchar_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_xor_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_wchar_t() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_wchar_t() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_wchar_t(wchar_t __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_wchar_t(const atomic_wchar_t&) = delete;
|
||
atomic_wchar_t& operator=(const atomic_wchar_t&) = delete;
|
||
atomic_wchar_t& operator=(const atomic_wchar_t&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_wchar_t(const atomic_wchar_t&);
|
||
atomic_wchar_t& operator=(const atomic_wchar_t&);
|
||
atomic_wchar_t& operator=(const atomic_wchar_t&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator=(wchar_t __v) volatile
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator=(wchar_t __v)
|
||
{store(__v); return __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator++(int) volatile
|
||
{return fetch_add(wchar_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator++(int)
|
||
{return fetch_add(wchar_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator--(int) volatile
|
||
{return fetch_sub(wchar_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator--(int)
|
||
{return fetch_sub(wchar_t(1));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator++() volatile
|
||
{return wchar_t(fetch_add(wchar_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator++()
|
||
{return wchar_t(fetch_add(wchar_t(1)) + 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator--() volatile
|
||
{return wchar_t(fetch_sub(wchar_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator--()
|
||
{return wchar_t(fetch_sub(wchar_t(1)) - 1);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator+=(wchar_t __v) volatile
|
||
{return wchar_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator+=(wchar_t __v)
|
||
{return wchar_t(fetch_add(__v) + __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator-=(wchar_t __v) volatile
|
||
{return wchar_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator-=(wchar_t __v)
|
||
{return wchar_t(fetch_sub(__v) - __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator&=(wchar_t __v) volatile
|
||
{return wchar_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator&=(wchar_t __v)
|
||
{return wchar_t(fetch_and(__v) & __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator|=(wchar_t __v) volatile
|
||
{return wchar_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator|=(wchar_t __v)
|
||
{return wchar_t(fetch_or(__v) | __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator^=(wchar_t __v) volatile
|
||
{return wchar_t(fetch_xor(__v) ^ __v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
wchar_t operator^=(wchar_t __v)
|
||
{return wchar_t(fetch_xor(__v) ^ __v);}
|
||
} atomic_wchar_t;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_wchar_t*)
|
||
{
|
||
typedef wchar_t type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_wchar_t* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_wchar_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_wchar_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_wchar_t* __obj, wchar_t __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_wchar_t* __obj, wchar_t __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_wchar_t*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_load(const volatile atomic_wchar_t* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_load(const atomic_wchar_t* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_wchar_t*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_load_explicit(const volatile atomic_wchar_t* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_load_explicit(const atomic_wchar_t* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_wchar_t*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_exchange(volatile atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_exchange(atomic_wchar_t* __obj, wchar_t __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_wchar_t*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_exchange_explicit(volatile atomic_wchar_t* __obj, wchar_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_exchange_explicit(atomic_wchar_t* __obj, wchar_t __desr,
|
||
memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_wchar_t*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_wchar_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_wchar_t*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_wchar_t* __obj,
|
||
wchar_t* __exp, wchar_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_wchar_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_wchar_t* __obj,
|
||
wchar_t* __exp, wchar_t __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_wchar_t* __obj, wchar_t* __exp,
|
||
wchar_t __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_wchar_t*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_add(volatile atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_add(atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_wchar_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_add_explicit(volatile atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_add_explicit(atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_wchar_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_sub(volatile atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_sub(atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_wchar_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_sub_explicit(volatile atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_sub_explicit(atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_wchar_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_and(volatile atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_and(atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return atomic_fetch_and(const_cast<volatile atomic_wchar_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_and_explicit(volatile atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_and(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_and_explicit(atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_and_explicit(const_cast<volatile atomic_wchar_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_or(volatile atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_or(atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return atomic_fetch_or(const_cast<volatile atomic_wchar_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_or_explicit(volatile atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_or(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_or_explicit(atomic_wchar_t* __obj, wchar_t __v, memory_order __o)
|
||
{
|
||
return atomic_fetch_or_explicit(const_cast<volatile atomic_wchar_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_xor(volatile atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_xor(atomic_wchar_t* __obj, wchar_t __v)
|
||
{
|
||
return atomic_fetch_xor(const_cast<volatile atomic_wchar_t*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_xor_explicit(volatile atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_xor(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
wchar_t
|
||
atomic_fetch_xor_explicit(atomic_wchar_t* __obj, wchar_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_xor_explicit(const_cast<volatile atomic_wchar_t*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
// atomic_address
|
||
|
||
struct atomic_address;
|
||
|
||
bool atomic_is_lock_free(const volatile atomic_address*);
|
||
bool atomic_is_lock_free(const atomic_address*);
|
||
void atomic_init(volatile atomic_address*, void*);
|
||
void atomic_init(atomic_address*, void*);
|
||
void atomic_store(volatile atomic_address*, void*);
|
||
void atomic_store(atomic_address*, void*);
|
||
void atomic_store_explicit(volatile atomic_address*, void*, memory_order);
|
||
void atomic_store_explicit(atomic_address*, void*, memory_order);
|
||
void* atomic_load(const volatile atomic_address*);
|
||
void* atomic_load(const atomic_address*);
|
||
void* atomic_load_explicit(const volatile atomic_address*, memory_order);
|
||
void* atomic_load_explicit(const atomic_address*, memory_order);
|
||
void* atomic_exchange(volatile atomic_address*, void*);
|
||
void* atomic_exchange(atomic_address*, void*);
|
||
void* atomic_exchange_explicit(volatile atomic_address*, void*, memory_order);
|
||
void* atomic_exchange_explicit(atomic_address*, void*, memory_order);
|
||
bool atomic_compare_exchange_weak(volatile atomic_address*, void**, void*);
|
||
bool atomic_compare_exchange_weak(atomic_address*, void**, void*);
|
||
bool atomic_compare_exchange_strong(volatile atomic_address*, void**, void*);
|
||
bool atomic_compare_exchange_strong(atomic_address*, void**, void*);
|
||
bool atomic_compare_exchange_weak_explicit(volatile atomic_address*, void**,
|
||
void*, memory_order, memory_order);
|
||
bool atomic_compare_exchange_weak_explicit(atomic_address*, void**,
|
||
void*, memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(volatile atomic_address*, void**,
|
||
void*, memory_order, memory_order);
|
||
bool atomic_compare_exchange_strong_explicit(atomic_address*, void**,
|
||
void*, memory_order, memory_order);
|
||
void* atomic_fetch_add(volatile atomic_address*, ptrdiff_t);
|
||
void* atomic_fetch_add(atomic_address*, ptrdiff_t);
|
||
void* atomic_fetch_add_explicit(volatile atomic_address*, ptrdiff_t,
|
||
memory_order);
|
||
void* atomic_fetch_add_explicit(atomic_address*, ptrdiff_t, memory_order);
|
||
void* atomic_fetch_sub(volatile atomic_address*, ptrdiff_t);
|
||
void* atomic_fetch_sub(atomic_address*, ptrdiff_t);
|
||
void* atomic_fetch_sub_explicit(volatile atomic_address*, ptrdiff_t,
|
||
memory_order);
|
||
void* atomic_fetch_sub_explicit(atomic_address*, ptrdiff_t, memory_order);
|
||
|
||
typedef struct atomic_address
|
||
{
|
||
void* __v_;
|
||
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const volatile
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool is_lock_free() const
|
||
{return atomic_is_lock_free(this);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(void* __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void store(void* __v, memory_order __o = memory_order_seq_cst)
|
||
{atomic_store_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* load(memory_order __o = memory_order_seq_cst) const volatile
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* load(memory_order __o = memory_order_seq_cst) const
|
||
{return atomic_load_explicit(this, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator void*() const volatile
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
operator void*() const
|
||
{return load();}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* exchange(void* __v, memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* exchange(void* __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_exchange_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(void*& __v, void* __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(void*& __v, void* __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(void*& __v, void* __e, memory_order __s,
|
||
memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(void*& __v, void* __e, memory_order __s,
|
||
memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(void*& __v, void* __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(void*& __v, void* __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(void*& __v, void* __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(void*& __v, void* __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this, &__v, __e, __s,
|
||
__translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(const void*& __v, const void* __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e), __s, __f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(const void*& __v, const void* __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_weak_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e), __s, __f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(const void*& __v, const void* __e,
|
||
memory_order __s, memory_order __f) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e), __s, __f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(const void*& __v, const void* __e,
|
||
memory_order __s, memory_order __f)
|
||
{return atomic_compare_exchange_strong_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e), __s, __f);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(const void*& __v, const void* __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_weak_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e),
|
||
__s, __translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_weak(const void*& __v, const void* __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_weak_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e),
|
||
__s, __translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(const void*& __v, const void* __e,
|
||
memory_order __s = memory_order_seq_cst) volatile
|
||
{return atomic_compare_exchange_strong_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e),
|
||
__s, __translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
bool compare_exchange_strong(const void*& __v, const void* __e,
|
||
memory_order __s = memory_order_seq_cst)
|
||
{return atomic_compare_exchange_strong_explicit(this,
|
||
&const_cast<void*&>(__v), const_cast<void*>(__e),
|
||
__s, __translate_memory_order(__s));}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* fetch_add(ptrdiff_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* fetch_add(ptrdiff_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_add_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* fetch_sub(ptrdiff_t __v,
|
||
memory_order __o = memory_order_seq_cst) volatile
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* fetch_sub(ptrdiff_t __v, memory_order __o = memory_order_seq_cst)
|
||
{return atomic_fetch_sub_explicit(this, __v, __o);}
|
||
#ifndef _LIBCPP_HAS_NO_DEFAULTED_FUNCTIONS
|
||
atomic_address() = default;
|
||
#else
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
atomic_address() {}
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
constexpr atomic_address(void* __v)
|
||
: __v_(__v) {}
|
||
#ifndef _LIBCPP_HAS_NO_DELETED_FUNCTIONS
|
||
atomic_address(const atomic_address&) = delete;
|
||
atomic_address& operator=(const atomic_address&) = delete;
|
||
atomic_address& operator=(const atomic_address&) volatile = delete;
|
||
#else
|
||
private:
|
||
atomic_address(const atomic_address&);
|
||
atomic_address& operator=(const atomic_address&);
|
||
atomic_address& operator=(const atomic_address&) volatile;
|
||
public:
|
||
#endif
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator=(const void* __v) volatile
|
||
{store(const_cast<void*>(__v)); return const_cast<void*>(__v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator=(const void* __v)
|
||
{store(const_cast<void*>(__v)); return const_cast<void*>(__v);}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator+=(ptrdiff_t __v) volatile
|
||
{return static_cast<char*>(fetch_add(__v)) + __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator+=(ptrdiff_t __v)
|
||
{return static_cast<char*>(fetch_add(__v)) + __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator-=(ptrdiff_t __v) volatile
|
||
{return static_cast<char*>(fetch_sub(__v)) - __v;}
|
||
_LIBCPP_INLINE_VISIBILITY
|
||
void* operator-=(ptrdiff_t __v)
|
||
{return static_cast<char*>(fetch_sub(__v)) - __v;}
|
||
} atomic_address;
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const volatile atomic_address*)
|
||
{
|
||
typedef void* type;
|
||
return __atomic_is_lock_free(type);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_is_lock_free(const atomic_address* __obj)
|
||
{
|
||
return atomic_is_lock_free(const_cast<volatile atomic_address*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(volatile atomic_address* __obj, void* __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_init(atomic_address* __obj, void* __desr)
|
||
{
|
||
__obj->__v_ = __desr;
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(volatile atomic_address* __obj, void* __desr)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store(atomic_address* __obj, void* __desr)
|
||
{
|
||
atomic_store(const_cast<volatile atomic_address*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(volatile atomic_address* __obj, void* __desr,
|
||
memory_order __o)
|
||
{
|
||
__atomic_store(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void
|
||
atomic_store_explicit(atomic_address* __obj, void* __desr, memory_order __o)
|
||
{
|
||
atomic_store_explicit(const_cast<volatile atomic_address*>(__obj), __desr,
|
||
__o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_load(const volatile atomic_address* __obj)
|
||
{
|
||
return __atomic_load(&__obj->__v_, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_load(const atomic_address* __obj)
|
||
{
|
||
return atomic_load(const_cast<const volatile atomic_address*>(__obj));
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_load_explicit(const volatile atomic_address* __obj, memory_order __o)
|
||
{
|
||
return __atomic_load(&__obj->__v_, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_load_explicit(const atomic_address* __obj, memory_order __o)
|
||
{
|
||
return atomic_load_explicit(const_cast<const volatile atomic_address*>
|
||
(__obj), __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_exchange(volatile atomic_address* __obj, void* __desr)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_exchange(atomic_address* __obj, void* __desr)
|
||
{
|
||
return atomic_exchange(const_cast<volatile atomic_address*>(__obj), __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_exchange_explicit(volatile atomic_address* __obj, void* __desr,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_exchange(&__obj->__v_, __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_exchange_explicit(atomic_address* __obj, void* __desr, memory_order __o)
|
||
{
|
||
return atomic_exchange_explicit(const_cast<volatile atomic_address*>
|
||
(__obj), __desr, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(volatile atomic_address* __obj, void** __exp,
|
||
void* __desr)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak(atomic_address* __obj, void** __exp, void* __desr)
|
||
{
|
||
return atomic_compare_exchange_weak(const_cast<volatile atomic_address*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(volatile atomic_address* __obj, void** __exp,
|
||
void* __desr)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr,
|
||
memory_order_seq_cst, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong(atomic_address* __obj, void** __exp,
|
||
void* __desr)
|
||
{
|
||
return atomic_compare_exchange_strong(const_cast<volatile atomic_address*>
|
||
(__obj), __exp, __desr);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(volatile atomic_address* __obj,
|
||
void** __exp, void* __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_weak(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_weak_explicit(atomic_address* __obj, void** __exp,
|
||
void* __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_weak_explicit(
|
||
const_cast<volatile atomic_address*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(volatile atomic_address* __obj,
|
||
void** __exp, void* __desr,
|
||
memory_order __s, memory_order __f)
|
||
{
|
||
return __atomic_compare_exchange_strong(&__obj->__v_, __exp, __desr, __s,
|
||
__f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
bool
|
||
atomic_compare_exchange_strong_explicit(atomic_address* __obj, void** __exp,
|
||
void* __desr, memory_order __s,
|
||
memory_order __f)
|
||
{
|
||
return atomic_compare_exchange_strong_explicit(
|
||
const_cast<volatile atomic_address*>(__obj), __exp, __desr, __s, __f);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_add(volatile atomic_address* __obj, ptrdiff_t __v)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_add(atomic_address* __obj, ptrdiff_t __v)
|
||
{
|
||
return atomic_fetch_add(const_cast<volatile atomic_address*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_add_explicit(volatile atomic_address* __obj, ptrdiff_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_add(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_add_explicit(atomic_address* __obj, ptrdiff_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_add_explicit(const_cast<volatile atomic_address*>(__obj),
|
||
__v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_sub(volatile atomic_address* __obj, ptrdiff_t __v)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, memory_order_seq_cst);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_sub(atomic_address* __obj, ptrdiff_t __v)
|
||
{
|
||
return atomic_fetch_sub(const_cast<volatile atomic_address*>(__obj), __v);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_sub_explicit(volatile atomic_address* __obj, ptrdiff_t __v,
|
||
memory_order __o)
|
||
{
|
||
return __atomic_fetch_sub(&__obj->__v_, __v, __o);
|
||
}
|
||
|
||
inline _LIBCPP_INLINE_VISIBILITY
|
||
void*
|
||
atomic_fetch_sub_explicit(atomic_address* __obj, ptrdiff_t __v,
|
||
memory_order __o)
|
||
{
|
||
return atomic_fetch_sub_explicit(const_cast<volatile atomic_address*>(__obj),
|
||
__v, __o);
|
||
}
|
||
*/
|
||
_LIBCPP_END_NAMESPACE_STD
|
||
|
||
#endif // _LIBCPP_ATOMIC
|